Use MySQL in Go language to achieve fast data retrieval
With the development of the Internet and the increase in data volume, fast retrieval has become a necessity for data storage and management. As one of the most popular relational databases currently, MySQL has powerful query capabilities and stable performance. This article will introduce how to use Go language to achieve fast retrieval of data.
1. MySQL database basics
- Creation and use of database
To create a new database in MySQL, you can use the following command:
CREATE DATABASE database_name;
Use the following command to enter the specified database:
USE database_name;
- Creation and use of tables
To create a table, use the following command:
CREATE TABLE table_name ( column_name1 data_type1, column_name2 data_type2, ... column_nameN data_typeN );
For example, we can create a user table:
CREATE TABLE user ( id INT PRIMARY KEY, name VARCHAR(20), age INT );
To insert data, use the following command:
INSERT INTO user (id, name, age) VALUES (1, 'Tom', 28);
To query data, you can use the following command:
SELECT * FROM user WHERE age >= 25;
2. Use Go to connect to MySQL
- Install the database driver
First you need to install the MySQL database driver. You can use the following command:
go get -u github.com/go-sql-driver/mysql
- Establish a connection
Use the following code to establish a connection with the MySQL database:
import ( "database/sql" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "user:password@tcp(ip:port)/database_name") if err != nil { panic(err.Error()) } defer db.Close() }
You need to enter user, password, Replace ip, port and database_name with the correct values.
- Query data
Use the following code to query data:
rows, err := db.Query("SELECT * FROM user WHERE age >= ?", 25) if err != nil { panic(err.Error()) } defer rows.Close() for rows.Next() { var id int var name string var age int err := rows.Scan(&id, &name, &age) if err != nil { panic(err.Error()) } fmt.Println(id, name, age) }
You need to replace the SELECT statement and query parameters with the correct values.
- Insert data
Use the following code to insert data:
stmt, err := db.Prepare("INSERT INTO user (id, name, age) VALUES (?, ?, ?)") if err != nil { panic(err.Error()) } defer stmt.Close() _, err = stmt.Exec(2, "Jerry", 30) if err != nil { panic(err.Error()) }
You need to replace the INSERT statement and insertion parameters with the correct values.
3. Use indexes to speed up queries
The index in MySQL is a data structure that can speed up query operations on a certain column in the table. When using MySQL database for data query in Go language, using indexes can significantly improve query efficiency. The following is some basic knowledge about indexes:
- Index types
Common index types in MySQL are: B-Tree index, hash index, full-text index, etc. The most commonly used one is the B-Tree index.
- Index creation
Use the following statement to create an index for a column in the table:
CREATE INDEX index_name ON table_name (column_name);
For example, we can create an index for the age column in the user table Create an index:
CREATE INDEX age_index ON user (age);
- Index usage
When querying, you can use the following statement to force MySQL to use the index:
SELECT * FROM user USE INDEX (age_index) WHERE age >= 25;
In actual situations, MySQL will automatically choose the most appropriate index to use. You can use the following command to view the execution plan of the query:
EXPLAIN SELECT * FROM user WHERE age >= 25;
4. Summary
This article introduces how to use Go language to connect to the MySQL database and use indexes to accelerate queries. In practical applications, using appropriate indexes and optimizing SQL statements can greatly improve the query efficiency and performance of the database and achieve rapid data retrieval.
The above is the detailed content of Use MySQL in Go language to achieve fast data retrieval. For more information, please follow other related articles on the PHP Chinese website!
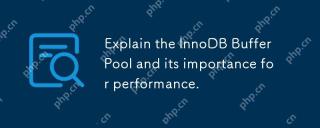
InnoDBBufferPool reduces disk I/O by caching data and indexing pages, improving database performance. Its working principle includes: 1. Data reading: Read data from BufferPool; 2. Data writing: After modifying the data, write to BufferPool and refresh it to disk regularly; 3. Cache management: Use the LRU algorithm to manage cache pages; 4. Reading mechanism: Load adjacent data pages in advance. By sizing the BufferPool and using multiple instances, database performance can be optimized.
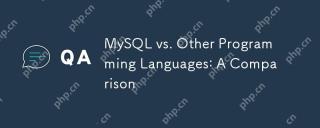
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
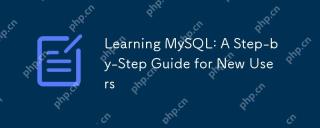
MySQL is worth learning because it is a powerful open source database management system suitable for data storage, management and analysis. 1) MySQL is a relational database that uses SQL to operate data and is suitable for structured data management. 2) The SQL language is the key to interacting with MySQL and supports CRUD operations. 3) The working principle of MySQL includes client/server architecture, storage engine and query optimizer. 4) Basic usage includes creating databases and tables, and advanced usage involves joining tables using JOIN. 5) Common errors include syntax errors and permission issues, and debugging skills include checking syntax and using EXPLAIN commands. 6) Performance optimization involves the use of indexes, optimization of SQL statements and regular maintenance of databases.
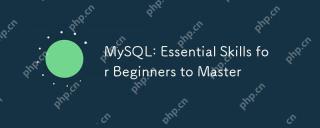
MySQL is suitable for beginners to learn database skills. 1. Install MySQL server and client tools. 2. Understand basic SQL queries, such as SELECT. 3. Master data operations: create tables, insert, update, and delete data. 4. Learn advanced skills: subquery and window functions. 5. Debugging and optimization: Check syntax, use indexes, avoid SELECT*, and use LIMIT.
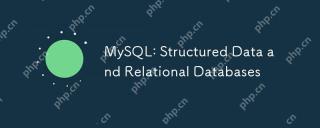
MySQL efficiently manages structured data through table structure and SQL query, and implements inter-table relationships through foreign keys. 1. Define the data format and type when creating a table. 2. Use foreign keys to establish relationships between tables. 3. Improve performance through indexing and query optimization. 4. Regularly backup and monitor databases to ensure data security and performance optimization.
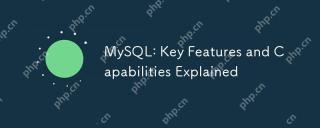
MySQL is an open source relational database management system that is widely used in Web development. Its key features include: 1. Supports multiple storage engines, such as InnoDB and MyISAM, suitable for different scenarios; 2. Provides master-slave replication functions to facilitate load balancing and data backup; 3. Improve query efficiency through query optimization and index use.
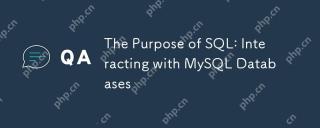
SQL is used to interact with MySQL database to realize data addition, deletion, modification, inspection and database design. 1) SQL performs data operations through SELECT, INSERT, UPDATE, DELETE statements; 2) Use CREATE, ALTER, DROP statements for database design and management; 3) Complex queries and data analysis are implemented through SQL to improve business decision-making efficiency.
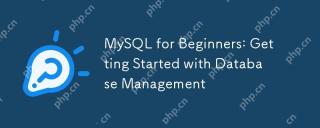
The basic operations of MySQL include creating databases, tables, and using SQL to perform CRUD operations on data. 1. Create a database: CREATEDATABASEmy_first_db; 2. Create a table: CREATETABLEbooks(idINTAUTO_INCREMENTPRIMARYKEY, titleVARCHAR(100)NOTNULL, authorVARCHAR(100)NOTNULL, published_yearINT); 3. Insert data: INSERTINTObooks(title, author, published_year)VA


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
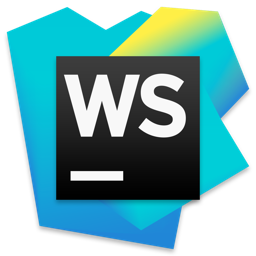
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
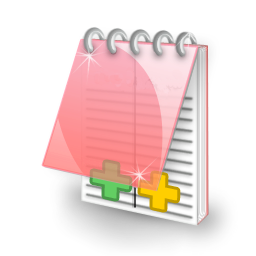
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software