What are the common variables in PHP programming?
In PHP programming, variables are the basic unit of storing values and are used to store and use data during program execution. In PHP, variables can be assigned different data types, including integers, floating point, strings, arrays, etc. In this article, we will introduce common variables and their usage in PHP programming.
- Simple variables
Simple variables are the most common variable type. They can store regular data types such as integers, floating point numbers, and strings. In PHP, the initial value of undefined variables is NULL. The following are a few examples:
Integer variable:
$num1 = 12; $num2 = -345; $num3 = 0x80 ;
Floating point variable:
$float1 = 1.234; $float2 = 10.2e3; $float3 = 4E-10;
String variable:
$str1 = "Hello World!"; $str2 = 'PHP is great!';
- Index array
An index array is a collection of values controlled by a numeric index key. It is usually used to store a set of ordered data. In PHP, we can create an indexed array using the array()
function. The following is an example:
$colors = array("Red", "Green", "Blue");
The values of an array can be accessed using its index value, for example:
echo $colors[0]; // 输出 "Red" echo $colors[1]; // 输出 "Green" echo $colors[2]; // 输出 "Blue"
You can also use a loop structure to traverse the array:
foreach($colors as $value){ echo $value . "<br>"; }
When traversing the array , you can use key
and value
to represent key values and array element values:
foreach($colors as $key => $value){ echo $key . " = " . $value . "<br>"; }
- Associative array
Associative array is A collection of values controlled by a string index key, usually used to store an unordered set of data. In PHP, we can create associative arrays using the array()
function. Here are a few examples:
$age = array("Peter"=>"35", "Ben"=>"37", "Joe"=>"43"); $months = array("Jan"=>"31", "Feb"=>"28", "Mar"=>"31", "Apr"=>"30");
The value of an array can be accessed using its key value, for example:
echo $age["Peter"]; // 输出 "35" echo $months["Jan"]; // 输出 "31"
When traversing an associative array, the foreach
structure can also be used:
foreach($age as $key => $value){ echo $key . " is " . $value . " years old.<br>"; }
- Global variables and local variables
In PHP, variables can be global or local. Global variables are defined and used outside the function, while local variables are defined and used inside the function. Local variables are destroyed when the function completes execution, while global variables exist throughout the execution of the program.
In order to access global variables inside a function, we need to use the global
keyword declaration in the function:
$num = 10; function test(){ global $num; echo $num; } test(); // 输出 "10"
Local variables can also be created and used inside the function:
function test(){ $num = 100; echo $num; } test(); // 输出 "100"
- Static variables
Static variables are local variables defined inside the function, but unlike ordinary local variables, static variables will not is destroyed and its value continues to be saved until the next function call. This is useful when you need to track changes in certain values. The following is an example:
function test(){ static $num = 0; echo $num; $num++; } test(); // 输出 "0" test(); // 输出 "1" test(); // 输出 "2"
At each function call, the value of the static variable $num
continues to increase.
In summary, these are common variable types and usages in PHP programming. Mastering the basic concepts and usage of these variables is very important for developing high-quality PHP programs.
The above is the detailed content of What are the common variables in PHP programming?. For more information, please follow other related articles on the PHP Chinese website!
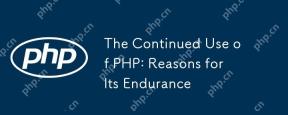
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
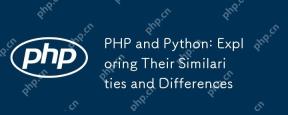
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
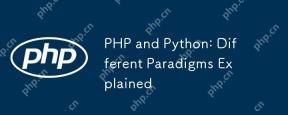
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
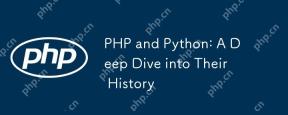
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
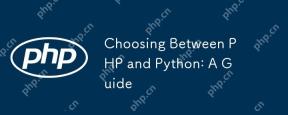
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
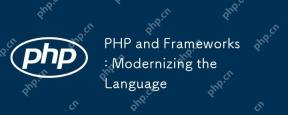
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
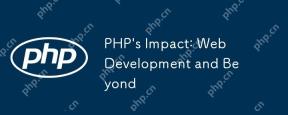
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
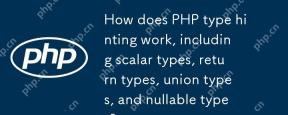
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.