How to implement concurrency safety in Go language?
With the continuous development of computer technology, we must shift from single thread to multi-thread for program processing. Compared with the traditional concurrency processing model, the powerful concurrency processing mechanism of Go language has attracted the attention of many developers. The Go language provides a lightweight implementation mechanism that makes authentic concurrent code easier to write.
However, it is inevitable that a multi-threaded environment will bring many race conditions (Race Condition). When multiple threads try to read and write the same shared resource at the same time, unexpected results may occur due to the uncertainty of the order of execution. Race Condition is one of the potential problems developers fear the most.
In order to avoid potential problems in concurrent processing, the Go language provides a rich variety of standard libraries: sync. This article will introduce the mechanism to achieve concurrency safety through the sync library.
Mutex and RWMutex
mutex is the most commonly used mechanism. At any time, only one coroutine can obtain the mutex object, and other coroutines need to wait for the previous coroutine to release the lock before they can continue execution. Mutex can be used to protect shared resources so that code can run safely and stably.
RWMutex is another mutex lock type, which is equivalent to the extension of mutex in the field of reading and writing. RWMutex contains two counters: read counter and write counter.
- When the reading coroutine is still performing a reading operation, the writing operation will be locked and waits for the reading operation to end.
- When the writing coroutine calls the lock operation, all ongoing read and write operations of the coroutine will be locked.
This mechanism ensures that multiple coroutines can perform read operations at the same time, and only a single coroutine can perform write operations.
var rwMutex sync.RWMutex var count int func read() { rwMutex.RLock() defer rwMutex.RUnlock() fmt.Println(count) } func write() { rwMutex.Lock() defer rwMutex.Unlock() count++ }
In the above example code, we use a RWMutex type lock to protect the read and write operations of the count variable. When a thread calls the write() function, the write counter is locked and all other coroutines are blocked from reading and writing. When a thread calls the read() function, the read counter will be locked and other coroutines will be allowed to perform read operations.
WaitGroup
WaitGroup is used to wait for a group of coroutines to complete execution. Suppose we have n coroutines that need to be executed, then in the main coroutine, we need to call waitGroup.Add(n). WaitGroup.Done() is called after each coroutine has completed execution.
func main() { var wg sync.WaitGroup for i := 0; i < 5; i++ { wg.Add(1) go func(n int) { fmt.Println("goroutine ", n) wg.Done() }(i) } wg.Wait() }
In this example, we use WaitGroup to wait for the execution of each goroutine, and finally wait for all goroutines to complete before ending the main execution process.
Cond
When multiple coroutines need to stop or perform some specific operations, we can use Cond. It is common to use Cond in conjunction with locks and WaitGroup. It allows goroutines to block simultaneously until a condition variable changes.
var cond = sync.NewCond(&sync.RWMutex{}) func printOddNumbers() { for i := 0; i < 10; i++ { cond.L.Lock() if i%2 == 1 { fmt.Println(i) cond.Signal() } else { cond.Wait() } cond.L.Unlock() } } func printEvenNumbers() { for i := 0; i < 10; i++ { cond.L.Lock() if i%2 == 0 { fmt.Println(i) cond.Signal() } else { cond.Wait() } cond.L.Unlock() } }
In the above code example, we used Cond to ensure that even numbers and odd numbers are output separately. Each coroutine uses sync.Mutex to lock the goroutine and wait for another coroutine to first access the shared variable and then monitor the value of the variable.
Once
In some cases, you need to ensure that certain operations are performed only once, such as reading a configuration file only once or initializing global state only once. The sync.Once type of Go language was born for this purpose. When the function is called for the first time it will execute the code inside it and will not be executed again on subsequent calls.
var once sync.Once func doSomething() { once.Do(func() { fmt.Println("Do something") }) }
In the above example, we used sync.Once to safely execute the doSomething function. The first time doSomething is called, the function will be executed only once using once.Do().
Conclusion
In this article, we introduce the locks and mechanisms commonly used in the Go language to ensure the safety of concurrent code. The Mutex, RWMutex, WaitGroup, Cond, and Once types using the sync library are all very powerful and can be used to design safe and efficient concurrent programs. As concurrency mechanisms continue to evolve, understanding the latest advances in concurrent programming is key to keeping your development skills competitive.
The above is the detailed content of How to implement concurrency safety in Go language?. For more information, please follow other related articles on the PHP Chinese website!
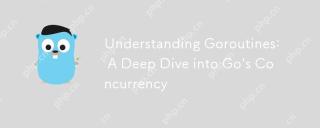
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
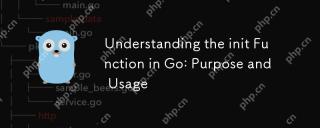
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
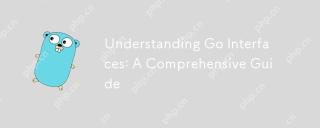
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
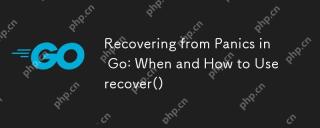
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.
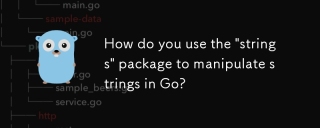
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
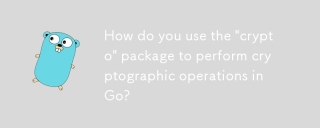
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
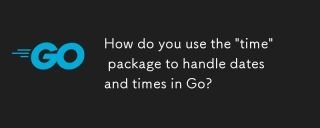
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
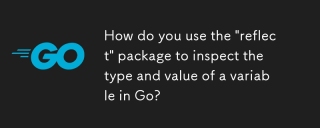
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
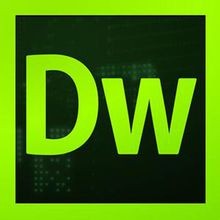
Dreamweaver CS6
Visual web development tools
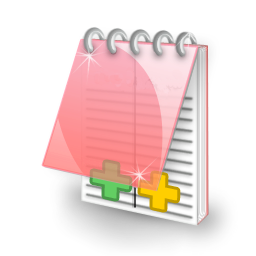
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
