


Advanced techniques for graphics processing and animation rendering in JavaScript
JavaScript, as a client-side language, is becoming increasingly important in modern web applications. Not only can it be used with HTML and CSS to build dynamic pages, it can also be used for graphics processing and animation rendering. This article will introduce some advanced techniques for graphics processing and animation rendering in JavaScript.
1. Use the Canvas element for graphics processing
First, we will explore the use of the Canvas element in HTML5 to implement graphics processing. The Canvas element is a container for drawing graphics and animations on web pages. It provides rich APIs, including methods for drawing graphics and manipulating pixels. Using the Canvas element, we can create complex graphics and animation effects.
To use the Canvas element for graphics processing, you need to perform the following basic steps:
1. Create the Canvas element
2. Use the getContext() method to obtain the drawing context (context)
3. Use the API in context for graphics drawing
For example, the following are some examples of using the Canvas element API for graphics drawing:
1. Draw a rectangle
var canvas = document.getElementById('myCanvas'); var ctx = canvas.getContext('2d'); ctx.fillStyle = 'blue'; ctx.fillRect(10, 10, 100, 100);
2. Draw a circle
var canvas = document.getElementById('myCanvas'); var ctx = canvas.getContext('2d'); ctx.beginPath(); ctx.arc(75, 75, 50, 0, Math.PI * 2, true); ctx.fillStyle = 'green'; ctx.fill();
3. Draw text
var canvas = document.getElementById('myCanvas'); var ctx = canvas.getContext('2d'); ctx.font = '30px Arial'; ctx.fillStyle = 'red'; ctx.fillText('Hello World', 10, 50);
The above example is just the tip of the iceberg of the Canvas element API. Using the Canvas element, you can create various types of animation and graphic effects.
2. Use CSS3 to achieve animation effects
In addition to the Canvas element, CSS3 also provides many animation effect options. These include transition and animation properties.
1.transition property
The transition property allows you to smoothly transition between two CSS states. For example, you can use it to make a smooth transition to another state when the user hovers over an element.
The following is an example of using the transition attribute:
div{ transition: all 0.2s ease-in-out; } div:hover{ background-color: red; transform: scale(1.5); color: white; }
In this example, when the mouse is hovered over the div element, the background color change, element enlargement and text color change will be applied. Transition effects.
2.animation property
The animation property allows you to create more complex animations by specifying the styles of animations and keyframes.
The following is an example of using the animation attribute:
div{ animation: myanimation 2s infinite; } @keyframes myanimation { 0% { background-color: red; transform: translateX(0); } 50% { background-color: yellow; transform: translateX(200px); } 100% { background-color: blue; transform: translateX(0); } }
In this example, an animation that repeats for 2 seconds will be created that moves a div element from a red background to a blue background , and return to the initial position.
3. Use third-party libraries to speed up development
Finally, if you want to create graphics and animation effects faster, then you can use third-party JavaScript libraries. The following are some widely used libraries:
1.Three.js
Three.js is a JavaScript library for creating 3D graphics and animations on the web. It uses WebGL technology to provide an API that can create complex 3D models and scenes.
2.Pixi.js
Pixi.js is a fast 2D rendering engine that can be used to create interactive animations and games. It uses canvas technology and provides a rich API, including image and text rendering.
3.GreenSock
GreenSock (or GSAP) is an animation library that can be used to create various types of animation effects. It uses JavaScript and provides an API to create complex, smooth and high-performance animations.
The above are some popular JavaScript libraries that can help you achieve complex graphics and animation effects more quickly.
Summary
JavaScript is a powerful language that can be used to implement rich graphics and animation effects in web applications. Using the Canvas element, you can create various types of graphic effects. Using CSS3 you can create dynamic and transition effects. Using third-party JavaScript libraries, you can implement complex graphics and animation effects more quickly. Using the tips mentioned in this article, you can expand your skills in web development.
The above is the detailed content of Advanced techniques for graphics processing and animation rendering in JavaScript. For more information, please follow other related articles on the PHP Chinese website!
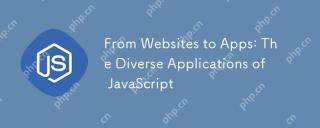
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
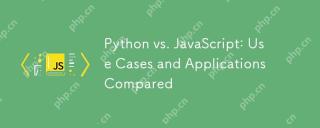
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
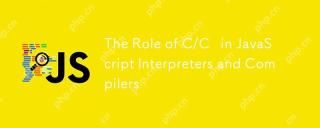
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
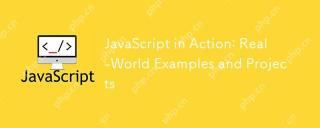
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
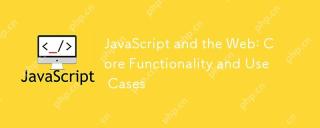
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
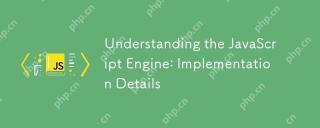
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
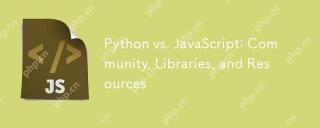
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
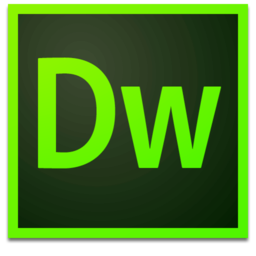
Dreamweaver Mac version
Visual web development tools
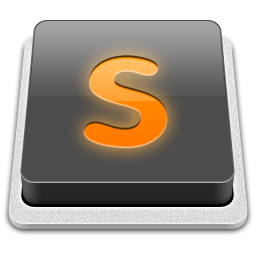
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
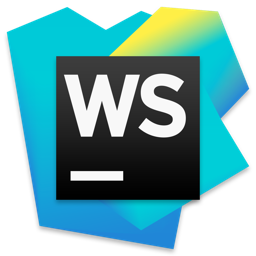
WebStorm Mac version
Useful JavaScript development tools