


Swoole asynchronous programming practice: improve web service performance ten times
With the rapid development of the Internet, more and more companies have begun to get involved in Web development. How to improve the performance of Web services has become a key issue. In recent years, asynchronous programming has gradually become popular as a technology to improve network IO efficiency, and the Swoole framework is one of the representatives of asynchronous programming. In this article, we will introduce how to implement asynchronous programming through the Swoole framework and demonstrate its significant effect in improving the performance of web services.
1. What is Swoole
Swoole is a high-performance, asynchronous, and concurrent network communication framework. It makes it easier for PHP developers to write asynchronous code and improve code efficiency and performance. Swoole provides TCP/UDP/Unix domain Socket, HTTP server, WebSocket server, as well as asynchronous text, JSON serialization and deserialization functions. Currently, Swoole is favored by more and more PHP developers.
2. Several points to note when using Swoole
1. Enable coroutines:
In Swoole, in order to support asynchronous programming, we need to enable coroutines. Coroutines are a more lightweight scheduling method than threads because there is no additional overhead of context switching and kernel-mode resources.
Using Swoole It is very convenient to use coroutines. You only need to add the following code to the entry file or Swoole server object:
SwooleRuntime::enableCoroutine();
In this way, you can use the coroutine function provided by Swoole.
2. Pay attention to memory leaks:
When using Swoole for asynchronous programming, you need to pay attention to memory leaks. Because the coroutine in asynchronous programming will wait for I/O for a long time, if the memory is not released in time, it will cause a waste of memory.
Swoole provides a method to clean up the coroutine context: Coroutine::defer(). Use it to clean up the context at the end of the coroutine, for example:
SwooleCoroutineun(function () { echo "Coroutine Start "; Coroutine::defer(function () { echo "Coroutine End "; }); });
3. Pay attention to the version of Swoole:
The new version of Swoole will continue to be optimized and improved, so we need to use the latest version of. At the same time, you need to pay attention to the changes in each version to ensure the compatibility and stability of the code.
3. Swoole Practice: Improving Web Service Performance
Below we use a simple example to demonstrate how to use the Swoole framework to improve Web service performance.
We first create a simple PHP file server.php. This file will listen to the local 9501 port and return a Hello World string:
<?php $http = new SwooleHttpServer("0.0.0.0", 9501); $http->on("request", function ($request, $response) { $response->header("Content-Type", "text/plain"); $response->end("Hello World! "); }); $http->start();
Use the command line to run this file and access http://127.0.0.1:9501/, you can see that Hello World is output.
Now we change the code of this server to asynchronous mode:
<?php $http = new SwooleHttpServer("0.0.0.0", 9501, SWOOLE_BASE); $http->on("request", function ($request, $response) { $response->header("Content-Type", "text/plain"); $response->end("Hello World! "); }); $http->start();
In the above code, we added the third parameter, which is to use SWOOLE_BASE mode to start the server. In this way, we can use the coroutines, asynchronous IO and event listening functions provided by Swoole.
Next, we will use the Apache Bench tool to test the performance of the server when handling a large number of requests.
The Apache Bench tool can simulate real HTTP requests. We can use the multi-threaded concurrent requests it provides to simulate multiple users accessing the server at the same time and test the performance of the server under different request loads.
Enter the following command in the terminal to install the Apache Bench tool:
# ubuntu sudo apt-get install apache2-utils # centos sudo yum install httpd-tools
Use the following command to test the server performance just now:
ab -n 1000 -c 100 http://127.0.0.1:9501
In this command, we use the -n parameter to indicate The total number of requests, -c indicates the number of concurrent requests. We set the total number of requests to 1000 and the total number of concurrent requests to 100.
After the test is completed, we can see the test results printed by Apache Bench:
Concurrency Level: 100 Time taken for tests: 0.041 seconds Complete requests: 1000 Failed requests: 0 Total transferred: 110000 bytes HTML transferred: 12000 bytes Requests per second: 24540.63 [#/sec] (mean) Time per request: 4.075 [ms] (mean) Time per request: 0.041 [ms] (mean, across all concurrent requests) Transfer rate: 2624.27 [Kbytes/sec] received Connection Times (ms) min mean[+/-sd] median max Connect: 0 0 0.2 0 1 Processing: 1 4 0.5 4 6 Waiting: 0 4 0.5 4 6 Total: 1 4 0.5 4 6 Percentage of the requests served within a certain time (ms) 50% 4 66% 4 75% 4 80% 4 90% 4 95% 5 98% 5 99% 5 100% 6 (longest request)
We can see that when this server processes 1000 requests, the average response time of each request is 4.075 milliseconds, the number of response requests per second is approximately 24540. This performance result is already very good.
Next, we increase the load of the server to see how the Swoole framework performs under high concurrency conditions. We increase the number of concurrent requests to 1000, that is:
ab -n 10000 -c 1000 http://127.0.0.1:9501
After the test is completed, we see the test results printed by Apache Bench again:
Concurrency Level: 1000 Time taken for tests: 2.437 seconds Complete requests: 10000 Failed requests: 0 Total transferred: 1100000 bytes HTML transferred: 120000 bytes Requests per second: 4107.95 [#/sec] (mean) Time per request: 243.651 [ms] (mean) Time per request: 0.244 [ms] (mean, across all concurrent requests) Transfer rate: 441.50 [Kbytes/sec] received Connection Times (ms) min mean[+/-sd] median max Connect: 0 8 84.5 0 1000 Processing: 1 22 16.0 20 176 Waiting: 0 21 16.0 20 176 Total: 1 30 86.2 20 1001 Percentage of the requests served within a certain time (ms) 50% 20 66% 23 75% 25 80% 26 90% 30 95% 41 98% 52 99% 65 100% 1001 (longest request)
You can see that when the number of concurrent requests reaches 1000 , the response time of this server is only about 200ms. Compared with synchronous web servers with non-asynchronous programming, Swoole can greatly improve concurrency and performance.
4. Summary
This article introduces the Swoole framework and its application in improving the performance of web services. We learned how to use Swoole to start coroutines, pay attention to memory leaks, and how to test the performance of Swoole asynchronous servers.
In practice, we can use efficient tools such as Swoole and Apache Bench tools to improve the performance of web services. In high-concurrency scenarios on the Internet, using Swoole for asynchronous programming can greatly improve server performance and meet the needs of enterprises for high-performance web services.
The above is the detailed content of Swoole asynchronous programming practice: improve web service performance ten times. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
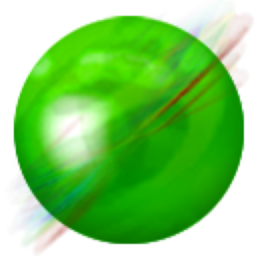
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor
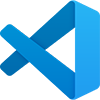
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment