Have you ever faced any challenges while working in distributed teams?
The biggest challenge of managing distributed teams is communication. To address this, use tools like Slack, Zoom, and GitHub; set clear expectations; foster trust and autonomy; implement asynchronous work patterns; and integrate task management with communication platforms for efficient collaboration.
Dealing with distributed teams? Oh, I've been there, and it's a rollercoaster! The biggest challenge? Communication, hands down. When you're not in the same room, or even the same time zone, things can get lost in translation. I remember working on a project where half the team was in San Francisco, and the other half was in Bangalore. The time difference meant that when one team was wrapping up their day, the other was just getting started. Syncing up became a Herculean task.
Let's dive into the world of distributed teams and explore how to navigate these challenges while harnessing the power of modern technology and collaboration tools. By the end of this journey, you'll have a toolkit of strategies to make your distributed team not just survive, but thrive.
When you're working across different continents, understanding the basics of effective communication and collaboration is key. Tools like Slack, Zoom, and GitHub are your best friends. They help bridge the gap, but it's not just about the tools; it's about how you use them. For instance, setting clear expectations on response times and availability can make a world of difference.
Now, let's talk about the real meat of the matter: how to make your distributed team work like a well-oiled machine. The secret sauce? Trust and autonomy. When you can't see your team members every day, you need to trust them to get the job done. Empowering them with the freedom to make decisions can lead to some incredible results. Here's a simple example of how you might set up a project management tool to facilitate this:
# Project Management Tool Setup from datetime import datetime class Task: def __init__(self, name, assignee, due_date): self.name = name self.assignee = assignee self.due_date = due_date self.status = "Pending" def update_status(self, new_status): self.status = new_status class Project: def __init__(self, name): self.name = name self.tasks = [] def add_task(self, task): self.tasks.append(task) def display_tasks(self): for task in self.tasks: print(f"Task: {task.name}, Assignee: {task.assignee}, Due Date: {task.due_date}, Status: {task.status}") # Example usage project = Project("Distributed Team Project") task1 = Task("Implement API", "John Doe", datetime(2023, 12, 1)) task2 = Task("Design UI", "Jane Smith", datetime(2023, 12, 5)) project.add_task(task1) project.add_task(task2) project.display_tasks()
This simple Python script demonstrates how you can manage tasks and keep everyone on the same page, even when they're scattered across the globe. But it's not just about the code; it's about the culture you foster. Encouraging open communication and regular check-ins can help build a sense of camaraderie and shared purpose.
When it comes to advanced strategies, consider implementing asynchronous work patterns. Not everyone can be online at the same time, so why force it? Encourage your team to leave detailed updates on their progress and next steps. This way, when someone logs on, they can pick up right where the last person left off. Here's a more complex example that showcases how you might implement an asynchronous task management system:
# Asynchronous Task Management System import asyncio from datetime import datetime class AsyncTask: def __init__(self, name, assignee, due_date): self.name = name self.assignee = assignee self.due_date = due_date self.status = "Pending" async def update_status(self, new_status): await asyncio.sleep(1) # Simulate some work self.status = new_status class AsyncProject: def __init__(self, name): self.name = name self.tasks = [] async def add_task(self, task): await asyncio.sleep(0.5) # Simulate some work self.tasks.append(task) async def display_tasks(self): for task in self.tasks: print(f"Task: {task.name}, Assignee: {task.assignee}, Due Date: {task.due_date}, Status: {task.status}") async def main(): project = AsyncProject("Distributed Team Project") task1 = AsyncTask("Implement API", "John Doe", datetime(2023, 12, 1)) task2 = AsyncTask("Design UI", "Jane Smith", datetime(2023, 12, 5)) await project.add_task(task1) await project.add_task(task2) await task1.update_status("In Progress") await task2.update_status("In Progress") await project.display_tasks() asyncio.run(main())
This asynchronous approach can help your team work more efficiently, but it's not without its challenges. One common pitfall is the potential for miscommunication. When team members are working at different times, it's easy for updates to be missed or misunderstood. To mitigate this, consider implementing a 'daily digest' system where key updates are summarized and sent out at a regular time.
Performance optimization in a distributed team context often revolves around efficient communication and task management. For instance, using tools like Jira or Trello can help visualize workflows and keep everyone aligned. Here's a snippet of how you might integrate a task management tool with a communication platform:
# Task Management Integration with Communication Platform class TaskIntegration: def __init__(self, task_manager, communication_platform): self.task_manager = task_manager self.communication_platform = communication_platform def notify_task_update(self, task): message = f"Task '{task.name}' updated to '{task.status}' by {task.assignee}" self.communication_platform.send_message(message) def sync_tasks(self): tasks = self.task_manager.get_tasks() for task in tasks: if task.status != "Pending": self.notify_task_update(task) # Example usage class MockTaskManager: def get_tasks(self): return [Task("Implement API", "John Doe", datetime(2023, 12, 1), "In Progress"), Task("Design UI", "Jane Smith", datetime(2023, 12, 5), "In Progress")] class MockCommunicationPlatform: def send_message(self, message): print(f"Sending message: {message}") integration = TaskIntegration(MockTaskManager(), MockCommunicationPlatform()) integration.sync_tasks()
This integration ensures that everyone stays in the loop, but it's crucial to balance automation with human touch. Over-reliance on automated notifications can lead to notification fatigue, so it's important to set up filters and prioritize critical updates.
In terms of best practices, fostering a culture of transparency and accountability is paramount. Regular stand-ups, even if they're asynchronous, can keep the team aligned. Additionally, celebrating successes and learning from failures can boost morale and drive continuous improvement.
In conclusion, working with distributed teams is challenging, but with the right tools, strategies, and mindset, it can be incredibly rewarding. By embracing communication, trust, and autonomy, you can turn the challenges into opportunities for growth and innovation.
The above is the detailed content of Have you ever faced any challenges while working in distributed teams?. For more information, please follow other related articles on the PHP Chinese website!

Methods to ensure that distributed team members have fair access to tools and resources include: 1) using low-bandwidth alternatives, such as asynchronous video or text updates, to solve connection problems; 2) setting up core overlapping working hours and providing flexible working hours to manage time zone differences; 3) adapt to different cultural needs through translation functions and cultural awareness training. These strategies help create an inclusive and efficient remote working environment.

Forenhancingremotecollaboration,aninstantmessagingtoolmusthave:1)reliabilityforconsistentmessagedelivery,2)anintuitiveuserinterfaceforeasynavigation,3)real-timenotificationstostayupdated,4)seamlessfilesharingforefficientdocumentexchange,5)integration

Thebiggestchallengeofmanagingdistributedteamsiscommunication.Toaddressthis,usetoolslikeSlack,Zoom,andGitHub;setclearexpectations;fostertrustandautonomy;implementasynchronousworkpatterns;andintegratetaskmanagementwithcommunicationplatformsforefficient

Laravel's latest version has significantly improved security, including: 1. Enhanced CSRF protection, through a more robust token verification mechanism; 2. Improved SQL injection protection, through an enhanced query construction method; 3. Better session encryption to ensure user data security; 4. Improved authentication system, supporting finer granular user authentication and multi-factor authentication (MFA).

Tonavigateschedulingconflictsinaglobalworkforce,usetechnology,empathy,andstrategicplanning:1)EmploytoolslikeWorldTimeBuddyorCalendlyforscheduling;2)Rotatemeetingtimestoensurefairness;3)Establishcorehoursforoverlap;4)Beculturallysensitiveandflexiblewi

In Laravel full-stack development, effective methods for managing APIs and front-end logic include: 1) using RESTful controllers and resource routing management APIs; 2) processing front-end logic through Blade templates and Vue.js or React; 3) optimizing performance through API versioning and paging; 4) maintaining the separation of back-end and front-end logic to ensure maintainability and scalability.

Totackleculturalintricaciesindistributedteams,fosteranenvironmentcelebratingdifferences,bemindfulofcommunication,andusetoolsforclarity.1)Implementculturalexchangesessionstosharestoriesandtraditions.2)Adjustcommunicationmethodstosuitculturalpreference

Toassesstheeffectivenessofremotecommunication,focuson:1)Engagementmetricslikemessagefrequencyandresponsetime,2)Sentimentanalysistogaugeemotionaltone,3)Meetingeffectivenessthroughattendanceandactionitems,and4)Networkanalysistounderstandcommunicationpa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
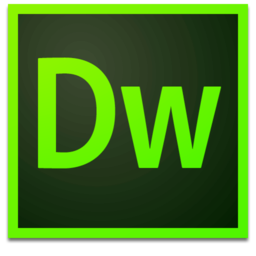
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
