


How do you connect React components to the Redux store using connect()?
To connect React components to the Redux store using the connect()
function from the react-redux
library, you follow these steps:
-
Import
connect
: First, you need to import theconnect
function fromreact-redux
:import { connect } from 'react-redux';
-
Define
mapStateToProps
andmapDispatchToProps
: These functions are optional but commonly used.mapStateToProps
takes the store's state and returns an object of props for your component.mapDispatchToProps
takes thedispatch
function and returns an object of action creators bound todispatch
.const mapStateToProps = (state) => { return { // Example props todos: state.todos, }; }; const mapDispatchToProps = (dispatch) => { return { // Example action addTodo: (text) => dispatch(addTodo(text)), }; };
-
Use
connect
to wrap your component: You useconnect
to wrap your component, passingmapStateToProps
andmapDispatchToProps
as its arguments. This creates a new component that is connected to the Redux store.const ConnectedComponent = connect( mapStateToProps, mapDispatchToProps )(YourComponent);
-
Export the Connected Component: Finally, you can export this new connected component to be used in other parts of your application.
export default ConnectedComponent;
By following these steps, your React component will be able to receive data from the Redux store and dispatch actions to the store, enabling a unidirectional data flow typical in Redux applications.
What are the benefits of using connect() to link React components with the Redux store?
Using connect()
to link React components with the Redux store provides several key benefits:
-
Declarative Data Fetching: With
connect()
, you can declaratively specify what data your component needs from the store, simplifying the process of managing state across your application. - Separation of Concerns: It helps maintain a clean separation between your UI components and the business logic contained in the Redux store, promoting better code organization and reusability.
-
Performance Optimization:
connect()
implementsshouldComponentUpdate
under the hood, which can help optimize the performance of your application by preventing unnecessary re-renders when the state changes but the relevant props have not. -
Simplified State Management: By centralizing the state in a Redux store,
connect()
simplifies how state is passed through components, reducing prop drilling and making it easier to manage complex state interactions. -
Easier Testing: Components connected with
connect()
are easier to test because you can mock the Redux store and test the component in isolation, with predefined states and actions.
Can you explain how mapStateToProps and mapDispatchToProps work within the connect() function?
mapStateToProps
and mapDispatchToProps
are crucial functions within the connect()
function that serve specific purposes:
-
mapStateToProps: This function takes the entire Redux store state as its first argument and returns an object. The keys in this object become the props that are passed to your component. Essentially, it maps parts of the Redux state to the props of your component, allowing your component to subscribe to state changes. For example:
const mapStateToProps = (state) => { return { todos: state.todos, }; };
In this example, whenever the state in the Redux store changes,
mapStateToProps
runs, and if thetodos
state has changed, it will pass this newtodos
data as a prop to the connected component. -
mapDispatchToProps: This function takes the
dispatch
function from the Redux store as its argument and returns an object with action creators as values. These action creators, when called, dispatch actions to the Redux store. The keys of the object become the props for your component. For instance:const mapDispatchToProps = (dispatch) => { return { addTodo: (text) => dispatch(addTodo(text)), }; };
Here,
addTodo
becomes a prop on the connected component, which can be called asthis.props.addTodo(text)
within the component, dispatching theaddTodo
action to the store.
How does the performance of React applications change when using connect() with Redux?
Using connect()
with Redux can impact the performance of React applications in both positive and negative ways:
-
Positive Impacts:
-
Optimized Re-renders: The
connect()
function implementsshouldComponentUpdate
automatically. This means that connected components only re-render when the relevant parts of the state (as determined bymapStateToProps
) have actually changed, potentially improving performance by reducing unnecessary re-renders. -
Reduced Prop Drilling: By centralizing state management, Redux with
connect()
can simplify the data flow through your application, reducing the need to pass props deeply through the component tree and thereby possibly improving component rendering efficiency.
-
Optimized Re-renders: The
-
Potential Negative Impacts:
- Overhead of Store Subscriptions: Every time the state in the Redux store changes, all connected components must check if their props have changed. In large applications with many connected components, this can lead to a performance hit, especially if many components are subscribing to the same state slice.
-
Complexity in Large Applications: As applications grow, managing the complexity of state slices and ensuring that
mapStateToProps
functions are optimized and do not inadvertently trigger re-renders can become challenging, potentially affecting performance if not handled correctly.
To mitigate these impacts, developers can use techniques like memoization with reselect
for mapStateToProps
, or consider using React.memo
for connected components to further control re-renders based on prop changes. Additionally, careful design of the state structure and use of the store can help manage performance effectively in larger applications.
The above is the detailed content of How do you connect React components to the Redux store using connect()?. For more information, please follow other related articles on the PHP Chinese website!

Classselectorsareversatileandreusable,whileidselectorsareuniqueandspecific.1)Useclassselectors(denotedby.)forstylingmultipleelementswithsharedcharacteristics.2)Useidselectors(denotedby#)forstylinguniqueelementsonapage.Classselectorsoffermoreflexibili

IDsareuniqueidentifiersforsingleelements,whileclassesstylemultipleelements.1)UseIDsforuniqueelementsandJavaScripthooks.2)Useclassesforreusable,flexiblestylingacrossmultipleelements.

Using a class-only selector can improve code reusability and maintainability, but requires managing class names and priorities. 1. Improve reusability and flexibility, 2. Combining multiple classes to create complex styles, 3. It may lead to lengthy class names and priorities, 4. The performance impact is small, 5. Follow best practices such as concise naming and usage conventions.

ID and class selectors are used in CSS for unique and multi-element style settings respectively. 1. The ID selector (#) is suitable for a single element, such as a specific navigation menu. 2.Class selector (.) is used for multiple elements, such as unified button style. IDs should be used with caution, avoid excessive specificity, and prioritize class for improved style reusability and flexibility.

Key goals and advantages of HTML5 include: 1) Enhanced web semantic structure, 2) Improved multimedia support, and 3) Promoting cross-platform compatibility. These goals lead to better accessibility, richer user experience and more efficient development processes.

The goal of HTML5 is to simplify the development process, improve user experience, and ensure the dynamic and accessible network. 1) Simplify the development of multimedia content by natively supporting audio and video elements; 2) Introduce semantic elements such as, etc. to improve content structure and SEO friendliness; 3) Enhance offline functions through application cache; 4) Use elements to improve page interactivity; 5) Optimize mobile compatibility and support responsive design; 6) Improve form functions and simplify verification process; 7) Provide performance optimization tools such as async and defer attributes.

HTML5transformswebdevelopmentbyintroducingsemanticelements,multimediacapabilities,powerfulAPIs,andperformanceoptimizationtools.1)Semanticelementslike,,,andenhanceSEOandaccessibility.2)Multimediaelementsandallowdirectembeddingwithoutplugins,improvingu

TherealdifferencebetweenusinganIDversusaclassinCSSisthatIDsareuniqueandhavehigherspecificity,whileclassesarereusableandbetterforstylingmultipleelements.UseIDsforJavaScripthooksoruniqueelements,anduseclassesforstylingpurposes,especiallywhenapplyingsty


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment
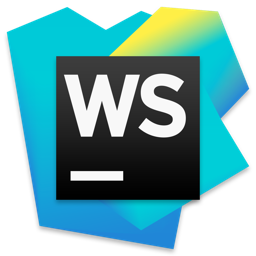
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
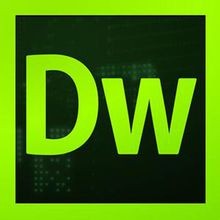
Dreamweaver CS6
Visual web development tools
