


Laravel development: How to import and export CSV files using Laravel Excel?
Laravel is one of the outstanding PHP frameworks in the industry. Its powerful functions and easy-to-use API make it very popular among developers. In actual development, we often need to import and export data, and CSV, as a widely used data format, has also become one of the commonly used import and export formats. This article will introduce how to use the Laravel Excel extension to import and export CSV files.
1. Install Laravel Excel
First, we need to use Composer to install Laravel Excel:
composer require maatwebsite/excel
After the installation is complete, we need to add in the config/app.php file Add the following code to the providers array:
MaatwebsiteExcelExcelServiceProvider::class,
Add the following code to the aliases array:
'Excel' => MaatwebsiteExcelFacadesExcel::class,
2. Export the CSV file
Suppose we have a user model User, which has A getExportData() method that returns the data to be exported. Then we can write the export code like this:
use MaatwebsiteExcelFacadesExcel; use AppModelsUser; class UserController extends Controller { public function exportUsers() { return Excel::download(new UserExport(), 'users.csv'); } } class UserExport implements FromQuery, WithHeadings { public function query() { return User::query(); } public function headings(): array { return [ 'ID', 'Name', 'Email', 'Created At', 'Updated At', ]; } }
In the above code, we use Laravel Excel’s FromQuery and WithHeadings interfaces to quickly export data to a CSV file. The FromQuery interface needs to implement a query() method to return the data set to be exported; the WithHeadings interface needs to implement a headings() method to return the header information of the CSV file. In the export operation, we use the Excel::download() method to download, passing in two parameters: the export class UserExport and the file name users.csv.
When users access this route, they can directly download the exported CSV file.
3. Import CSV files
To import CSV files, we need to write an import class and implement the FromCollection interface.
Suppose we have a User import model with an importUsers() method that accepts an uploaded CSV file and imports the data into the database. We can write like this:
use MaatwebsiteExcelFacadesExcel; use AppModelsUser; class UserController extends Controller { public function importUsers(Request $request) { $request->validate([ 'file' => 'required|mimes:csv,txt', ]); $path = $request->file('file')->getRealPath(); $data = Excel::import(new UserImport(), $path); return redirect()->back()->with('success', '导入成功'); } } class UserImport implements FromCollection { public function collection(Collection $rows) { foreach ($rows as $row) { User::create([ 'name' => $row[0], 'email' => $row[1], ]); } } }
In the above code, we define a UserImport class and implement the FromCollection interface. The collection() method here is used to process the data imported from the CSV file and convert it into a Collection object. In this example, we simply create a user and use the first column in the file as the username and the second column as the email address.
When implementing the import function, we need to use the Excel::import() method to pass in the import class and file path. This method will return an array of imported data, which we can pass into other processors.
4. Summary
Using the Laravel Excel extension, we can easily import and export CSV data into Laravel applications. In the above example, we used the FromQuery, WithHeadings and FromCollection interfaces to export and import data. Of course, Laravel Excel also supports other interfaces, such as FromArray, WithTitle, WithMapping, etc. In actual projects, we can choose the appropriate interface to complete specific data import and export work based on actual needs.
The above is the detailed content of Laravel development: How to import and export CSV files using Laravel Excel?. For more information, please follow other related articles on the PHP Chinese website!

Methods to ensure that distributed team members have fair access to tools and resources include: 1) using low-bandwidth alternatives, such as asynchronous video or text updates, to solve connection problems; 2) setting up core overlapping working hours and providing flexible working hours to manage time zone differences; 3) adapt to different cultural needs through translation functions and cultural awareness training. These strategies help create an inclusive and efficient remote working environment.

Forenhancingremotecollaboration,aninstantmessagingtoolmusthave:1)reliabilityforconsistentmessagedelivery,2)anintuitiveuserinterfaceforeasynavigation,3)real-timenotificationstostayupdated,4)seamlessfilesharingforefficientdocumentexchange,5)integration

Thebiggestchallengeofmanagingdistributedteamsiscommunication.Toaddressthis,usetoolslikeSlack,Zoom,andGitHub;setclearexpectations;fostertrustandautonomy;implementasynchronousworkpatterns;andintegratetaskmanagementwithcommunicationplatformsforefficient

Laravel's latest version has significantly improved security, including: 1. Enhanced CSRF protection, through a more robust token verification mechanism; 2. Improved SQL injection protection, through an enhanced query construction method; 3. Better session encryption to ensure user data security; 4. Improved authentication system, supporting finer granular user authentication and multi-factor authentication (MFA).

Tonavigateschedulingconflictsinaglobalworkforce,usetechnology,empathy,andstrategicplanning:1)EmploytoolslikeWorldTimeBuddyorCalendlyforscheduling;2)Rotatemeetingtimestoensurefairness;3)Establishcorehoursforoverlap;4)Beculturallysensitiveandflexiblewi

In Laravel full-stack development, effective methods for managing APIs and front-end logic include: 1) using RESTful controllers and resource routing management APIs; 2) processing front-end logic through Blade templates and Vue.js or React; 3) optimizing performance through API versioning and paging; 4) maintaining the separation of back-end and front-end logic to ensure maintainability and scalability.

Totackleculturalintricaciesindistributedteams,fosteranenvironmentcelebratingdifferences,bemindfulofcommunication,andusetoolsforclarity.1)Implementculturalexchangesessionstosharestoriesandtraditions.2)Adjustcommunicationmethodstosuitculturalpreference

Toassesstheeffectivenessofremotecommunication,focuson:1)Engagementmetricslikemessagefrequencyandresponsetime,2)Sentimentanalysistogaugeemotionaltone,3)Meetingeffectivenessthroughattendanceandactionitems,and4)Networkanalysistounderstandcommunicationpa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
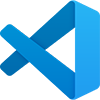
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
