


With the development of Internet applications and the continuous updating of adopted technologies, data replication and synchronization have increasingly become essential functions for many systems. In the Golang language, many people hope to use the MySQL database for data replication and synchronization. This article will introduce how to use MySQL to achieve data replication and synchronization in the Go language.
- Determine the requirements for replication and synchronization
Before we start to implement data replication and synchronization, we need to first determine the requirements for data replication and synchronization. For example, we need to know which tables require data replication and synchronization, what level of data synchronization is required, whether full data synchronization is required, etc. After determining the needs, we can proceed to the next step.
- Import MySQL library
In order to use the MySQL database, we need to import the MySQL library first. You can use the following command to install the MySQL library:
go get -u github.com/go-sql-driver/mysql
After the installation is complete, we can use the following command to import the MySQL library:
import ( "database/sql" _ "github.com/go-sql-driver/mysql" )
- Connect to the MySQL database
Before connecting to the MySQL database, we need to establish the MySQL database first. After it is established, we need to know the address, user name, password and other information of the MySQL database in order to correctly connect to the MySQL database.
func connectDB() error { var err error db, err = sql.Open("mysql", "username:password@tcp(address:port)/database") if err != nil { log.Printf("连接数据库失败:%s", err.Error()) return err } return nil }
- Query the tables that need to be replicated and synchronized
After connecting to the MySQL database, we can query the tables that need to be replicated and synchronized. The query method can use SQL statements to query, and then use Golang for processing.
func getTables() ([]string, error) { rows, err := db.Query("show tables") if err != nil { log.Printf("查询表失败:%s", err.Error()) return nil, err } var tables []string for rows.Next() { var table string err = rows.Scan(&table) if err != nil { log.Printf("获取表名失败:%s", err.Error()) continue } tables = append(tables, table) } return tables, nil }
- Copy data
After querying the tables that need to be copied and synchronized, we can start copying the data. In the process of data copying, we need to use Golang's concurrency mechanism to speed up data copying.
func copyData() { tables, err := getTables() if err != nil { return } var wg sync.WaitGroup for _, table := range tables { wg.Add(1) go func(table string) { defer wg.Done() rows, err := db.Query(fmt.Sprintf("select * from %s", table)) if err != nil { log.Printf("查询表失败:%s", err.Error()) return } for rows.Next() { // 复制数据到指定的位置 } }(table) } wg.Wait() }
- Data synchronization
In the process of data synchronization, we need to use MySQL's master-slave replication mechanism. For the specific implementation of master-slave replication, please refer to the official documentation of MySQL. In the process of achieving synchronization, we also need to use Golang's concurrency mechanism.
func syncData() { statements, err := getSyncStatements() if err != nil { return } var wg sync.WaitGroup for _, statement := range statements { wg.Add(1) go func(s string) { defer wg.Done() _, err := db.Exec(s) if err != nil { log.Printf("同步数据失败:%s", err.Error()) } }(statement) } wg.Wait() }
Using the above method, you can easily implement data replication and synchronization of MySQL database in Go language. At the same time, it can also better meet the needs of the system.
The above is the detailed content of Use MySQL to implement data replication and synchronization in Go language. For more information, please follow other related articles on the PHP Chinese website!
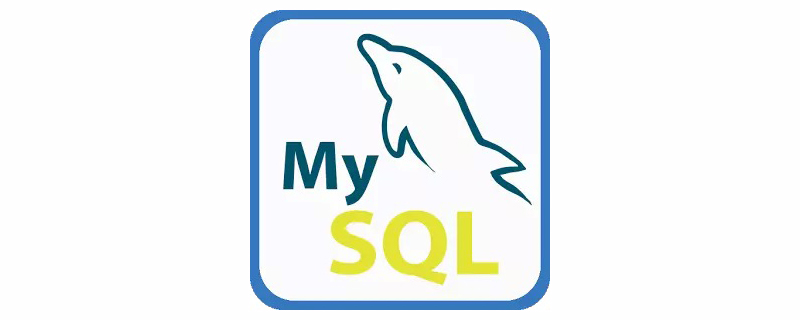
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于架构原理的相关内容,MySQL Server架构自顶向下大致可以分网络连接层、服务层、存储引擎层和系统文件层,下面一起来看一下,希望对大家有帮助。
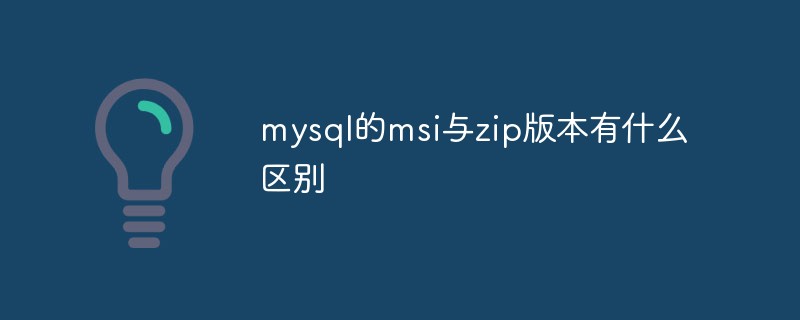
mysql的msi与zip版本的区别:1、zip包含的安装程序是一种主动安装,而msi包含的是被installer所用的安装文件以提交请求的方式安装;2、zip是一种数据压缩和文档存储的文件格式,msi是微软格式的安装包。
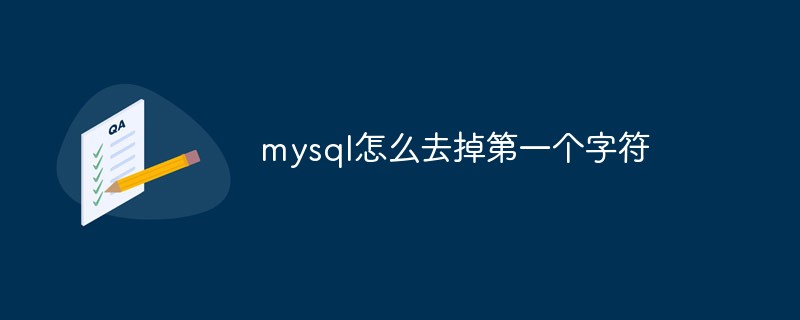
方法:1、利用right函数,语法为“update 表名 set 指定字段 = right(指定字段, length(指定字段)-1)...”;2、利用substring函数,语法为“select substring(指定字段,2)..”。
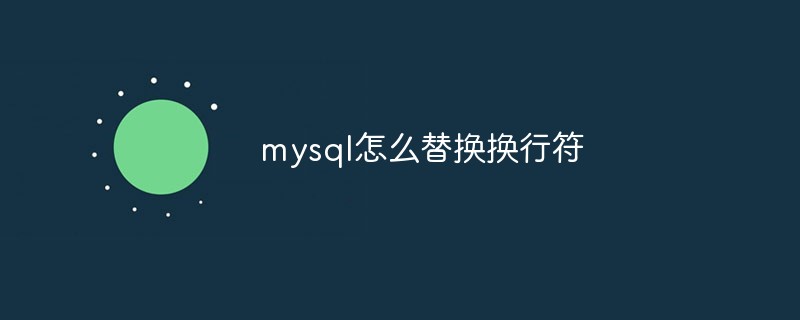
在mysql中,可以利用char()和REPLACE()函数来替换换行符;REPLACE()函数可以用新字符串替换列中的换行符,而换行符可使用“char(13)”来表示,语法为“replace(字段名,char(13),'新字符串') ”。
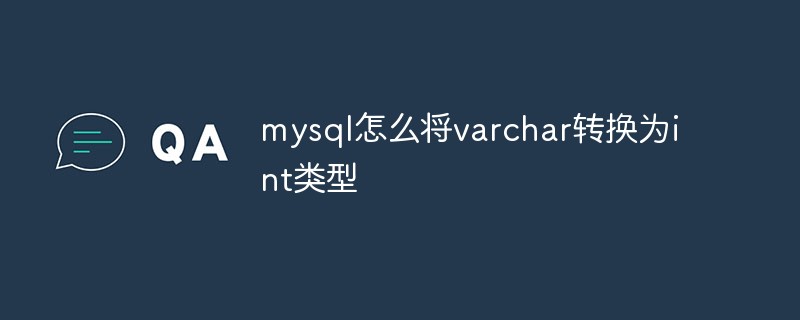
转换方法:1、利用cast函数,语法“select * from 表名 order by cast(字段名 as SIGNED)”;2、利用“select * from 表名 order by CONVERT(字段名,SIGNED)”语句。
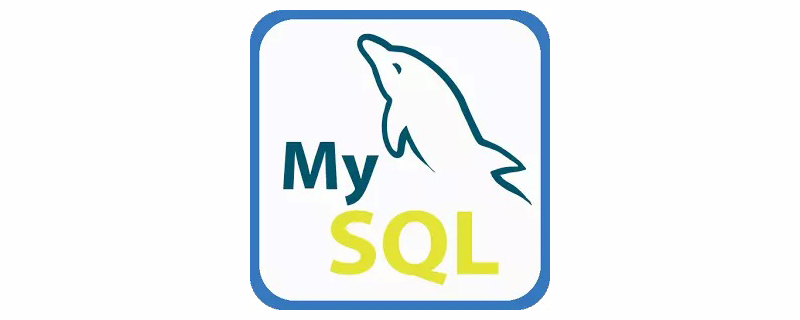
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于MySQL复制技术的相关问题,包括了异步复制、半同步复制等等内容,下面一起来看一下,希望对大家有帮助。
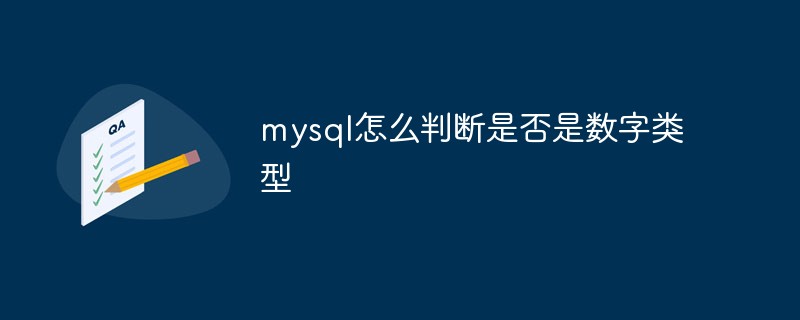
在mysql中,可以利用REGEXP运算符判断数据是否是数字类型,语法为“String REGEXP '[^0-9.]'”;该运算符是正则表达式的缩写,若数据字符中含有数字时,返回的结果是true,反之返回的结果是false。
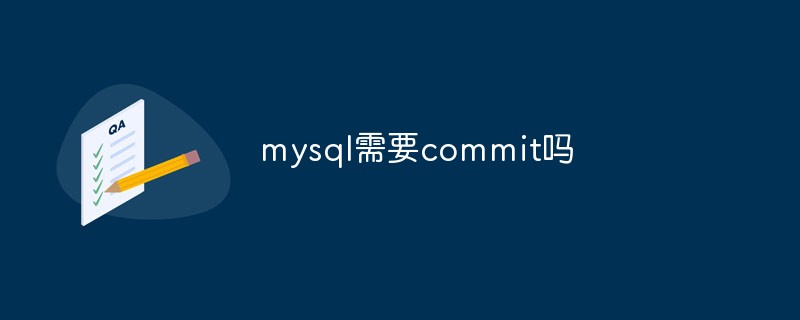
在mysql中,是否需要commit取决于存储引擎:1、若是不支持事务的存储引擎,如myisam,则不需要使用commit;2、若是支持事务的存储引擎,如innodb,则需要知道事务是否自动提交,因此需要使用commit。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
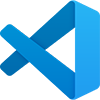
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
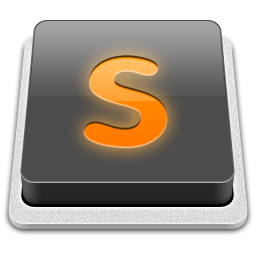
SublimeText3 Mac version
God-level code editing software (SublimeText3)
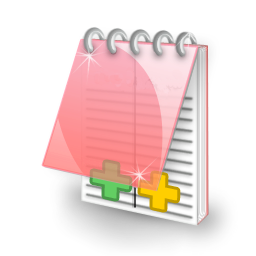
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
