


Differences and applications of abstract classes and interfaces in Java language
Differences and Applications of Abstract Classes and Interfaces in Java Language
In Java language, abstract classes and interfaces are two important concepts. They all share abstract characteristics, but there are also some obvious differences between them. When using Java language for programming, choosing to use abstract classes or interfaces has an important impact on the structural design and scalability of the program.
Abstract class
In the Java language, an abstract class refers to a class that contains abstract methods. Abstract methods refer to methods that have no specific implementation in the method declaration. For example:
abstract class Shape{ public abstract void draw(); public abstract void resize(); }
Abstract classes cannot be instantiated and can only be inherited. Subclasses must implement all abstract methods in the abstract class, unless the subclass is also an abstract class.
The purpose of an abstract class is to provide a basic class that can provide certain common methods and attributes for subclasses. At the same time, subclasses can implement or rewrite these methods according to their own needs, which has a certain degree of flexibility. sex.
When we need to design a class with polymorphic properties, abstract classes are a good choice. For example:
abstract class Animal{ public abstract void move(); } class Cat extends Animal{ public void move(){ System.out.println("跑"); } } class Dog extends Animal{ public void move(){ System.out.println("走"); } }
In the above code, we created an abstract class Animal, which has an abstract method move(), and we created two subclasses Cat and Dog that implement this method. We can obtain their respective move() methods by instantiating Cat and Dog objects. Since they are all subclasses of the Animal class, they can be referenced through variables of type Animal.
Interface
In the Java language, the interface is a special abstract class with the following characteristics:
- The interface cannot be instantiated, but can only be implemented .
- The methods defined in the interface must be public abstract methods.
- The properties defined in the interface must be public static constants.
- An interface can inherit multiple interfaces.
The purpose of interfaces is to create common classes and components. For example:
interface Drawable{ public void draw(); } interface Resizable{ public void resize(); } class Circle implements Drawable, Resizable{ private int radius; public void draw(){ System.out.println("绘制圆形"); } public void resize(){ System.out.println("重设圆形大小"); } }
In the above code, we created two interfaces, Drawable and Resizable, and defined a common method draw() and resize(). Then, we created a Circle class that implemented these two interfaces, and implemented the draw() and resize() methods in the Circle class. In this way, when drawing a circle, you can directly use an instance of the Circle class.
Summary
Abstract classes and interfaces are important concepts in the Java language and are often used in programming. They can all provide abstract templates and common methods to help program designers complete the design of program structure and components.
The specific application of abstract classes and interfaces can be selected according to specific circumstances. If you need to design a class with a specific implementation, you can use an abstract class; if you need to design a general class or component, you can use an interface. In specific use, it needs to be designed according to actual needs to achieve optimal program effects.
The above is the detailed content of Differences and applications of abstract classes and interfaces in Java language. For more information, please follow other related articles on the PHP Chinese website!
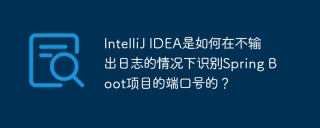
Start Spring using IntelliJIDEAUltimate version...
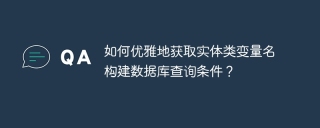
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
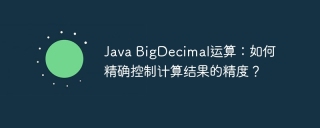
Java...
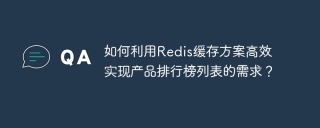
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
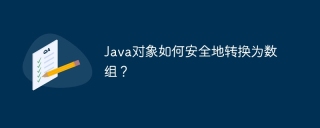
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
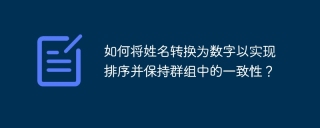
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
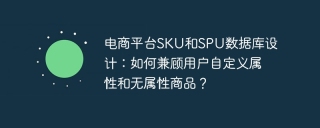
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
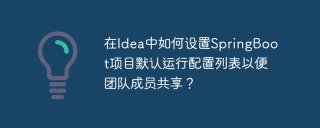
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
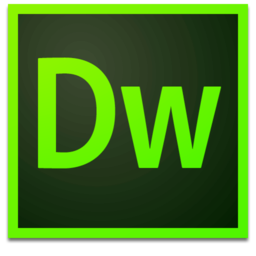
Dreamweaver Mac version
Visual web development tools
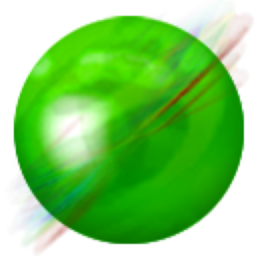
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software