


Detailed explanation of the command pattern in PHP and its application methods
The command pattern is a design pattern that encapsulates requests into an object, allowing you to parameterize the client with different requests, queue or log requests, and supports undoable operations. The core idea of this pattern is to separate the behavior requested by the client from the implementation behavior. The command mode in PHP is widely used in systems that need to record application logs, and systems that need to dynamically execute historical commands.
The core components of the command pattern include commands, receivers and callers. The command object carries the client's operation request and parameters. The receiver is the object that actually executes these requests, and the caller sends the request to the receiver. The advantage of this is that the caller does not need to know which receiver the request will be performed by, this work can be easily achieved through the command object.
A command object usually needs to contain the following parts:
-
execute
method, which will actually execute the command operation. -
undo
method, when the caller needs to cancel the command, this method will restore the recipient to the original state. -
redo
method, when the caller needs to redo the command, this method will restore the receiver to the state of the last execution of the command.
The receiver implements specific operations, such as processing files, performing database operations, etc. The caller is responsible for making requests to the receiver, but does not interact directly with the receiver. The command object acts as a bridge between the caller and the receiver. It separates the specific content of the command from the caller and receiver, allowing the command to be stored, serialized, transmitted, or executed repeatedly.
Below we will use specific examples to further understand the command mode in PHP.
Suppose we need to implement a simple text editor that supports undo and redo operations. First, we need to define an abstract base class Command, which will declare three methods execute
, undo
and redo
.
abstract class Command { abstract public function execute(); abstract public function undo(); abstract public function redo(); }
Then, we need to implement specific commands, such as opening files, saving files, and deleting text.
class OpenFileCommand extends Command { public function __construct(FileReceiver $receiver) { $this->receiver = $receiver; } public function execute() { $this->receiver->openFile(); } public function undo() { $this->receiver->closeFile(); } public function redo() { $this->execute(); } } class SaveFileCommand extends Command { public function __construct(FileReceiver $receiver) { $this->receiver = $receiver; } public function execute() { $this->receiver->saveFile(); } public function undo() { // No need to implement } public function redo() { $this->execute(); } } class DeleteTextCommand extends Command { public function __construct(TextReceiver $receiver) { $this->receiver = $receiver; } public function execute() { $this->receiver->deleteText(); } public function undo() { $this->receiver->insertText(); } public function redo() { $this->execute(); } }
The receiver implements specific operations, such as opening files, saving files, and deleting text.
class FileReceiver { public function openFile() { // Open file } public function closeFile() { // Close file } public function saveFile() { // Save file } } class TextReceiver { private $text = ''; public function insertText($text) { $this->text .= $text; } public function deleteText() { $this->text = substr($this->text, 0, -1); } public function getText() { return $this->text; } }
Finally, we need to implement the device for sending requests to the receiver.
class Invoker { private $commands = []; private $current = 0; public function addCommand(Command $command) { array_splice($this->commands, $this->current); $this->commands[] = $command; $command->execute(); $this->current++; } public function undo() { if ($this->current > 0) { $this->current--; $command = $this->commands[$this->current]; $command->undo(); } } public function redo() { if ($this->current < count($this->commands)) { $command = $this->commands[$this->current]; $command->redo(); $this->current++; } } }
When using a text editor, we can use Invoker to add and undo commands, which will retain the history of commands for subsequent operations. For example:
$invoker = new Invoker(); // Open file $invoker->addCommand(new OpenFileCommand(new FileReceiver())); // Type 'Hello' $textReceiver = new TextReceiver(); $textReceiver->insertText('Hello'); $invoker->addCommand(new DeleteTextCommand($textReceiver)); // Save file $invoker->addCommand(new SaveFileCommand(new FileReceiver())); // Undo $invoker->undo(); // Redo $invoker->redo();
As shown in the above code, we first execute the command to open the file, then add a command to delete text, execute the command, and save the file command. Finally, we use Invoker to undo an operation and repeat it. Do an operation.
In summary, the command pattern in PHP can help us encapsulate requests and separate them from receivers and callers, making the application more modular and easy to extend. We can easily add new functionality to our application simply by creating different command objects. Whether it is a text editor or other applications, the command pattern in PHP is a very useful design pattern.
The above is the detailed content of Detailed explanation of the command pattern in PHP and its application methods. For more information, please follow other related articles on the PHP Chinese website!
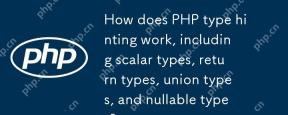
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
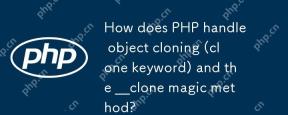
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
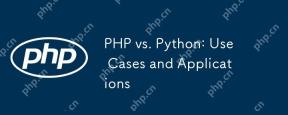
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
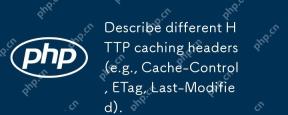
Key players in HTTP cache headers include Cache-Control, ETag, and Last-Modified. 1.Cache-Control is used to control caching policies. Example: Cache-Control:max-age=3600,public. 2. ETag verifies resource changes through unique identifiers, example: ETag: "686897696a7c876b7e". 3.Last-Modified indicates the resource's last modification time, example: Last-Modified:Wed,21Oct201507:28:00GMT.
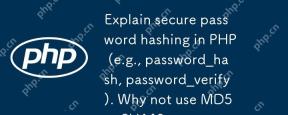
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
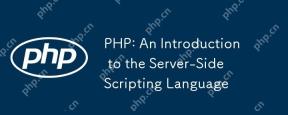
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
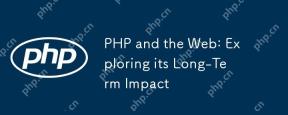
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
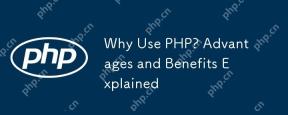
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
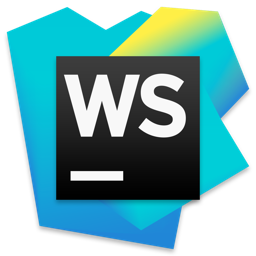
WebStorm Mac version
Useful JavaScript development tools
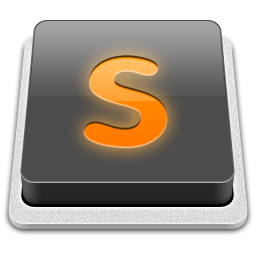
SublimeText3 Mac version
God-level code editing software (SublimeText3)