


Laravel Development: How to handle subscription payments using Laravel Cashier and Braintree?
Laravel Development: How to handle subscription payments using Laravel Cashier and Braintree?
In today’s subscription economy, many businesses and startups offer a variety of subscription services, from music, movies, games to cloud storage and applications, and more. If you are a developer, you need to build a system that can handle subscription payments. In this article, we will explain how to achieve this using Laravel Cashier and Braintree.
Laravel Cashier is a Laravel extension that provides a simple yet powerful interface to handle subscriptions and payments. Braintree is a global online payment processing platform that makes it easy to accept various payment methods.
We will use Laravel 8 and Braintree for specific implementation. You need to install Laravel Cashier and Braintree extensions in your project. The following are the specific implementation steps:
- Set environment variables
First, you need to set environment variables in the project to store the Braintree API key and other settings. You can set environment variables in .env files. Here is an example of using a Braintree API test key:
BRAINTREE_ENV=sandbox BRAINTREE_MERCHANT_ID=your_merchant_id BRAINTREE_PUBLIC_KEY=your_public_key BRAINTREE_PRIVATE_KEY=your_private_key
- Creating a Braintree Payment Portal
Next, you need to create a payment portal in the Braintree control panel. Each portal has a unique identifier and credentials that will be used to process payments. After creating a portal in the control panel, copy and save your credentials.
- Configuring Braintree
Then, you need to add a Braintree configuration in the config/services.php file. Here is an example configuration:
'braintree' => [ 'environment' => env('BRAINTREE_ENV'), 'merchant_id' => env('BRAINTREE_MERCHANT_ID'), 'public_key' => env('BRAINTREE_PUBLIC_KEY'), 'private_key' => env('BRAINTREE_PRIVATE_KEY'), ],
- Creating a Subscription Plan
Now you can create a subscription plan using Laravel Cashier and Braintree. A subscription plan is a service that is charged a recurring fee, such as monthly or annually. Here is an example of how to create a subscription plan:
use LaravelCashierSubscription; $subscription = Subscription::create([ 'name' => 'Monthly Subscription Plan', 'stripe_id' => 'monthly-subscription-plan', 'stripe_plan' => 'monthly', 'quantity' => 1, 'trial_period_days' => 14, ]); $user->subscriptions()->save($subscription);
In this example, we create a subscription plan called "Monthly Subscription Plan", its stripe_id is "monthly-subscription-plan", stripe_plan is "monthly". The 'quantity' parameter specifies the quantity of the subscription, and the 'trial_period_days' parameter specifies the number of days of the trial period. After saving the subscription information, the user will be able to subscribe to the plan.
- Processing Payments
Finally, you need to process your subscription payments. Luckily, Laravel Cashier already handles the payment details for us. With Laravel Cashier, you can easily process payments without worrying about the complexities of payment gateways. Here is an example of handling a payment:
use LaravelCashierCashier; $user = User::find(1); $paymentMethod = $user->defaultPaymentMethod(); $payment = $user->charge(10, $paymentMethod); if ($user->subscribed('monthly-subscription-plan')) { // 更新用户的订阅和付款信息 $user->subscription('monthly-subscription-plan')->update([ 'stripe_id' => $payment->id, 'ends_at' => null, ]); }
In this example, we use the $user->defaultPaymentMethod() method to get the user's default payment method and charge them $10. If the subscription plan is "monthly-subscription-plan", we will update the user's subscription and payment information.
Conclusion
Processing subscription payments has become easier with Laravel Cashier and Braintree. You can easily create and manage subscription plans, and process payments. Of course, this is just a simple example and you can add more functionality to your own projects. Hope this article helps you!
The above is the detailed content of Laravel Development: How to handle subscription payments using Laravel Cashier and Braintree?. For more information, please follow other related articles on the PHP Chinese website!

Laravel stands out by simplifying the web development process and delivering powerful features. Its advantages include: 1) concise syntax and powerful ORM system, 2) efficient routing and authentication system, 3) rich third-party library support, allowing developers to focus on writing elegant code and improve development efficiency.

Laravelispredominantlyabackendframework,designedforserver-sidelogic,databasemanagement,andAPIdevelopment,thoughitalsosupportsfrontenddevelopmentwithBladetemplates.

Laravel and Python have their own advantages and disadvantages in terms of performance and scalability. Laravel improves performance through asynchronous processing and queueing systems, but due to PHP limitations, there may be bottlenecks when high concurrency is present; Python performs well with the asynchronous framework and a powerful library ecosystem, but is affected by GIL in a multi-threaded environment.

Laravel is suitable for projects that teams are familiar with PHP and require rich features, while Python frameworks depend on project requirements. 1.Laravel provides elegant syntax and rich features, suitable for projects that require rapid development and flexibility. 2. Django is suitable for complex applications because of its "battery inclusion" concept. 3.Flask is suitable for fast prototypes and small projects, providing great flexibility.

Laravel can be used for front-end development. 1) Use the Blade template engine to generate HTML. 2) Integrate Vite to manage front-end resources. 3) Build SPA, PWA or static website. 4) Combine routing, middleware and EloquentORM to create a complete web application.

PHP and Laravel can be used to build efficient server-side applications. 1.PHP is an open source scripting language suitable for web development. 2.Laravel provides routing, controller, EloquentORM, Blade template engine and other functions to simplify development. 3. Improve application performance and security through caching, code optimization and security measures. 4. Test and deployment strategies to ensure stable operation of applications.

Laravel and Python have their own advantages and disadvantages in terms of learning curve and ease of use. Laravel is suitable for rapid development of web applications. The learning curve is relatively flat, but it takes time to master advanced functions. Python's grammar is concise and the learning curve is flat, but dynamic type systems need to be cautious.

Laravel's advantages in back-end development include: 1) elegant syntax and EloquentORM simplify the development process; 2) rich ecosystem and active community support; 3) improved development efficiency and code quality. Laravel's design allows developers to develop more efficiently and improve code quality through its powerful features and tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
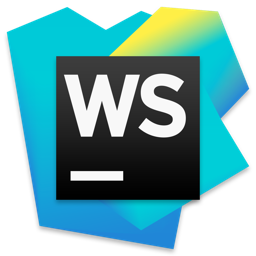
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
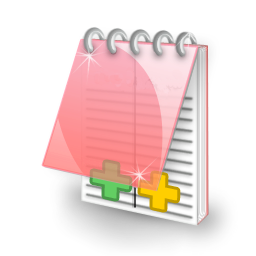
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software