Overview
As Node.js is more and more widely used in web development, many developers will encounter the problem of reading garbled files. Although this problem is not unsolvable, it will consume a lot of time and energy. This article will introduce how to solve the problem of garbled files read by Node.js.
Problem Analysis
In Node.js, we can use the fs
module to read local files. When reading a file, we need to specify the encoding method of the file, otherwise Node.js will read the file in binary mode by default, resulting in garbled characters when reading the file.
The following is an example code for reading a Chinese file:
const fs = require('fs'); fs.readFile('test.txt', 'utf8', function (err, data) { if (err) { console.error(err); } else { console.log(data); } });
In this example, we read test by calling
fs.readFile() .txt
file. In the second parameter, we specify the encoding method of the file as utf8
. However, even if we specify the encoding method of the file, the read file will still be garbled.
Solution
Node.js reads garbled files. There are several solutions:
- Confirm the file encoding method
Before determining that the second parameter of fs.readFile()
specifies the correct encoding method, we need to confirm whether the encoding method of the file is correct. In Windows, you can view the file encoding by right-clicking the file, selecting Properties, and then selecting the General tab.
If the file encoding method is not utf8
, we need to specify the correct encoding method when calling fs.readFile()
. Common file encoding methods are:
- UTF-8: used to support various languages and special characters.
- GB2312: Applicable to Simplified Chinese.
- BIG5: Applicable to Traditional Chinese.
- Use the iconv-lite module
If you confirm that the file encoding method is correct but garbled characters still appear, we can use the iconv-lite
module Perform encoding conversion.
iconv-lite
is a Node.js module specially used for encoding conversion. The content of the read binary file can be read by calling its decode()
method. Perform encoding conversion.
The code using the iconv-lite
module is as follows:
const fs = require('fs'); const iconv = require('iconv-lite'); fs.readFile('test.txt', function (err, data) { if (err) { console.error(err); } else { const content = iconv.decode(data, 'gbk'); // 将读取出的二进制文件解码为GBK console.log(content); } });
In this example, we read the The extracted binary file is decoded into GBK encoding. In order to decode correctly, we need to specify the correct encoding.
- Another solution is to use the
object provided by Node.js for encoding conversion. When reading a file, we can specify the file encoding as null
, which will cause fs.readFile()
to return a Buffer
object. Then we can use the decode()
method in the iconv-lite
module to convert the Buffer
object into text with the specified encoding method. The code for converting encoding using
is as follows: <pre class='brush:php;toolbar:false;'>const fs = require('fs');
const iconv = require('iconv-lite');
fs.readFile('test.txt', function (err, data) {
if (err) {
console.error(err);
} else {
const buffer = Buffer.from(data);
const content = iconv.decode(buffer, 'gbk'); // 将Buffer对象解码为GBK
console.log(content);
}
});</pre>
In this example, we will read by calling the
method Convert the outgoing content to a Buffer
object, and then use the iconv.decode()
method to convert it to GBK-encoded text. Summary
The problem of Node.js reading garbled files requires choosing a solution based on the actual situation. If you confirm that the file encoding method is correct but garbled characters still appear, we can try to use the
iconv-lite module or Buffer
object for encoding conversion. When using the fs
module to read files, reasonably specifying the file encoding method is the basic method to avoid garbled characters.
The above is the detailed content of Nodejs reads garbled files. For more information, please follow other related articles on the PHP Chinese website!
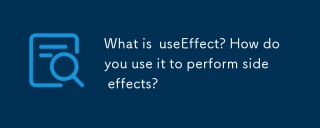
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
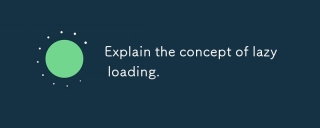
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
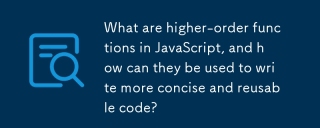
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
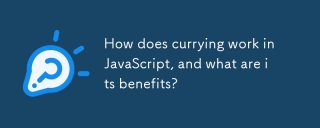
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
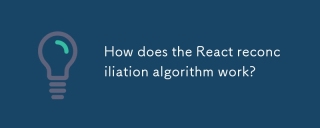
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
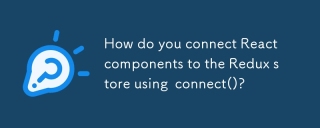
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
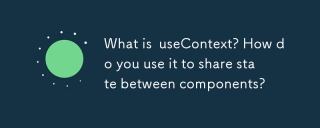
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
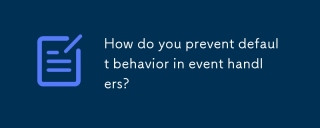
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
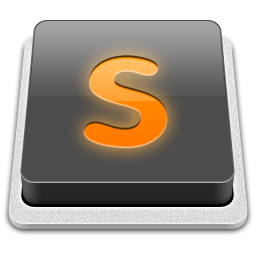
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.