OAuth2.0 is a protocol used to authorize third-party applications to access user resources. It is now widely used in the Internet field. With the development of Internet business, more and more applications need to support the OAuth2.0 protocol. This article will introduce the best way to implement the OAuth2.0 protocol using PHP.
1. Basic knowledge of OAuth2.0
Before introducing the implementation method of OAuth2.0, we need to understand some basic knowledge of OAuth2.0.
- Authorization type
The OAuth2.0 protocol defines 4 different authorization types: authorization code mode (authorization code), implicit authorization mode (implicit grant), Password mode (resource owner password credentials) and client mode (client credentials). Different authorization types are suitable for different scenarios. The specific authorization type to use needs to be decided based on business needs.
- Roles
The OAuth2.0 protocol defines three roles: resource owner, client and resource server. . The resource owner refers to the authorized user, the client refers to the third-party application, and the resource server refers to the server where the user's resources are stored.
- Process
The process in the OAuth2.0 protocol includes the following steps:
(1) The client requests authorization from the resource owner;
(2) The resource owner agrees to the authorization and issues an authorization code to the client;
(3) The client carries the authorization code to request an access token from the resource server;
( 4) The resource server verifies the authorization code and issues an access token to the client.
2. Implementation method
There are many mature solutions on the market for the implementation of the OAuth2.0 protocol, such as Laravel Passport, PHP League OAuth2 Server, etc. This article will focus on the implementation of PHP League OAuth2 Server.
- Environment setup
First, you need to create a new PHP project and install the PHP League OAuth2 Server component. The installation method is as follows:
composer require league/oauth2-server
Then, you need to create a database and create the following table structure:
``
CREATE TABLE oauth_clients (
client_id VARCHAR(80) NOT NULL, client_secret VARCHAR(80) NOT NULL, redirect_uri VARCHAR(2000) NOT NULL, grant_types VARCHAR(80), scope VARCHAR(4000), user_id VARCHAR(255), CONSTRAINT clients_client_id_pk PRIMARY KEY (client_id)
);
CREATE TABLE oauth_access_tokens (
access_token VARCHAR(40) NOT NULL, client_id VARCHAR(80) NOT NULL, user_id VARCHAR(255), expires TIMESTAMP NOT NULL, scope VARCHAR(4000), CONSTRAINT access_token_pk PRIMARY KEY (access_token)
);
CREATE TABLE oauth_authorization_codes (
authorization_code VARCHAR(40) NOT NULL, client_id VARCHAR(80) NOT NULL, user_id VARCHAR(255), redirect_uri VARCHAR(2000), expires TIMESTAMP NOT NULL, scope VARCHAR(4000), CONSTRAINT auth_code_pk PRIMARY KEY (authorization_code)
);
CREATE TABLE oauth_refresh_tokens (
refresh_token VARCHAR(40) NOT NULL, client_id VARCHAR(80) NOT NULL, user_id VARCHAR(255), expires TIMESTAMP NOT NULL, scope VARCHAR(4000), CONSTRAINT refresh_token_pk PRIMARY KEY (refresh_token)
);
2. 配置服务器 在PHP项目中创建一个OAuth2服务器文件,并进行基本配置。实现代码如下:
require_once DIR . '/vendor/autoload.php';
use LeagueOAuth2ServerAuthorizationServer;
use LeagueOAuth2ServerResourceServer;
use LeagueOAuth2ServerGrantClientCredentialsGrant ;
use LeagueOAuth2ServerGrantPasswordGrant;
use LeagueOAuth2ServerGrantAuthCodeGrant;
use LeagueOAuth2ServerGrantImplicitGrant;
use LeagueOAuth2ServerRepositories{AccessTokenRepositoryInterface,
AuthCodeRepositoryInterface, ClientRepositoryInterface, RefreshTokenRepositoryInterface, UserRepositoryInterface};
use LeagueOAuth2Server{ResourceServer, AuthorizationValidatorsBearerTokenValidator};
/ / Configure server
$server = new AuthorizationServer(
$clientRepository, $accessTokenRepository, $scopeRepository, $privateKey, $publicKey
);
// Configure authorization type
$server->enableGrantType(new ClientCredentialsGrant());
$server->enableGrantType(
new PasswordGrant( $userRepository, $refreshTokenRepository )
);
$server->enableGrantType(
new AuthCodeGrant( $authCodeRepository, $refreshTokenRepository, new DateInterval('PT10M') )
);
$server->enableGrantType(
new ImplicitGrant( new DateInterval('PT1H') )
);
//Configure the resource server
$resourceServer = new ResourceServer(
$accessTokenRepository, $publicKey
);
//Configure the BearerTokenValidator
$bearerTokenValidator = new BearerTokenValidator(
$accessTokenRepository, $publicKey
);
上述代码中的各个参数需要根据实际的业务需求进行具体配置。 3. 执行授权请求 在OAuth2服务器文件中,需要实现授权请求的处理逻辑。实现代码如下:
use PsrHttpMessageServerRequestInterface;
use PsrHttpMessageResponseInterface;
// Process authorization request
$server->respondToAccessTokenRequest($request , $response);
上述代码中的$request和$response分别为从HTTP传输层获取的请求参数和响应结果。需要根据实际业务需求进行具体实现。 4. 请求受保护的资源 完成授权后,客户端可以携带访问令牌请求受保护的资源。OAuth2.0服务器需要对访问令牌进行验证,并返回相应的结果。实现代码如下:
// Verify Bearer Token
try {
$bearerTokenValidator->validateAuthorization($request);
} catch (Exception $e) {
$response->getBody()->write($e->getMessage()); return $response->withHeader('Content-Type', 'text/plain')->withStatus(401);
}
// Process the access token in the request header
$accessToken = $request->getHeader('Authorization')[0];
$accessToken = substr($accessToken, strpos($accessToken, ' ' ) 1);
//Verify access token
try {
$resourceServer->validateAuthenticatedRequest($request); $response->getBody()->write('Access granted');
} catch (Exception $e) {
$errorMessage = $e->getMessage(); $response->getBody()->write($errorMessage); return $response->withHeader('Content-Type', 'text/plain')->withStatus(401);
}
5. 总结
The above is the detailed content of The best way to implement OAuth2.0 using PHP. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
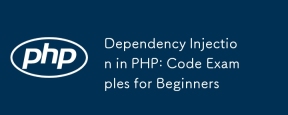
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
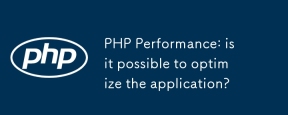
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
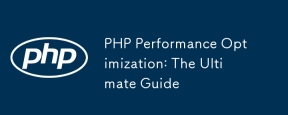
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
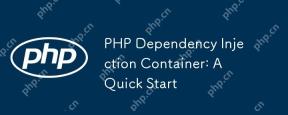
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
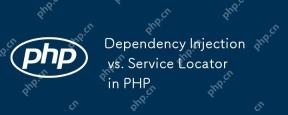
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
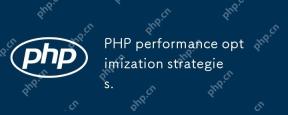
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
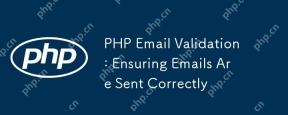
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
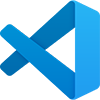
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
