Python Server Programming: Numerical Computation with NumPy
As an efficient, easy-to-learn, and scalable programming language, Python also has advantages in server-side programming. In terms of data processing and numerical calculations, the NumPy library in Python provides powerful functions that can greatly improve the processing speed and efficiency of Python on the server side.
In this article, we will introduce how to program in Python on the server side and perform numerical calculations using NumPy. We'll walk through the basic concepts of NumPy and provide example programs to help you better understand how to use it to perform numerical calculations.
1. What is NumPy?
NumPy is a Python library that provides a large number of mathematical tools and functions for processing and calculating numerical data. The purpose of NumPy is to become the basic library for numerical calculations in Python. It allows users to perform numerical calculations using efficient array operations, and provides a variety of mathematical functions and functions such as quick sorting, random number generation, and file I/O.
NumPy introduces a new data type - "ndarray", that is, n-dimensional array (N-dimensional array), also known as NumPy array. It is a multi-dimensional array composed of elements of the same type and can store not only numeric data but also any other data type.
2. How to install NumPy?
You can use pip to install NumPy, which is a package manager in Python that can help us quickly install and upgrade libraries. You can use the following code in the terminal command to install NumPy:
pip install numpy
3. Create a NumPy array
In Python, we can use the NumPy library to create multi-dimensional array objects. Here are the different ways to create a NumPy array:
1. Using lists in Python
You can create a NumPy array using lists in Python. Here is an example:
import numpy as np my_list = [1, 2, 3] my_array = np.array(my_list)
Output:
[1 2 3]
2. Using functions in NumPy
In the NumPy library, there are many functions that can create arrays, such as "arange ()" function, which creates an array using syntax similar to the range() function in Python. The following is an example:
import numpy as np my_array = np.arange(10)
Output:
[0 1 2 3 4 5 6 7 8 9]
3. Using random functions
NumPy also provides some random functions that can be used to generate arrays of random numbers. The following is an example:
import numpy as np my_random_array = np.random.rand(5)
Output:
[0.94326482 0.19496915 0.80260931 0.28997978 0.2489395 ]
4. Manipulating NumPy arrays
The NumPy library provides some powerful functions for operating arrays, which can be used in different mathematics Computing and data processing. The following are some commonly used functions for operating arrays:
1. Array addition and subtraction
NumPy arrays can be added and subtracted, as shown below:
import numpy as np a = np.array([1,2,3]) b = np.array([4,5,6]) c = a + b d = a - b print(c) print(d)
Output:
[5 7 9] [-3 -3 -3]
2. Array multiplication and division
NumPy arrays can be multiplied and divided as follows:
import numpy as np a = np.array([1,2,3]) b = np.array([4,5,6]) c = a * b d = a / b print(c) print(d)
Output:
[ 4 10 18] [0.25 0.4 0.5 ]
3. Transpose
You can use NumPy's "transpose()" function to perform the transpose operation of the array, as shown below:
import numpy as np a = np.array([[1,2,3],[4,5,6]]) b = np.transpose(a) print(b)
Output:
[[1 4] [2 5] [3 6]]
5. Use NumPy Performing mathematical operations
The NumPy library provides a number of mathematical functions that can be used to perform various mathematical operations on arrays. The following are some commonly used mathematical functions:
1. Exponentiation operation
You can use the "power()" function in the NumPy library to perform exponentiation operations, as shown below:
import numpy as np a = np.array([1,2,3]) b = np.power(a, 2) print(b)
Output:
[1 4 9]
2. Find the square root
You can use the "sqrt()" function in the NumPy library to perform the square root operation, as shown below:
import numpy as np a = np.array([4,9,16]) b = np.sqrt(a) print(b)
Output:
[2. 3. 4.]
3. Find the exponential function
You can use the "exp()" function in the NumPy library to perform exponential operations, as shown below:
import numpy as np a = np.array([1,2,3]) b = np.exp(a) print(b)
Output:
[ 2.71828183 7.3890561 20.08553692]
6. Use NumPy to process large amounts of data
For server-side development, data processing speed and efficiency are very important. Using NumPy can help us process large amounts of data quickly and efficiently. The following is a sample program for calculating statistical values of some large amounts of data:
import numpy as np # 生成随机数据 data = np.random.rand(1000000) # 计算平均值和方差 mean = np.mean(data) variance = np.var(data) print('平均值:{}'.format(mean)) print('数据方差:{}'.format(variance))
Output:
平均值:0.500170053072905 数据方差:0.08331254680620618
7. Summary
NumPy is a very easy-to-use tool in Python The library provides many powerful mathematical functions and tools that can help us better process numerical data. Using NumPy, you can quickly calculate complex mathematical formulas and process large amounts of data, improving the speed and efficiency of server-side development.
The above is the detailed content of Python Server Programming: Numerical Computation with NumPy. For more information, please follow other related articles on the PHP Chinese website!
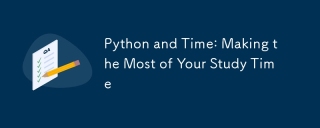
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
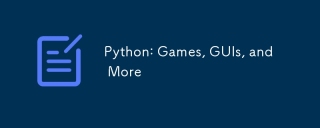
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
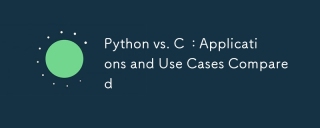
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
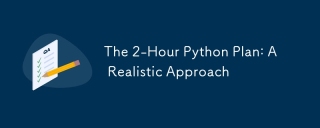
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
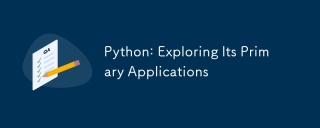
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
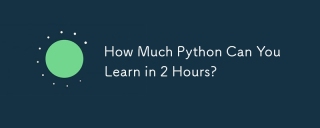
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
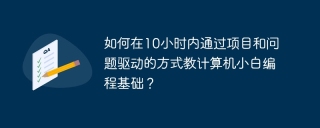
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
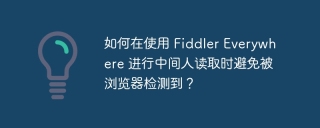
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
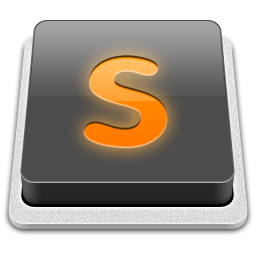
SublimeText3 Mac version
God-level code editing software (SublimeText3)
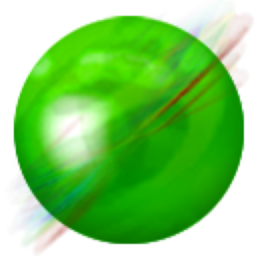
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
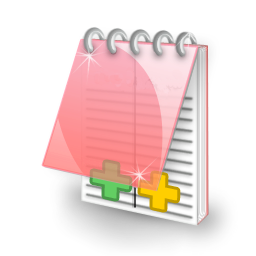
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function