With the development of web applications, API authentication has become an integral part of modern web applications. JSON Web Token (JWT) is an open standard for authentication and authorization that provides a secure and reliable way to authenticate users and implement single sign-on. This article will explain how to use JWT for API authentication in PHP.
What is JWT?
JSON Web Token (JWT) is a standard based on the JSON format, which can be used to securely transmit information over the Internet. JWT usually consists of three parts: header, payload and signature.
- Header: Contains the algorithm used by JWT, such as HMAC SHA256 or RSA.
- Payload: Contains data about users, permissions, or other related information.
- Signature: used to verify the authenticity and integrity of the Token.
JWT workflow
When the user logs in, the server will assign a JWT to the user. This JWT will be sent to the client and saved in the client's cookie or local storage. When a user wants to access a protected API, the client sends a JWT back to the server. The server will check the JWT's signature and verify the data in the payload to ensure the user has permission to access the API. If the JWT passes validation, the server authorizes the user to access the API.
Using PHP to implement JWT authentication
In PHP, we can use some popular open source libraries to implement JWT authentication. This article will use Firebase's JWT library, which provides a simple API to create and verify JWTs. Here is a simple example:
- Install Firebase JWT library:
composer require firebase/php-jwt
- Create JWT:
The following code will create a JWT that contains a signature and custom payload data.
use FirebaseJWTJWT; // Set the payload data (custom data) $payload = [ "user_id" => 1, "username" => "john.doe", ]; // Generate JWT using secret key $jwt = JWT::encode($payload, 'secret_key');
- Verify JWT:
The code below will verify the signature of the JWT and check if the payload data is valid.
use FirebaseJWTJWT; // Verify JWT using secret key try { $decoded = JWT::decode($jwt, 'secret_key', array('HS256')); } catch (Exception $e) { // Handle invalid JWT } // Access the payload data $user_id = $decoded->user_id; $username = $decoded->username;
- Send JWT to client:
After creating the JWT, we can send it to the client and store it in a cookie or local storage.
// Set JWT as a cookie setcookie('token', $jwt, time()+3600, '/');
- Verify the JWT sent by the client:
We can use similar code as the server side to verify the JWT sent by the client.
use FirebaseJWTJWT; // Get JWT from cookie or local storage $jwt = $_COOKIE['token']; // Verify JWT using secret key try { $decoded = JWT::decode($jwt, 'secret_key', array('HS256')); } catch (Exception $e) { // Handle invalid JWT } // Access the payload data $user_id = $decoded->user_id; $username = $decoded->username;
Summary
JWT is a secure and reliable authentication mechanism suitable for modern web applications. JWT authentication can be easily implemented using PHP and Firebase JWT library. When implementing API authentication, make sure to only store JWTs in a secure place and use HTTPS for protected transmission.
The above is the detailed content of How to use JWT for API authentication in PHP. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
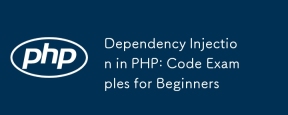
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
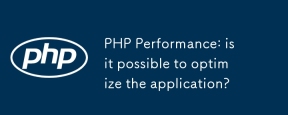
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
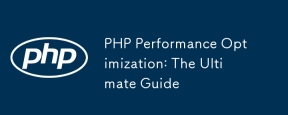
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
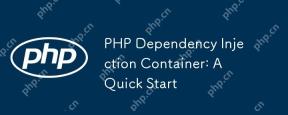
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
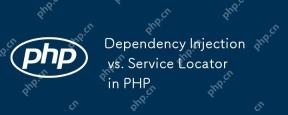
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
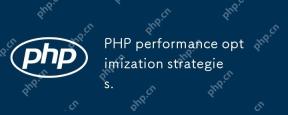
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
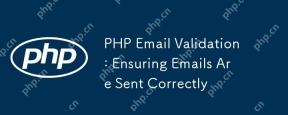
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
