


In recent years, with the rapid development of Internet technology, the requirements for high performance, high concurrency and high availability on the server side are getting higher and higher. As a high-performance, asynchronous and non-blocking network communication framework, Netty is becoming more and more popular. Developer attention and use.
This article will introduce how to use the Netty framework to implement a high-performance API server.
1. What is Netty
Netty is an asynchronous event-driven network application framework based on Java NIO, used to quickly develop high-performance, high-reliability network communication programs, such as clients and server side.
Its core components include Buffer, Channel, EventLoop, Codec, etc. Buffer is Netty's buffer component, Channel provides an abstract network communication interface, EventLoop is Netty's event-driven model, and Codec is a codec. Through these components, the Netty framework can provide high-performance, high-concurrency, and low-latency network communication capabilities.
2. Basic use of Netty
First, we need to introduce Netty dependencies:
<dependency> <groupId>io.netty</groupId> <artifactId>netty-all</artifactId> <version>4.1.42.Final</version> </dependency>
Then, we need to create a Bootstrap object and use this object to start our Netty server:
EventLoopGroup bossGroup = new NioEventLoopGroup(); EventLoopGroup workerGroup = new NioEventLoopGroup(); try{ ServerBootstrap bootstrap = new ServerBootstrap(); bootstrap.group(bossGroup, workerGroup) .channel(NioServerSocketChannel.class) .childHandler(new ChannelInitializer<SocketChannel>() { @Override public void initChannel(SocketChannel ch) throws Exception { ChannelPipeline pipeline = ch.pipeline(); pipeline.addLast(new HttpServerCodec()); pipeline.addLast(new HttpObjectAggregator(65536)); pipeline.addLast(new ChunkedWriteHandler()); pipeline.addLast(new HttpServerHandler()); } }); ChannelFuture future = bootstrap.bind(port).sync(); future.channel().closeFuture().sync(); }finally{ bossGroup.shutdownGracefully(); workerGroup.shutdownGracefully(); }
In the above code, we created two EventLoopGroup objects, a bossGroup for receiving client requests, and a workerGroup for processing client requests. Configure the parameters of the Netty server through the ServerBootstrap object, including the communication protocol (NioServerSocketChannel), processor (Handler), and Channel initialization and other operations.
We can also see that in the above code, we added the HttpServerCodec and HttpObjectAggregator components to implement encoding, decoding and aggregation of HTTP requests and responses. At the same time, we also added ChunkedWriteHandler to process big data streams.
Finally, we bind the port and start the Netty server through the bootstrap.bind method, and block the main thread and wait for the Netty server to shut down through the future.channel().closeFuture().sync() method.
3. Use Netty to implement high-performance API server
For an API server, we usually need to handle a large number of requests and responses while ensuring system availability and high-performance response time.
Here, we take the implementation of a simple API server as an example to introduce how to use the Netty framework to implement a high-performance API server.
1. Interface definition
Let’s first define a simple API interface. This interface is used to implement the function of obtaining user information:
GET /user/{id} HTTP/1.1 Host: localhost:8888
where {id} is the user The ID number, we need to query the user information based on this ID number and return it to the client.
2. Business processing
Next, we need to implement business logic processing, that is, query user information based on the ID number in the client request, and return the query results to the client.
First, let's create a processor HttpServerHandler, which inherits from SimpleChannelInboundHandler. We can implement our business logic in this processor.
public class HttpServerHandler extends SimpleChannelInboundHandler<FullHttpRequest> { @Override protected void channelRead0(ChannelHandlerContext ctx, FullHttpRequest msg) throws Exception { HttpServerRoute route = HttpServerRoute.builder() .addRoute("/user/{id}", new GetUserHandler()) .build(); HttpServerRequest request = new HttpServerRequest(msg); HttpServerResponse response = new HttpServerResponse(ctx, msg); route.route(request, response); } }
As you can see, in the above code, we implement route matching through the HttpServerRoute object. When receiving a client request, we will convert the request into an HttpServerRequest object, wrap the response object HttpServerResponse in it, then match the routing rules through the HttpServerRoute object, and distribute the request to the corresponding processor for processing.
We need to implement the GetUserHandler processor, which is used to query user information based on the user ID:
public class GetUserHandler implements HttpServerHandlerInterface { @Override public void handle(HttpServerRequest request, HttpServerResponse response) throws Exception { String id = request.getPathParam("id"); //查询用户信息 User user = UserService.getUserById(id); if (user != null) { JSONObject json = new JSONObject(); json.put("id", user.getId()); json.put("name", user.getName()); response.sendJSON(HttpResponseStatus.OK, json.toJSONString()); } else { response.sendError(HttpResponseStatus.NOT_FOUND); } } }
In the above code, we will query the user information based on the ID number in the request, and Use JSONObject to construct the JSON string data of the request response, and finally return the query results to the client.
We also need to implement the UserService class to provide the function of querying user information:
public class UserService { public static User getUserById(String id) { //查询数据库中的用户信息 } }
3. Performance test
Finally, let’s test the high performance of Netty we implemented API server response time and QPS (number of concurrent requests per second).
Through the Apache ab tool, we can simulate multiple client concurrent requests and collect statistics on response time and QPS information. Use the following command:
ab -n 10000 -c 100 -k http://localhost:8888/user/1
Parameter description:
-n: indicates the total number of requests
-c: indicates the number of concurrent requests
-k: indicates Enable Keep-alive connection
Through the test, we can get the response time and QPS information:
Server Software: Server Hostname: localhost Server Port: 8888 Document Path: /user/1 Document Length: 36 bytes Concurrency Level: 100 Time taken for tests: 3.777 seconds Complete requests: 10000 Failed requests: 0 Keep-Alive requests: 10000 Total transferred: 1460000 bytes HTML transferred: 360000 bytes Requests per second: 2647.65 [#/sec] (mean) Time per request: 37.771 [ms] (mean) Time per request: 0.378 [ms] (mean, across all concurrent requests) Transfer rate: 377.12 [Kbytes/sec] received Connection Times (ms) min mean[+/-sd] median max Connect: 0 2 1.2 2 10 Processing: 3 32 11.3 32 84 Waiting: 3 32 11.3 32 84 Total: 6 34 11.2 34 86 Percentage of the requests served within a certain time (ms) 50% 34 66% 38 75% 40 80% 42 90% 49 95% 55 98% 64 99% 71 100% 86 (longest request)
As you can see, our API server can effectively handle 100 concurrent requests from the test The simulation can handle 2647.65 requests per second, and the average response time is only 37.771 milliseconds.
4. Summary
Through the above introduction and steps, we have learned how to use Netty as a network communication framework and use it to develop a high-performance API server. Using the Netty framework can greatly improve server performance, making our server have high concurrency, high reliability, low latency and other characteristics. At the same time, the Netty framework also has high scalability and flexibility and can be easily integrated into any application.
As part of the Java back-end development technology stack, using the Netty framework is also one of the skills that must be mastered.
The above is the detailed content of Java backend development: Implementing a high-performance API server using Netty. For more information, please follow other related articles on the PHP Chinese website!
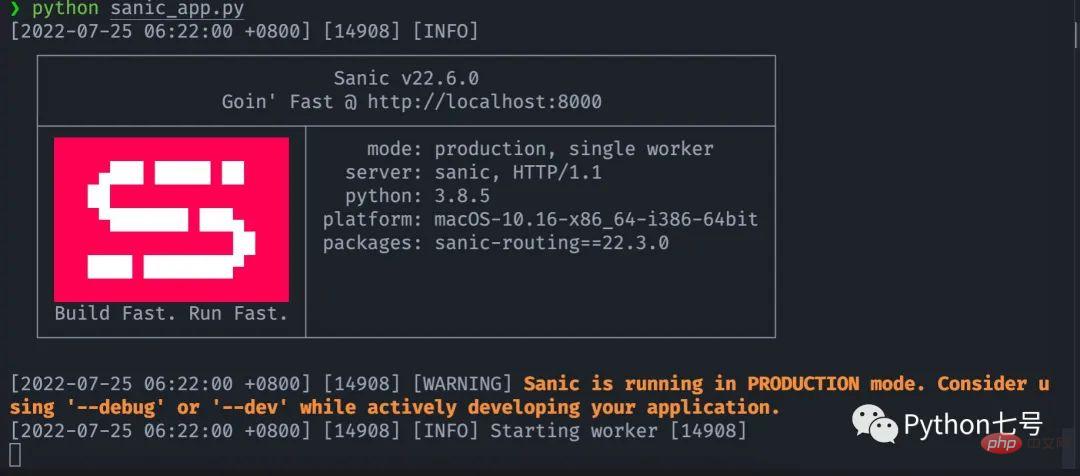
提到API开发,你可能会想到DjangoRESTFramework,Flask,FastAPI,没错,它们完全可以用来编写API,不过,今天分享的这个框架可以让你更快把现有的函数转化为API,它就是Sanic。Sanic简介Sanic[1],是Python3.7+Web服务器和Web框架,旨在提高性能。它允许使用Python3.5中添加的async/await语法,这可以有效避免阻塞从而达到提升响应速度的目的。Sanic致力于提供一种简单且快速,集创建和启动于一体的方法
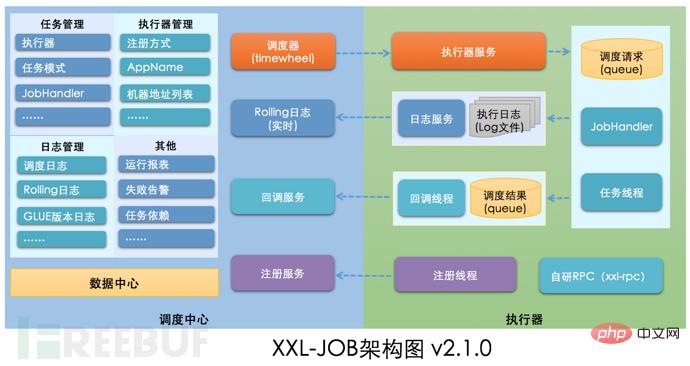
XXL-JOB描述XXL-JOB是一个轻量级分布式任务调度平台,其核心设计目标是开发迅速、学习简单、轻量级、易扩展。现已开放源代码并接入多家公司线上产品线,开箱即用。一、漏洞详情此次漏洞核心问题是GLUE模式。XXL-JOB通过“GLUE模式”支持多语言以及脚本任务,该模式任务特点如下:●多语言支持:支持Java、Shell、Python、NodeJS、PHP、PowerShell……等类型。●WebIDE:任务以源码方式维护在调度中心,支持通过WebIDE在线开发、维护。●动态生效:用户在线通
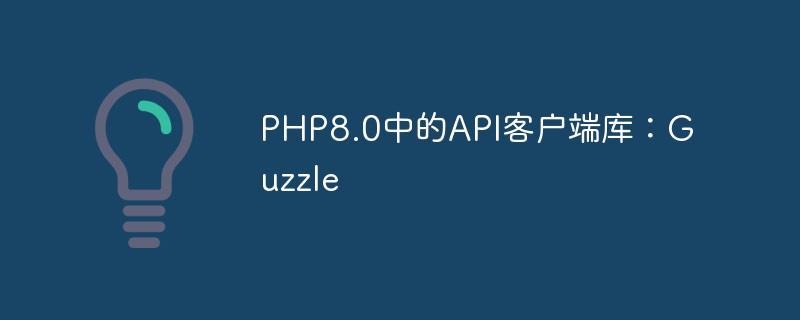
随着网络技术的发展,Web应用程序和API应用程序越来越普遍。为了访问这些应用程序,需要使用API客户端库。在PHP中,Guzzle是一个广受欢迎的API客户端库,它提供了许多功能,使得在PHP中访问Web服务和API变得更加容易。Guzzle库的主要目标是提供一个简单而又强大的HTTP客户端,它可以处理任何形式的HTTP请求和响应,并且支持并发请求处理。在
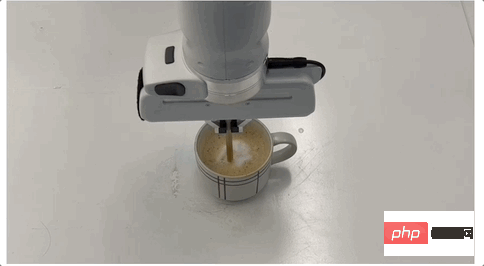
机器人也能干咖啡师的活了!比如让它把奶泡和咖啡搅拌均匀,效果是这样的:然后上点难度,做杯拿铁,再用搅拌棒做个图案,也是轻松拿下:这些是在已被ICLR 2023接收为Spotlight的一项研究基础上做到的,他们推出了提出流体操控新基准FluidLab以及多材料可微物理引擎FluidEngine。研究团队成员分别来自CMU、达特茅斯学院、哥伦比亚大学、MIT、MIT-IBM Watson AI Lab、马萨诸塞大学阿默斯特分校。在FluidLab的加持下,未来机器人处理更多复杂场景下的流体工作也都
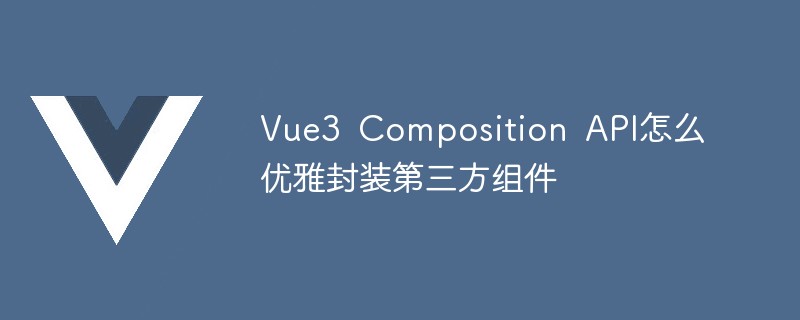
前言对于第三方组件,如何在保持第三方组件原有功能(属性props、事件events、插槽slots、方法methods)的基础上,优雅地进行功能的扩展了?以ElementPlus的el-input为例:很有可能你以前是这样玩的,封装一个MyInput组件,把要使用的属性props、事件events和插槽slots、方法methods根据自己的需要再写一遍://MyInput.vueimport{computed}from'vue'constprops=define
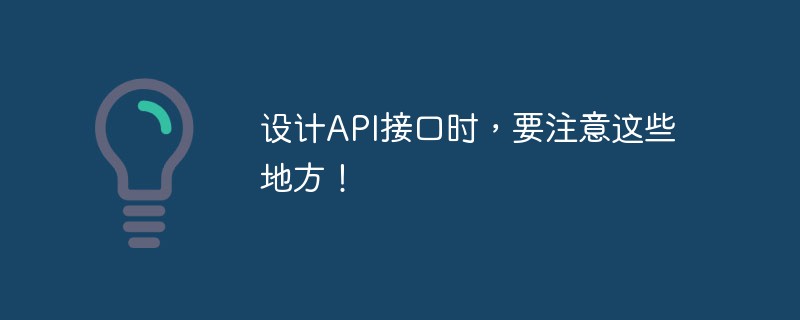
本篇文章给大家带来了关于API的相关知识,其中主要介绍了设计API需要注意哪些地方?怎么设计一个优雅的API接口,感兴趣的朋友,下面一起来看一下吧,希望对大家有帮助。
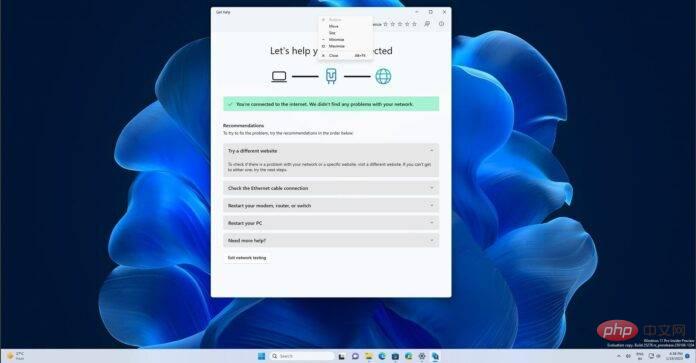
当您的WindowsPC出现网络问题时,问题出在哪里并不总是很明显。很容易想象您的ISP有问题。然而,Windows笔记本电脑上的网络并不总是顺畅的,Windows11中的许多东西可能会突然导致Wi-Fi网络中断。随机消失的Wi-Fi网络是Windows笔记本电脑上报告最多的问题之一。网络问题的原因各不相同,也可能因Microsoft的驱动程序或Windows而发生。Windows是大多数情况下的问题,建议使用内置的网络故障排除程序。在Windows11
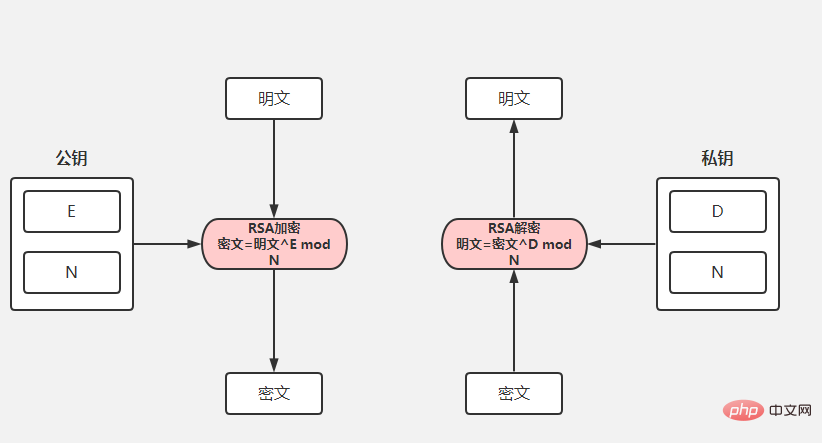
SpringBoot的API加密对接在项目中,为了保证数据的安全,我们常常会对传递的数据进行加密。常用的加密算法包括对称加密(AES)和非对称加密(RSA),博主选取码云上最简单的API加密项目进行下面的讲解。下面请出我们的最亮的项目rsa-encrypt-body-spring-boot项目介绍该项目使用RSA加密方式对API接口返回的数据加密,让API数据更加安全。别人无法对提供的数据进行破解。SpringBoot接口加密,可以对返回值、参数值通过注解的方式自动加解密。什么是RSA加密首先我


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
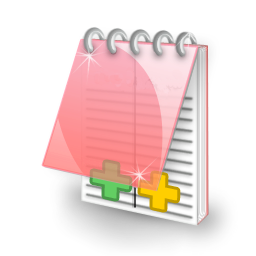
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use
