How to use OpenID Connect for API authentication in PHP
In today's digital era, API authentication has become a widely used technical means. To keep API information secure, many websites and applications use the OpenID Connect protocol for authentication. This protocol allows you to use a third-party identity provider (IdP) to authenticate users and provide your API with the ability to exchange identity tokens and access tokens. In this article, we will explore how to use OpenID Connect for API authentication in PHP.
- Install PHP development package
First, you need to install the relevant PHP development package of OpenID Connect in your PHP project to use the OpenID Connect API in your application . If you use the Composer Action Manager, execute the following command in your project root directory to download and install:
composer require php-openid/php-openid-connect
If you don't Using Composer, you can install it manually by downloading the OpenID Connect PHP package from GitHub and placing it in your project directory.
- Register your application
To start using the OpenID Connect protocol, you need to register your application with the provider of your choice and obtain a client ID and client secret . The registration process may vary depending on the provider you use, but most providers' registrations are set up based on the OAuth2 specification.
- Writing Authentication Code
Once you have installed the OpenID Connect package into your PHP project and registered your application, you can start writing the authentication code.
In this example, we will use Auth0 as the open ID provider. First, you need to set up configuration options, filling in your client ID and client secret:
require_once "vendor/autoload.php"; $config = [ 'auth0' => [ 'domain' => 'YOUR_AUTH0_DOMAIN', 'client_id' => 'YOUR_AUTH0_CLIENT_ID', 'client_secret' => 'YOUR_AUTH0_CLIENT_SECRET', 'redirect_uri' => 'YOUR_AUTH0_REDIRECT_URI', 'scope' => 'openid profile email' ] ];
Next, you need to create an authentication object and build the authentication URL:
$auth0 = new Auth0SDKAuth0($config['auth0']); $auth_url = $auth0->get_login_url();
This will present the user with a login page where they will need to enter their credentials to authenticate. Once the user has authenticated, the code will be redirected to the provided URI by setting the redirect URI.
You then need to obtain the access token by getting the authorization code:
$auth0 = new Auth0SDKAuth0($config['auth0']); $access_token = $auth0->get_access_token();
At this point, you have successfully completed authentication and obtained the access token from the OpenID Connect exchange service. You can now use this access token to access the protected API endpoint and authorize user requests with it.
- Use access token to access API
You can use the obtained access token to make requests to the protected API endpoint to access relevant data and resource.
$auth0 = new Auth0SDKAuth0($config['auth0']); $access_token = $auth0->get_access_token(); $api_url = "https://example.com/api/protected-endpoint"; $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_RETURNTRANSFER => 1, CURLOPT_URL => $api_url, CURLOPT_HTTPHEADER => array( 'Authorization: Bearer ' . $access_token, 'Content-Type: application/json' ) )); $response = curl_exec($curl); curl_close($curl); echo $response;
In the above example, we use cURL to make a GET request to access the API endpoint using the obtained access token. Notice that we set the Authorization header in the request header and use the Bearer token type with the access token.
Conclusion
By using OAuth 2.0 and the OpenID Connect protocol, implementing API authentication in PHP is no longer a problem. By following the above steps and code examples correctly, you can ensure that your applications and API endpoints are secure and reliable in terms of authentication.
The above is the detailed content of How to use OpenID Connect for API authentication in PHP. For more information, please follow other related articles on the PHP Chinese website!
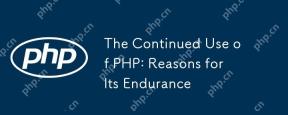
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
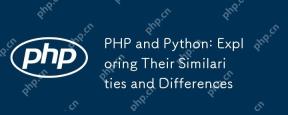
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
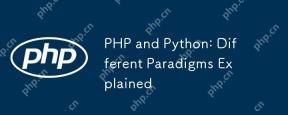
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
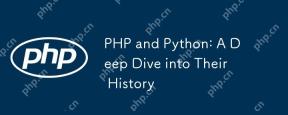
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
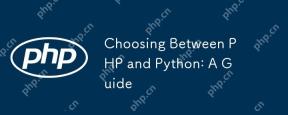
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
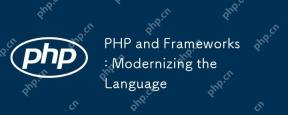
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
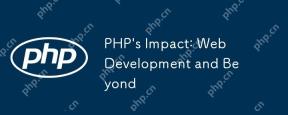
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
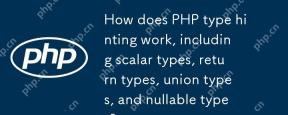
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
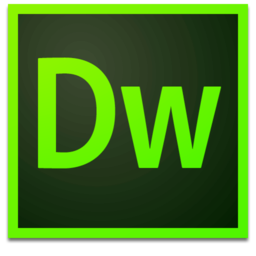
Dreamweaver Mac version
Visual web development tools
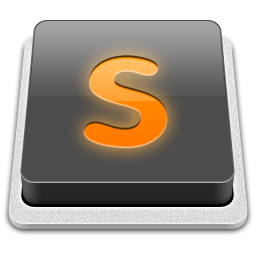
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
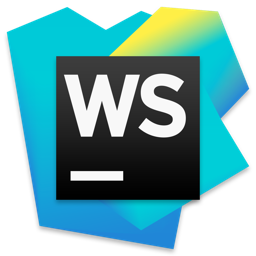
WebStorm Mac version
Useful JavaScript development tools