1. Create a database
First, we need to create a database for adding pages to our articles. Open phpMyAdmin or other database management tools, create a database named "blog", and create a data table named "article" in it. The fields of the data table include: id (ID of the article), title (title of the article), content (content of the article), create_time (creation time of the article), update_time (update time of the article).
2. Create models, controllers and views
Next, we need to create models, controllers and views, as well as corresponding operation methods. We need to create an Article model first so that we can obtain article data at any time.
In ThinkPHP, creating a model is very simple, just create an Article.php file in the common directory under the application directory and add the following code to it:
<?php namespace app\common\model; use think\Model; class Article extends Model { //表名 protected $table = 'article'; }
Next, We need to create a controller that handles the adding of articles and renders the article adding page.
In ThinkPHP, creating a controller is also very simple. Just create an Article.php file in the admin directory under the application directory and add the following code to it:
<?php namespace app\admin\controller; use think\Controller; use app\common\model\Article; class Article extends Controller { public function add() { if(request()->isPost()){ $data = input('post.'); $data['create_time'] = time(); $data['update_time'] = time(); $article = new Article(); if($article->allowField(true)->save($data)){ $this->success('添加成功!'); } $this->error('添加失败!'); } return view(); } }
The The add method of the controller is used to render the article addition page and handle the operation of adding articles. When saving an article, the title, content, creation time, and update time need to be stored in a database table.
Finally, we need to create a view that is used to display the article addition interface. Create an article/add.html file in the admin directory under the application directory, and add the following code to it:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>添加文章</title> </head> <body> <form action="" method="post"> <div> <label for="title">标题:</label> <input type="text" name="title" id="title"> </div> <div> <label for="content">内容:</label> <textarea name="content" id="content" cols="30" rows="10"></textarea> </div> <div> <input type="submit" value="添加"> <input type="reset" value="重置"> </div> </form> </body> </html>
3. Test the article adding interface
After completing the above steps, we can already test our article adding interface. Enter "http://your_domain/admin/article/add" in your browser to access the article add page. To save an article to the database, simply fill in the page with the article title and content and click the "Add" button.
The above is the detailed content of How to use ThinkPHP to implement article adding interface. For more information, please follow other related articles on the PHP Chinese website!
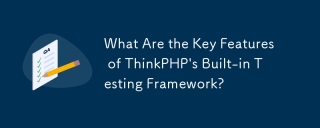
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
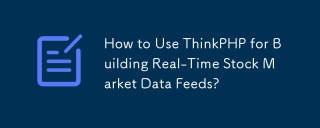
Article discusses using ThinkPHP for real-time stock market data feeds, focusing on setup, data accuracy, optimization, and security measures.

The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
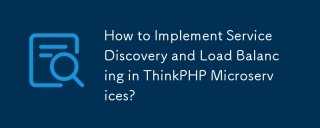
The article discusses implementing service discovery and load balancing in ThinkPHP microservices, focusing on setup, best practices, integration methods, and recommended tools.[159 characters]
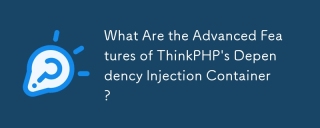
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
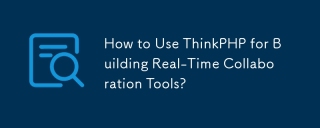
The article discusses using ThinkPHP to build real-time collaboration tools, focusing on setup, WebSocket integration, and security best practices.
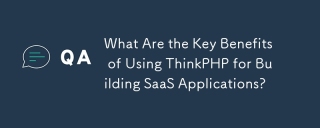
ThinkPHP benefits SaaS apps with its lightweight design, MVC architecture, and extensibility. It enhances scalability, speeds development, and improves security through various features.
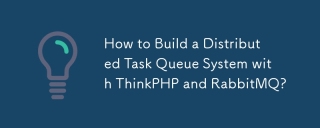
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
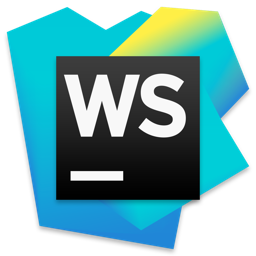
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor