How to custom write unit tests in Kajona framework?
Kajona is a popular PHP framework that allows developers to quickly build scalable web applications. In the development process of Kajona, unit testing is a crucial link. Unit testing ensures the quality and reliability of your code and reduces bugs and errors. However, unit tests in the Kajona framework require certain skills and experience to write.
The following is a guide on how to custom write unit tests in the Kajona framework to help you better understand the testing process and principles in the Kajona framework.
1. What is unit testing?
In software development, unit testing is a method of testing software components (such as functions, modules, classes). It can detect possible errors in software and help software developers eliminate bugs and improve software quality.
Unit testing usually adopts a code-based testing approach, where developers write test scripts and run them to ensure that each component works properly. Unit testing can also help developers understand the behavior and logic of the code, as well as ensure that the code meets specified design requirements and business processes.
2. Unit testing in the Kajona framework
The Kajona framework has provided developers with a set of predefined test classes that can test functions in the framework. Developers can use these test classes directly or write their own unit tests to test specific functionality.
Test classes in the Kajona framework are usually stored in the "/tests" directory. Each test class usually has a corresponding source file, which is stored in the "/src" directory. The name of the test class usually ends with "Test", such as HelloWorldTest.php.
Unit testing in the Kajona framework requires the use of the PHPUnit testing framework, which provides common test methods such as assertEquals(), assertArrayHasKey(), and assertNotEmpty().
The following is an example of unit testing in the Kajona framework:
<?php class HelloWorldTest extends KajonaSystemTestsTestbase{ public function testSayHello(){ $hello = new HelloWorld(); $this->assertEquals($hello->sayHello(), "Hello, World!"); } } ?>
In this test class, we test a method in HelloWorld.php and use the assertEquals() method to check HelloWorld's sayHello () method returns "Hello, World!".
3. Write custom unit tests
If you want to custom-write unit tests to test functions in the Kajona framework, you need to use the following steps:
1. Determine which features should be tested. First, you need to select the component, class, or method you want to test. Make sure you understand what you are testing and what the expected results are.
2. Write test class. Next, you need to write a test class and add one or more test methods in it. The test class should extend the KajonaSystemTestsTestbase class and implement the setUp() method. The setUp() method should be run before each test method and initialize the necessary test data.
3. Write test methods. In the test class you need to write the method you want to test. Test methods should use the various methods provided by the PHPUnit testing framework to test the functionality and behavior of the code.
4. Run the test. Finally, you need to run the test class you wrote and see the test results. You can use PHPUnit to run your test class and see if the test passes.
The following is a custom unit test example that demonstrates how to test a class in the Kajona framework:
<?php class MyCustomClassTest extends KajonaSystemTestsTestbase{ private $customClass; public function setUp(){ parent::setUp(); $this->customClass = new MyCustomClass(); } public function testCustomMethod(){ $result = $this->customClass->customMethod(); $this->assertEquals($result, "Hello, Kajona!"); } } ?>
In this test class, we test a class named customMethod in the MyCustomClass class () method and check whether its output is what we expected.
Summary
Unit testing is a necessary step in any software development project to ensure the quality and reliability of the software. In the Kajona framework, unit testing is a very important link. If you want to custom write unit tests, the Kajona framework already provides you with a set of predefined test classes and provides methods to use the PHPUnit testing framework. I hope this article was helpful and allowed you to better understand and apply unit testing techniques.
The above is the detailed content of How to custom write unit tests in Kajona framework?. For more information, please follow other related articles on the PHP Chinese website!
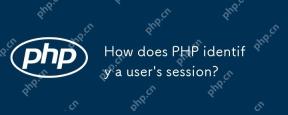
PHPidentifiesauser'ssessionusingsessioncookiesandsessionIDs.1)Whensession_start()iscalled,PHPgeneratesauniquesessionIDstoredinacookienamedPHPSESSIDontheuser'sbrowser.2)ThisIDallowsPHPtoretrievesessiondatafromtheserver.
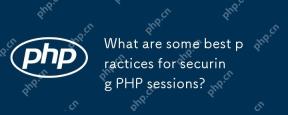
The security of PHP sessions can be achieved through the following measures: 1. Use session_regenerate_id() to regenerate the session ID when the user logs in or is an important operation. 2. Encrypt the transmission session ID through the HTTPS protocol. 3. Use session_save_path() to specify the secure directory to store session data and set permissions correctly.
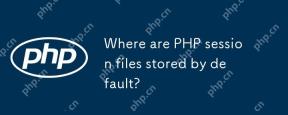
PHPsessionfilesarestoredinthedirectoryspecifiedbysession.save_path,typically/tmponUnix-likesystemsorC:\Windows\TemponWindows.Tocustomizethis:1)Usesession_save_path()tosetacustomdirectory,ensuringit'swritable;2)Verifythecustomdirectoryexistsandiswrita
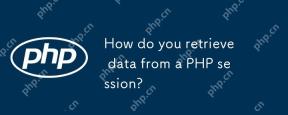
ToretrievedatafromaPHPsession,startthesessionwithsession_start()andaccessvariablesinthe$_SESSIONarray.Forexample:1)Startthesession:session_start().2)Retrievedata:$username=$_SESSION['username'];echo"Welcome,".$username;.Sessionsareserver-si
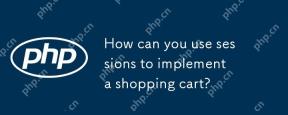
The steps to build an efficient shopping cart system using sessions include: 1) Understand the definition and function of the session. The session is a server-side storage mechanism used to maintain user status across requests; 2) Implement basic session management, such as adding products to the shopping cart; 3) Expand to advanced usage, supporting product quantity management and deletion; 4) Optimize performance and security, by persisting session data and using secure session identifiers.
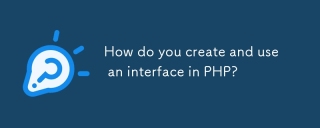
The article explains how to create, implement, and use interfaces in PHP, focusing on their benefits for code organization and maintainability.
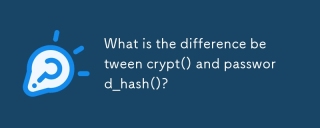
The article discusses the differences between crypt() and password_hash() in PHP for password hashing, focusing on their implementation, security, and suitability for modern web applications.
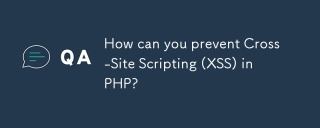
Article discusses preventing Cross-Site Scripting (XSS) in PHP through input validation, output encoding, and using tools like OWASP ESAPI and HTML Purifier.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
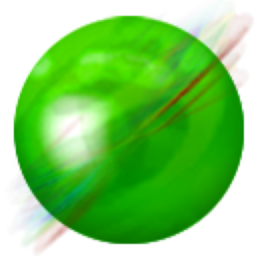
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
