With the continuous development of web applications and services, RESTful API has become an essential part of modern web applications. REST (Representational State Transfer) is a web-based architecture for building scalable and maintainable web services. Due to the advantages of RESTful APIs in data transfer, they are widely used in the design and development of modern applications.
In this article, we will explore how to use RESTful API in PHP programming. We will take an in-depth look at how RESTful APIs work, how to send and receive data using PHP, and how to build a complete web application using RESTful APIs in PHP.
1. What is RESTful API?
RESTful API is a web-based API design that follows the principles of REST architecture. REST architecture is an architecture for building scalable and maintainable web services. It involves the following four key elements:
- Resources: Information or data in a web application.
- Methods: HTTP methods used to access and operate resources, such as GET, POST, PUT and DELETE, etc.
- means: how to represent the data format of the resource, such as JSON, XML, etc.
- URL: A unique URL used to identify and locate a resource.
Through these elements, RESTful API abstracts web services into a set of identifiable and reusable resources, providing an API design method that is easy to extend and maintain.
2. How to use PHP to send and receive RESTful API data?
When programming in PHP, you can use the curl library to send and receive RESTful API data. The curl library is a common tool library for communicating with URLs. It can send HTTP methods such as GET, POST, PUT, and DELETE and receive response data.
The following is a sample code that uses PHP to send a GET request:
$url = 'https://api.example.com/products'; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $output = curl_exec($ch); curl_close($ch); $data = json_decode($output); print_r($data);
In this example, we use the curl library to send a GET request named "products" and save the response data in the $output variable and use json_decode to parse the response data. Finally, use the print_r function to print the parsed data.
3. How to build a RESTful API in PHP?
When programming in PHP, you can use the Slim framework to build a RESTful API. The Slim framework is a lightweight PHP framework with flexible routing capabilities, middleware support, and easy extensibility. The following is a sample code for building a RESTful API using the Slim framework:
require 'vendor/autoload.php'; $app = new SlimSlim(); $app->get('/products', function () { echo "List of products"; }); $app->get('/products/:id', function ($id) { echo "Product with id: " . $id; }); $app->post('/products', function () { echo "Create a new product"; }); $app->put('/products/:id', function ($id) { echo "Update product with id: " . $id; }); $app->delete('/products/:id', function ($id) { echo "Delete product with id: " . $id; }); $app->run();
In this example, we use the Slim framework to define 5 routes to handle GET, POST, PUT and DELETE requests. These routes are defined by using the corresponding methods of the $app object. For example, $app->get('/products', function () {}) means defining a GET route named "products".
4. Summary
How to use RESTful API in PHP programming? This article answers this question and provides sample code for sending and receiving RESTful API data using the curl library and building a RESTful API using the Slim framework. Using RESTful API can help us better build scalable and maintainable web services, and greatly improve the efficiency and reliability of web applications.
The above is the detailed content of How to use RESTful API in PHP programming?. For more information, please follow other related articles on the PHP Chinese website!
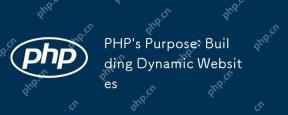
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
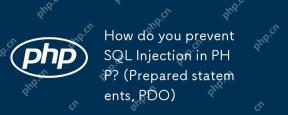
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
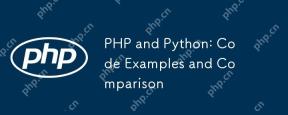
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
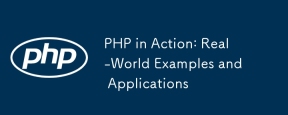
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
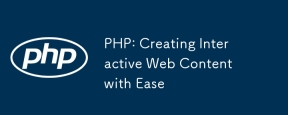
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
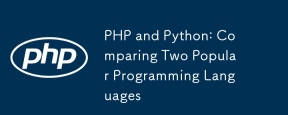
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
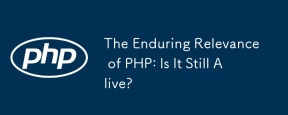
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!
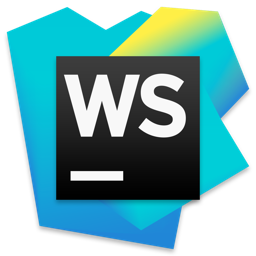
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment