MySQL database and Go language: How to segment data?
As the amount of data grows, database performance and scalability become more and more important. For relational databases like MySQL, how to handle segmented data is a very important issue. To solve this problem, many techniques have been invented, one of which is using Go language for data segmentation processing.
In this article, we will introduce how the MySQL database and the Go language can be used together to implement data segmentation processing. We will explore how to use Go to write an application to connect to a MySQL database, how to use MySQL delimiters to achieve data splitting and how to use Go to process segmented data.
How to connect to MySQL database using Go language
To connect to MySQL database, we need to use the MySQL driver in Go language. Currently, the most popular MySQL driver in Go language is "github.com/go-sql-driver/mysql". To use this driver, we need to download it using the "go get" command.
Next, we need to use the driver in our code. We can use the following code to connect to the MySQL database:
import ( "database/sql" _ "github.com/go-sql-driver/mysql" ) func main() { // 创建一个与数据库的连接 db, err := sql.Open("mysql", "user:password@tcp(localhost:3306)/dbname") // 检查错误 if err != nil { panic(err.Error()) } // 关闭连接 defer db.Close() }
In the above code, we use the "sql.Open" method to create the connection. The first parameter of this method is the driver name and the second parameter is the connection string. The connection string contains username, password, hostname, port number, and database name.
Once we have established a connection to the MySQL database, we can start using the Go language to implement data segmentation.
How to use MySQL delimiters for data segmentation
In the MySQL database, we can use delimiters to split data. This is very useful for large data collections as it allows us to process large amounts of data at once.
For example, suppose we have a table named "users" with the following data:
User ID | Username | Password |
---|---|---|
Alice | 123 | |
Bob | 456 | |
Carol | 789 | |
David | 012 | |
Eric | 345 | |
Fred | 678 |
// 设置 MySQL 分隔符为 ";" _, err := db.Exec("DELIMITER ;") if err != nil { panic(err.Error()) } // 开始处理数据 _, err = db.Exec("BEGIN;") if err != nil { panic(err.Error()) } // 分段处理数据 for i := 1; i <= 6; i++ { _, err = db.Exec("INSERT INTO users VALUES (?, ?, ?);", i, "User "+strconv.Itoa(i), strconv.Itoa(i)+strconv.Itoa(i)+strconv.Itoa(i)) if err != nil { panic(err.Error()) } } // 结束处理数据 _, err = db.Exec("COMMIT;") if err != nil { panic(err.Error()) }
In the above code, we first use the "DELIMITER" command to set the MySQL delimiter to ";". Then, we start processing the data, using the "BEGIN" command to start a transaction. In this transaction, we use the "INSERT INTO" command to insert data into the table "users". Finally, we use the "COMMIT" command to end the transaction and commit all changes.
How to use Go language to process segmented data
Now that we have understood how to use MySQL delimiters to split data, we will explore how to use Go language to process these segmented data.
We can use the following code to get segmented data from the MySQL database:
// 设置 MySQL 分隔符为 ";" _, err := db.Exec("DELIMITER ;") if err != nil { panic(err.Error()) } // 开始处理数据 _, err = db.Exec("BEGIN;") if err != nil { panic(err.Error()) } // 分段处理数据 for i := 1; i <= 6; i++ { // 查询数据 rows, err := db.Query("SELECT * FROM users WHERE user_id = ?;", i) if err != nil { panic(err.Error()) } defer rows.Close() // 处理数据 for rows.Next() { var userID int var username string var password string err = rows.Scan(&userID, &username, &password) if err != nil { panic(err.Error()) } fmt.Println("User ID:", userID) fmt.Println("Username:", username) fmt.Println("Password:", password) } // 检查错误 err = rows.Err() if err != nil { panic(err.Error()) } } // 结束处理数据 _, err = db.Exec("COMMIT;") if err != nil { panic(err.Error()) }
In the above code, we first use the "DELIMITER" command to set the MySQL delimiter to ";" . Then, we start processing the data, using the "BEGIN" command to start a transaction. In this transaction, we use the "SELECT" command to query the data. We use the "Query" method to execute this query and the "Scan" method to process the query results. Finally, we use the "COMMIT" command to end the transaction and commit all changes.
Conclusion
In this article, we introduced how to use the Go language and MySQL delimiters to implement data segmentation processing. We learned how to connect to a MySQL database using Go, how to split data using MySQL delimiters and how to process these segmented data using Go.
Data segmentation processing can help us process large data collections more efficiently and improve performance and scalability when processing this data. Using the Go language and MySQL delimiters makes this process easy and intuitive.
The above is the detailed content of MySQL database and Go language: How to segment data?. For more information, please follow other related articles on the PHP Chinese website!
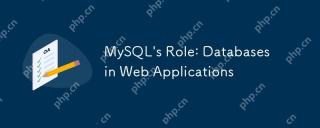
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
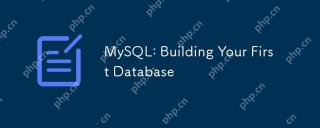
The steps to build a MySQL database include: 1. Create a database and table, 2. Insert data, and 3. Conduct queries. First, use the CREATEDATABASE and CREATETABLE statements to create the database and table, then use the INSERTINTO statement to insert the data, and finally use the SELECT statement to query the data.
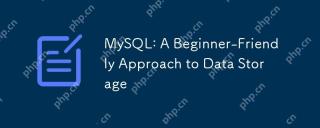
MySQL is suitable for beginners because it is easy to use and powerful. 1.MySQL is a relational database, and uses SQL for CRUD operations. 2. It is simple to install and requires the root user password to be configured. 3. Use INSERT, UPDATE, DELETE, and SELECT to perform data operations. 4. ORDERBY, WHERE and JOIN can be used for complex queries. 5. Debugging requires checking the syntax and use EXPLAIN to analyze the query. 6. Optimization suggestions include using indexes, choosing the right data type and good programming habits.
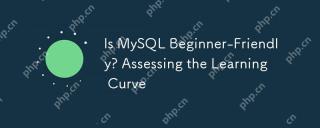
MySQL is suitable for beginners because: 1) easy to install and configure, 2) rich learning resources, 3) intuitive SQL syntax, 4) powerful tool support. Nevertheless, beginners need to overcome challenges such as database design, query optimization, security management, and data backup.
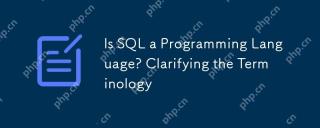
Yes,SQLisaprogramminglanguagespecializedfordatamanagement.1)It'sdeclarative,focusingonwhattoachieveratherthanhow.2)SQLisessentialforquerying,inserting,updating,anddeletingdatainrelationaldatabases.3)Whileuser-friendly,itrequiresoptimizationtoavoidper
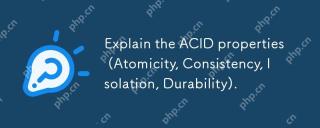
ACID attributes include atomicity, consistency, isolation and durability, and are the cornerstone of database design. 1. Atomicity ensures that the transaction is either completely successful or completely failed. 2. Consistency ensures that the database remains consistent before and after a transaction. 3. Isolation ensures that transactions do not interfere with each other. 4. Persistence ensures that data is permanently saved after transaction submission.
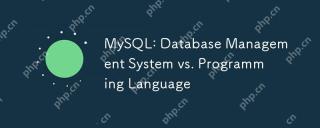
MySQL is not only a database management system (DBMS) but also closely related to programming languages. 1) As a DBMS, MySQL is used to store, organize and retrieve data, and optimizing indexes can improve query performance. 2) Combining SQL with programming languages, embedded in Python, using ORM tools such as SQLAlchemy can simplify operations. 3) Performance optimization includes indexing, querying, caching, library and table division and transaction management.
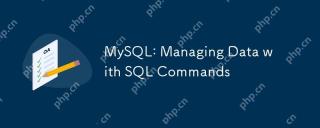
MySQL uses SQL commands to manage data. 1. Basic commands include SELECT, INSERT, UPDATE and DELETE. 2. Advanced usage involves JOIN, subquery and aggregate functions. 3. Common errors include syntax, logic and performance issues. 4. Optimization tips include using indexes, avoiding SELECT* and using LIMIT.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
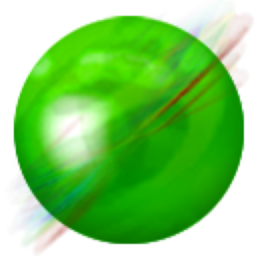
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment
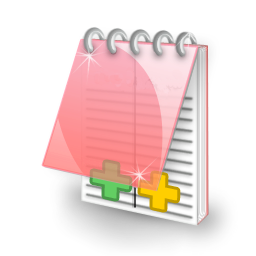
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
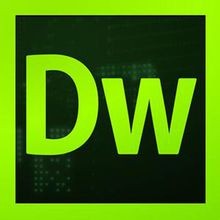
Dreamweaver CS6
Visual web development tools