With the rapid development of the Internet, more and more websites and applications are developed, and the amount of data becomes larger and larger, so data processing and management become more and more important. Among them, paging is a very common data query method, which can easily display large amounts of data and provide users with a better user experience. In development, we generally use MySQL database to store data. This article introduces how to use Node.js to encapsulate MySQL to implement paging query function.
- Create database table
First, we need to create a table in MySQL to store the data we need. This article takes the "users" table as an example, which contains three fields: id, name, and age. The specific table structure and data are as follows:
CREATE TABLE users ( id INT(11) NOT NULL AUTO_INCREMENT, name VARCHAR(20) NOT NULL, age INT(11) NOT NULL, PRIMARY KEY (id) ); INSERT INTO users (name, age) VALUES ('小明', 18); INSERT INTO users (name, age) VALUES ('小红', 20); INSERT INTO users (name, age) VALUES ('小华', 22); INSERT INTO users (name, age) VALUES ('小张', 25); INSERT INTO users (name, age) VALUES ('小李', 28); INSERT INTO users (name, age) VALUES ('小刘', 30);
- Install MySQL and mysql modules
Next, we need to install the MySQL database and the corresponding mysql module. It can be installed through the following command:
npm install mysql
- Paging query implementation
Before using the mysql module, we need to connect to the MySQL database through the following code:
const mysql = require('mysql'); const connection = mysql.createConnection({ host: 'localhost', user: 'root', password: 'password', database: 'test' }); connection.connect();
Here, we set the connected database name to "test", you need to change it according to your actual situation.
Next, we encapsulate a paging query function to query the specified number of pages of data. The function name is "queryByPage", and the parameters passed in are:
- tableName: the name of the data table to be queried
- pageNum: the number of pages to be queried
- pageSize: The number of data displayed on each page
- callback: callback function, used to receive query results
The function implementation code is as follows:
function queryByPage(tableName, pageNum, pageSize, callback) { const start = (pageNum - 1) * pageSize; const sql = `SELECT * FROM ${tableName} ORDER BY id DESC LIMIT ${start}, ${pageSize}`; connection.query(sql, (err, results) => { if (err) { return callback(err, null); } const countSql = `SELECT count(*) as count FROM ${tableName}`; connection.query(countSql, (err, count) => { if (err) { return callback(err, null); } const totalCount = count[0].count; const totalPage = Math.ceil(totalCount / pageSize); return callback(null, { pageNum, pageSize, totalCount, totalPage, data: results }); }); }); }
The above code implements the following Several functions:
- Calculate the starting position of query data based on the number of pages passed in and the number of data displayed on each page
- Execute MySQL query statements to implement paging queries and obtain Query results
- Execute the MySQL query statement and obtain the total amount of data
- Calculate the total number of pages based on the total amount of data and the number of data displayed on each page
- Page the query results and paging The information is encapsulated into an object for callback
- Call the paging query function
Finally, we can call the encapsulated paging query function in the Node.js application , and display the query results as needed.
queryByPage('users', 1, 2, (err, result) => { if (err) { console.error(err.message); return; } console.log(result); });
The above code will query the first page of data in the "users" table, display 2 records on each page, and output the query results to the console.
- Summary
This article introduces how to use Node.js to encapsulate MySQL to implement paging query function. By creating database tables, installing the mysql module and encapsulating query functions, we can easily query the data of a specified number of pages and display it in the application. Of course, in actual applications, we also need to improve the code according to the actual situation, and perform error handling and exception handling.
The above is the detailed content of nodejs encapsulates mysql to implement paging. For more information, please follow other related articles on the PHP Chinese website!

HTML and React can be seamlessly integrated through JSX to build an efficient user interface. 1) Embed HTML elements using JSX, 2) Optimize rendering performance using virtual DOM, 3) Manage and render HTML structures through componentization. This integration method is not only intuitive, but also improves application performance.

React efficiently renders data through state and props, and handles user events through the synthesis event system. 1) Use useState to manage state, such as the counter example. 2) Event processing is implemented by adding functions in JSX, such as button clicks. 3) The key attribute is required to render the list, such as the TodoList component. 4) For form processing, useState and e.preventDefault(), such as Form components.

React interacts with the server through HTTP requests to obtain, send, update and delete data. 1) User operation triggers events, 2) Initiate HTTP requests, 3) Process server responses, 4) Update component status and re-render.

React is a JavaScript library for building user interfaces that improves efficiency through component development and virtual DOM. 1. Components and JSX: Use JSX syntax to define components to enhance code intuitiveness and quality. 2. Virtual DOM and Rendering: Optimize rendering performance through virtual DOM and diff algorithms. 3. State management and Hooks: Hooks such as useState and useEffect simplify state management and side effects handling. 4. Example of usage: From basic forms to advanced global state management, use the ContextAPI. 5. Common errors and debugging: Avoid improper state management and component update problems, and use ReactDevTools to debug. 6. Performance optimization and optimality

React can be embedded in HTML to enhance or completely rewrite traditional HTML pages. 1) The basic steps to using React include adding a root div in HTML and rendering the React component via ReactDOM.render(). 2) More advanced applications include using useState to manage state and implement complex UI interactions such as counters and to-do lists. 3) Optimization and best practices include code segmentation, lazy loading and using React.memo and useMemo to improve performance. Through these methods, developers can leverage the power of React to build dynamic and responsive user interfaces.

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.

React is a JavaScript library for building user interfaces. Its core idea is to build UI through componentization. 1. Components are the basic unit of React, encapsulating UI logic and styles. 2. Virtual DOM and state management are the key to component work, and state is updated through setState. 3. The life cycle includes three stages: mount, update and uninstall. The performance can be optimized using reasonably. 4. Use useState and ContextAPI to manage state, improve component reusability and global state management. 5. Common errors include improper status updates and performance issues, which can be debugged through ReactDevTools. 6. Performance optimization suggestions include using memo, avoiding unnecessary re-rendering, and using us


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
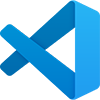
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft