When it comes to classifying large amounts of data, manually processing this data is a very time-consuming and difficult task. In this case, using a neural network for classification can do the job quickly and easily. Python is a good choice because it has many mature and easy-to-use neural network libraries. This article explains how to use neural networks for classification in Python.
- Neural Networks and Classification
Before explaining how to use neural networks for classification, we need to briefly understand the concept of neural networks. A neural network is a computational model that works by building a model based on relationships between large amounts of input and output data to predict certain properties of unknown data. This model performs very well on classification problems and can be used to classify different types of data such as pictures, emails, and voices.
Classification is one of the main applications of neural networks. The purpose of classification problems is to classify data into different categories. For example, in image recognition, neural networks can classify different images into different categories such as cats, dogs, or cars. In this case, the neural network takes images as input data and classification as output data. Classification is the process of dividing data into different categories, usually using supervised learning methods.
- Install Neural Network Library
There are many neural network libraries to choose from in Python, such as TensorFlow, Keras, PyTorch, etc. In this article, we will use TensorFlow, an open source artificial intelligence library developed by the Google brain team. TensorFlow is a very popular framework that is easy to learn and use, and it is used in a large number of machine learning projects.
If you have not installed TensorFlow, you can open a terminal or command prompt and enter the following command:
pip install tensorflow
After the installation is complete, you can Use the TensorFlow library.
- Data preparation
Data preparation is a critical step in the classification task. The data needs to be converted into a numerical format that can be understood by the neural network. Here, we will introduce a very popular dataset MNIST, which consists of digital images, each image represents a number. The MNIST dataset is available in TensorFlow, and you can load the data directly using the following command:
from tensorflow.keras.datasets import mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
This command loads the MNIST dataset into the variables x_train and y_train, which are used to train the neural network. Test data is loaded into variables x_test and y_test and is used to test the neural network. x_train and x_test contain the numeric image data, y_train and y_test contain the labels of the numeric images.
Next, let’s take a look at the dataset to learn more:
print('x_train shape:', x_train.shape)
print('y_train shape :', y_train.shape)
print('x_test shape:', x_test.shape)
print('y_test shape:', y_test.shape)
at In the output, you will see the following information:
x_train shape: (60000, 28, 28)
y_train shape: (60000,)
x_test shape: (10000 , 28, 28)
y_test shape: (10000,)
This shows that the training data set contains 60000 digital images, each image is 28 pixels x 28 pixels. The test dataset has 10,000 images.
- Neural Network Model
After preparing the data, you need to select a neural network model. We will choose a very simple neural network model consisting of two fully connected layers (Dense). The first fully connected layer contains 128 neurons, and the second fully connected layer contains 10 neurons. The code is as follows:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Flatten
model = Sequential()
model.add(Flatten(input_shape=(28, 28)))
model.add(Dense(128, activation='relu'))
model.add(Dense(10, activation='softmax'))
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
Here, we first created a Sequential model and then added a Flatten layer, which is used to flatten the 28x28 image data into a one-dimensional array. Next, we added a fully connected layer with 128 neurons and used ReLU as the activation function. Finally, we add another fully connected layer with 10 neurons and use the Softmax activation function to obtain a probability distribution for each number. The model is compiled using the adam optimizer and the sparse categorical cross-entropy loss function.
- Training model
We have prepared the data and model, now we need to use the training data to train the model. The following command can be used to train the model:
history = model.fit(x_train, y_train, epochs=10, validation_data=(x_test, y_test))
This code will use 10 epochs (epochs) to train the model and use the test set for validation. After training is complete, we can use the following code to evaluate the model:
test_loss, test_acc = model.evaluate(x_test, y_test)
print('Test accuracy:', test_acc)
In the output you will see the accuracy metrics on the test set.
- Prediction
After training and evaluating the model, we can use the model to predict unknown data. We can use the following code to predict the label of an image:
import numpy as np
image_index = 7777 # Starting from 0
img = x_test[image_index]
img = np.expand_dims(img, axis=0)
predictions = model.predict(img)
print(predictions)
print("Predicted label :", np.argmax(predictions))
In the output, we can see that the image is predicted to be the number 2.
- Conclusion
In this article, we introduced how to use neural networks for classification in Python. We used TensorFlow to build and train the neural network model, and the MNIST dataset for testing and prediction. You can use this model for different categories of image classification tasks and adjust the neural network layers in the model as needed. Classification using neural networks is a very effective method that can easily handle large amounts of data classification, allowing us to perform model development and classification tasks faster.
The above is the detailed content of How to use neural networks for classification in Python?. For more information, please follow other related articles on the PHP Chinese website!
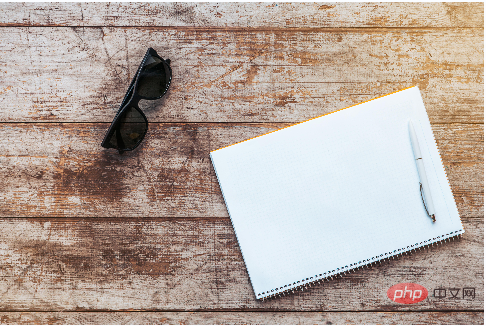
近年来,图神经网络(GNN)取得了快速、令人难以置信的进展。图神经网络又称为图深度学习、图表征学习(图表示学习)或几何深度学习,是机器学习特别是深度学习领域增长最快的研究课题。本次分享的题目为《GNN的基础、前沿和应用》,主要介绍由吴凌飞、崔鹏、裴健、赵亮几位学者牵头编撰的综合性书籍《图神经网络基础、前沿与应用》中的大致内容。一、图神经网络的介绍1、为什么要研究图?图是一种描述和建模复杂系统的通用语言。图本身并不复杂,它主要由边和结点构成。我们可以用结点表示任何我们想要建模的物体,可以用边表示两
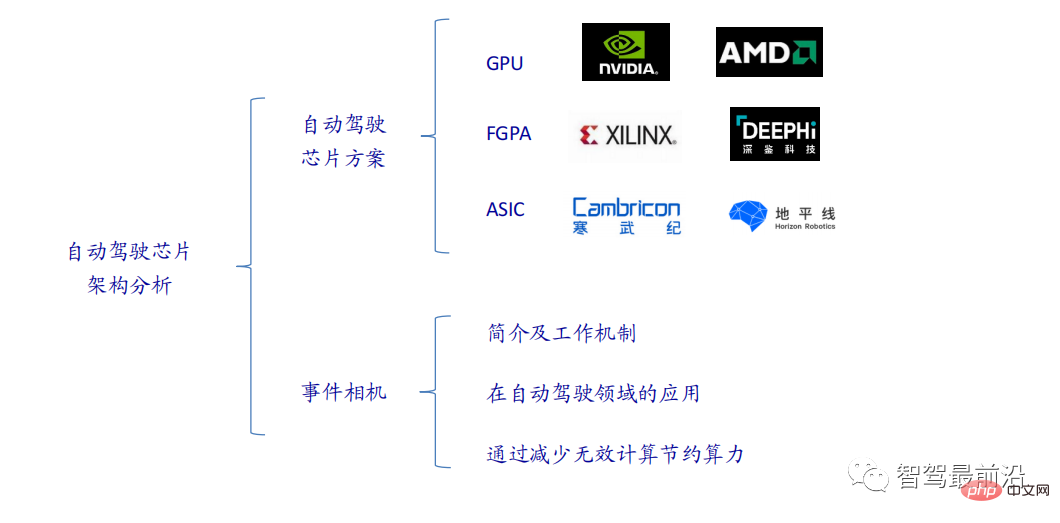
当前主流的AI芯片主要分为三类,GPU、FPGA、ASIC。GPU、FPGA均是前期较为成熟的芯片架构,属于通用型芯片。ASIC属于为AI特定场景定制的芯片。行业内已经确认CPU不适用于AI计算,但是在AI应用领域也是必不可少。 GPU方案GPU与CPU的架构对比CPU遵循的是冯·诺依曼架构,其核心是存储程序/数据、串行顺序执行。因此CPU的架构中需要大量的空间去放置存储单元(Cache)和控制单元(Control),相比之下计算单元(ALU)只占据了很小的一部分,所以CPU在进行大规模并行计算
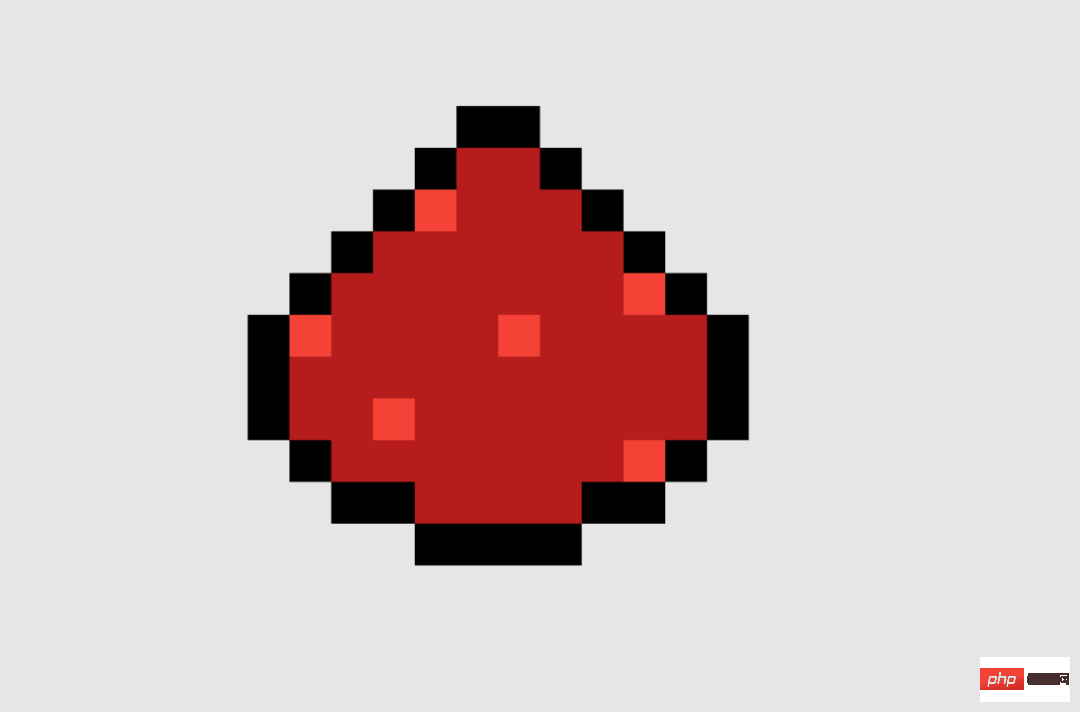
在我的世界(Minecraft)中,红石是一种非常重要的物品。它是游戏中的一种独特材料,开关、红石火把和红石块等能对导线或物体提供类似电流的能量。红石电路可以为你建造用于控制或激活其他机械的结构,其本身既可以被设计为用于响应玩家的手动激活,也可以反复输出信号或者响应非玩家引发的变化,如生物移动、物品掉落、植物生长、日夜更替等等。因此,在我的世界中,红石能够控制的机械类别极其多,小到简单机械如自动门、光开关和频闪电源,大到占地巨大的电梯、自动农场、小游戏平台甚至游戏内建的计算机。近日,B站UP主@
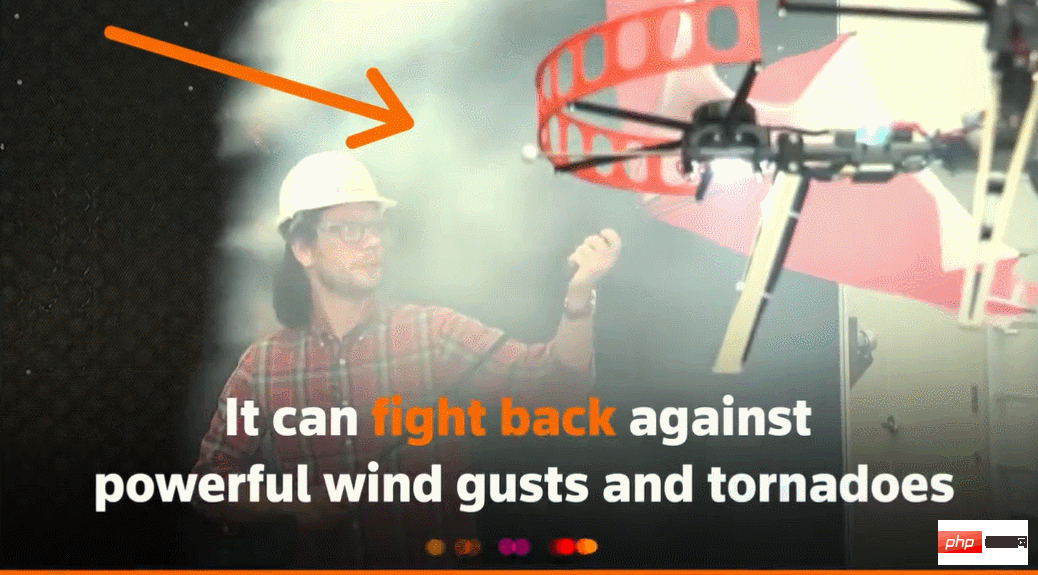
当风大到可以把伞吹坏的程度,无人机却稳稳当当,就像这样:御风飞行是空中飞行的一部分,从大的层面来讲,当飞行员驾驶飞机着陆时,风速可能会给他们带来挑战;从小的层面来讲,阵风也会影响无人机的飞行。目前来看,无人机要么在受控条件下飞行,无风;要么由人类使用遥控器操作。无人机被研究者控制在开阔的天空中编队飞行,但这些飞行通常是在理想的条件和环境下进行的。然而,要想让无人机自主执行必要但日常的任务,例如运送包裹,无人机必须能够实时适应风况。为了让无人机在风中飞行时具有更好的机动性,来自加州理工学院的一组工
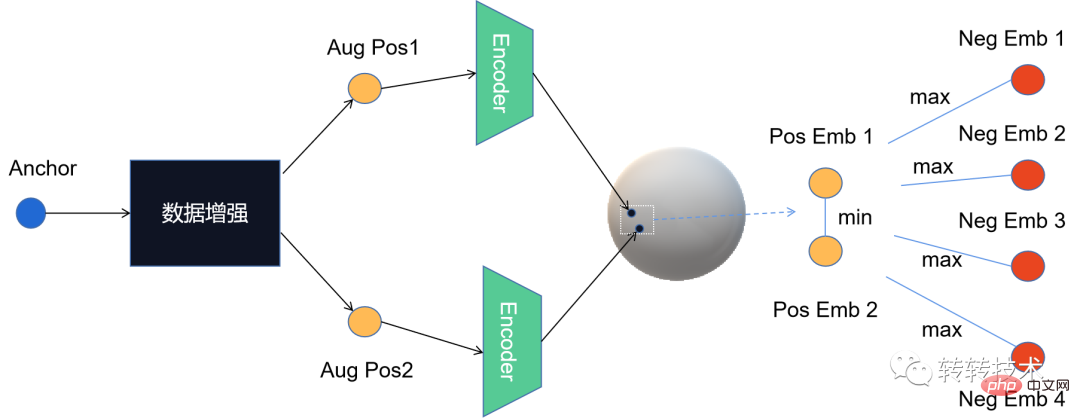
1 什么是对比学习1.1 对比学习的定义1.2 对比学习的原理1.3 经典对比学习算法系列2 对比学习的应用3 对比学习在转转的实践3.1 CL在推荐召回的实践3.2 CL在转转的未来规划1 什么是对比学习1.1 对比学习的定义对比学习(Contrastive Learning, CL)是近年来 AI 领域的热门研究方向,吸引了众多研究学者的关注,其所属的自监督学习方式,更是在 ICLR 2020 被 Bengio 和 LeCun 等大佬点名称为 AI 的未来,后陆续登陆 NIPS, ACL,
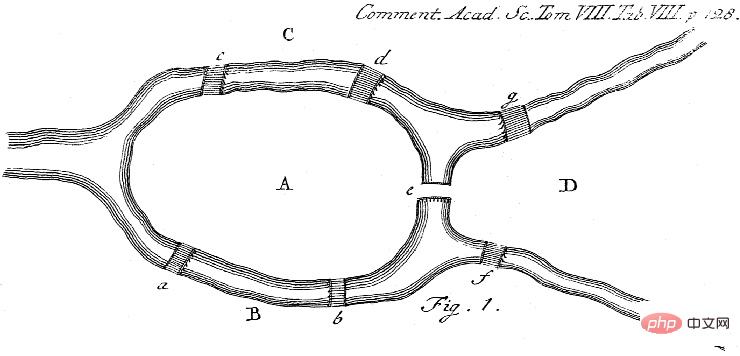
本文由Cristian Bodnar 和Fabrizio Frasca 合著,以 C. Bodnar 、F. Frasca 等人发表于2021 ICML《Weisfeiler and Lehman Go Topological: 信息传递简单网络》和2021 NeurIPS 《Weisfeiler and Lehman Go Cellular: CW 网络》论文为参考。本文仅是通过微分几何学和代数拓扑学的视角讨论图神经网络系列的部分内容。从计算机网络到大型强子对撞机中的粒子相互作用,图可以用来模

OTO 是业内首个自动化、一站式、用户友好且通用的神经网络训练与结构压缩框架。 在人工智能时代,如何部署和维护神经网络是产品化的关键问题考虑到节省运算成本,同时尽可能小地损失模型性能,压缩神经网络成为了 DNN 产品化的关键之一。DNN 压缩通常来说有三种方式,剪枝,知识蒸馏和量化。剪枝旨在识别并去除冗余结构,给 DNN 瘦身的同时尽可能地保持模型性能,是最为通用且有效的压缩方法。三种方法通常来讲可以相辅相成,共同作用来达到最佳的压缩效果。然而现存的剪枝方法大都只针对特定模型,特定任务,且需要很
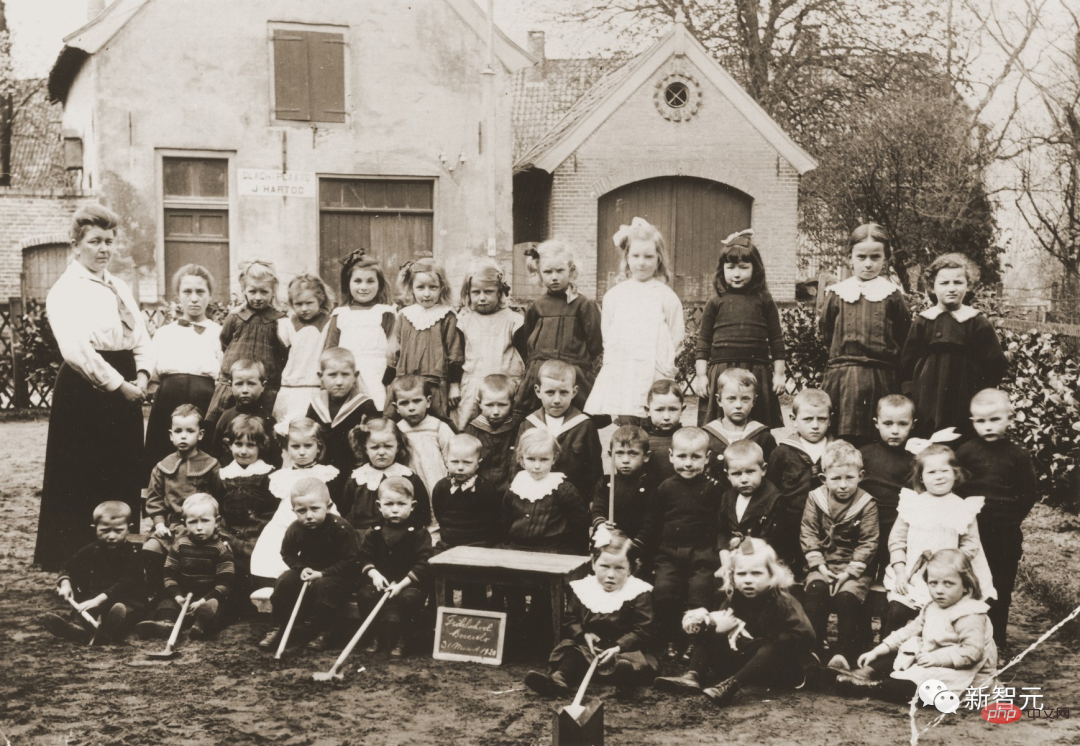
AI面部识别领域又开辟新业务了?这次,是鉴别二战时期老照片里的人脸图像。近日,来自谷歌的一名软件工程师Daniel Patt 研发了一项名为N2N(Numbers to Names)的 AI人脸识别技术,它可识别二战前欧洲和大屠杀时期的照片,并将他们与现代的人们联系起来。用AI寻找失散多年的亲人2016年,帕特在参观华沙波兰裔犹太人纪念馆时,萌生了一个想法。这一张张陌生的脸庞,会不会与自己存在血缘的联系?他的祖父母/外祖父母中有三位是来自波兰的大屠杀幸存者,他想帮助祖母找到被纳粹杀害的家人的照


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
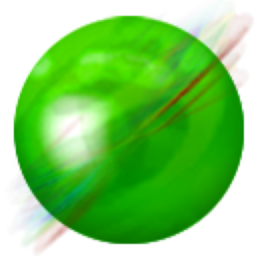
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
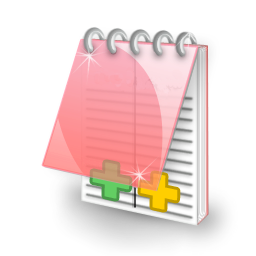
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
