


With the advent of the digital age, computer programs play an increasingly important role in people's daily lives. However, since program codes can contain various errors, error handling has also become crucial. As a widely used scripting language, PHP introduced a new exception handling mechanism in its recently released version 7.0. This article introduces this new mechanism and discusses how to use it to handle exceptions.
Exception handling mechanism is an error handling mechanism in computer programming. This mechanism is used to catch errors in the program and handle them accordingly when an error occurs. In PHP, the exception mechanism was first introduced in version 5.0, however, the way it was implemented was not intuitive or easy to read. In version 7.0, PHP introduced a new exception handling mechanism with a more concise and intuitive syntax, which also makes exception handling easier to implement and maintain.
The custom exception handling mechanism in PHP7.0 has the following implementation methods:
- Create an exception class
In PHP, it is recommended Use exception classes to handle exceptions that occur in your program. This exception class must implement PHP's built-in exception interface Throwable. In the constructor, you can specify the exception message and call the parent class's constructor. The following code demonstrates how to create an exception class:
<? php class MyCustomException extends Exception { public function __construct($message, $code = 0, Throwable $previous = null) { parent::__construct($message, $code, $previous); } } ?>
- Throw an exception
To throw an exception, you need to use PHP's built-in throw keyword. In order to throw a custom exception, just create an instance of the exception class and pass it as a parameter to the throw statement. The following code demonstrates how to throw a custom exception:
<? php throw new MyCustomException("An error has occurred!", 1); ?>
When the program executes the throw statement, the program will stop execution, and control will be passed to the code block that catches the exception.
- Catch exceptions
To catch an exception, you need to use a try-catch block. Code that may cause an exception is executed in the try block. If an exception is thrown, the program will jump to the catch block. In the catch block, use parameter $e to specify the exception instance to be caught, and handle it accordingly in the catch block. The following code demonstrates how to use try-catch blocks to catch exceptions:
<?php try { // Code that may throw an exception } catch (MyCustomException $e) { // Handle the caught exception } ?>
If no exception is thrown in the try block, the program will continue to execute the code after the catch block. If the exception is not caught, the program will throw a fatal error and stop execution.
- Multiple catch blocks
A try block can have multiple catch blocks to catch different exception types. The order of multiple catch blocks is important. Subclass exceptions must be placed before parent class exceptions, otherwise unpredictable results will occur. The following code demonstrates how to use multiple catch blocks to catch different exception types:
<?php try { // Code that may throw an exception } catch (MyCustomException $e) { // Handle MyCustomException } catch (Exception $e) { // Handle all other exceptions } ?>
In the above example, if MyCustomException is thrown, then the first catch block handles the exception. If another type of exception is thrown, the second catch block handles the exception.
- Finally block
The finally block is executed after the try-catch block and will be executed regardless of whether the exception is caught or not. The finally block is usually used to release system resources or perform some general cleanup work. The following code demonstrates how to use the finally block:
<?php try { // Code that may throw an exception } catch (MyCustomException $e) { // Handle MyCustomException } finally { // This code always runs } ?>
In summary, PHP7.0 provides a more convenient and intuitive exception handling mechanism. The above five methods can be used in combination, which also makes it easier to implement a complete exception handling mechanism. Especially in large projects, using good exception handling mechanisms can improve the readability, reliability, and maintainability of the code.
The above is the detailed content of What are the implementation methods of custom exception handling in PHP7.0?. For more information, please follow other related articles on the PHP Chinese website!
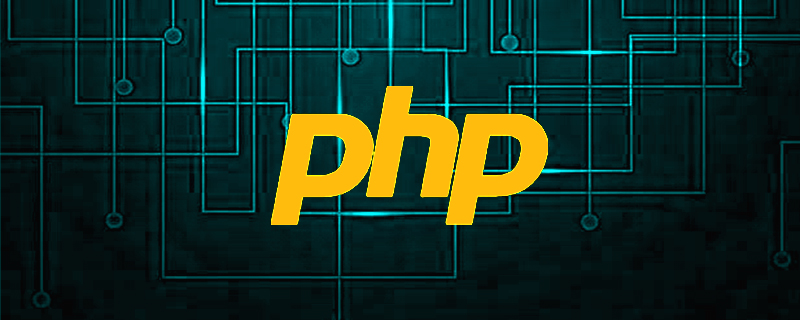
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
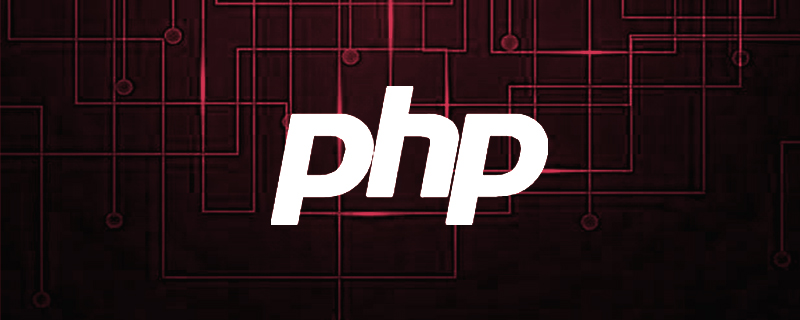
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
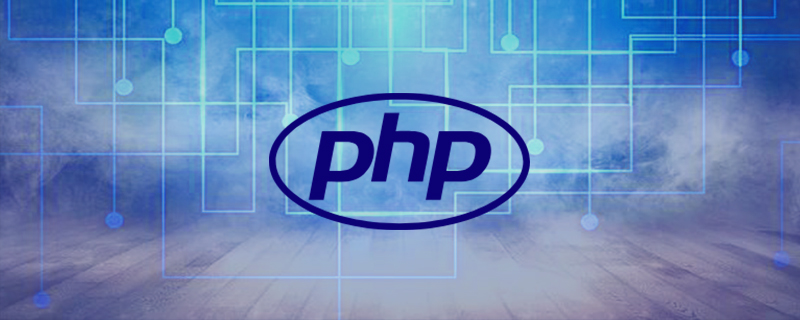
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
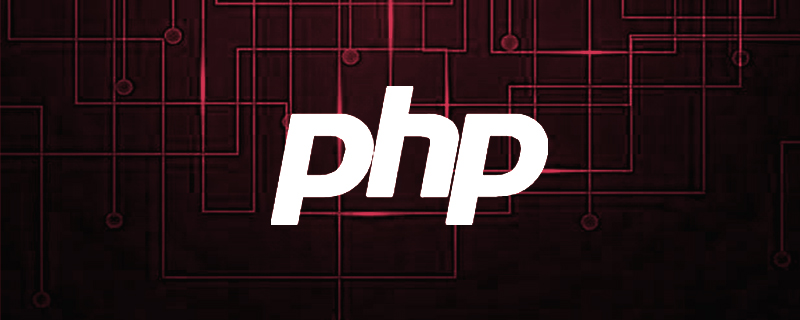
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
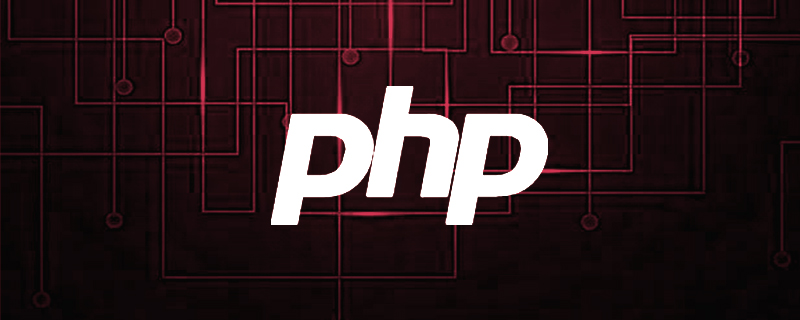
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
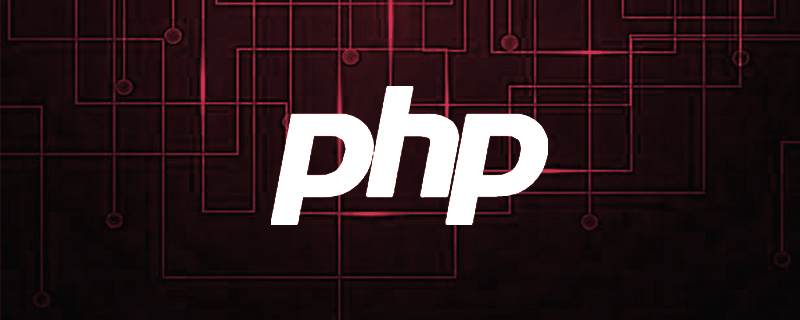
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
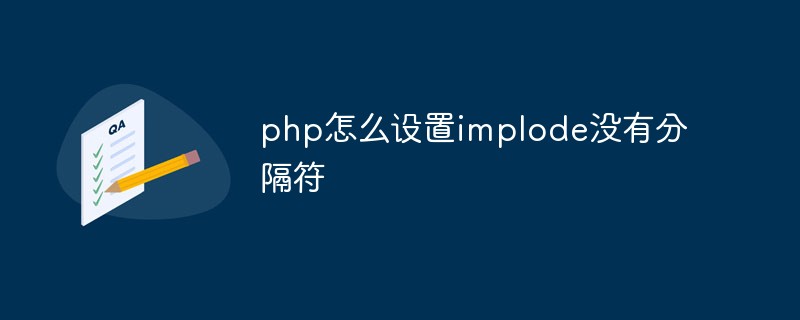
在PHP中,可以利用implode()函数的第一个参数来设置没有分隔符,该函数的第一个参数用于规定数组元素之间放置的内容,默认是空字符串,也可将第一个参数设置为空,语法为“implode(数组)”或者“implode("",数组)”。
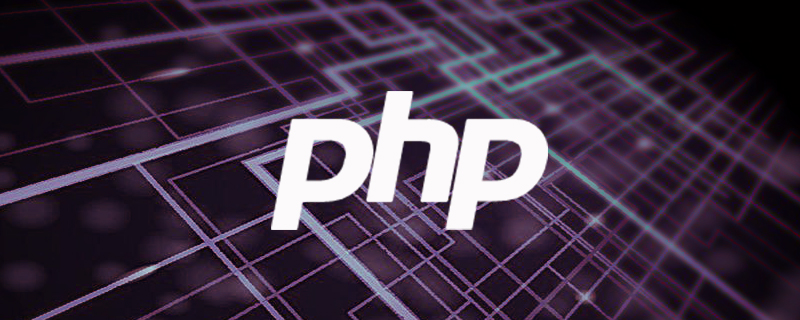
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
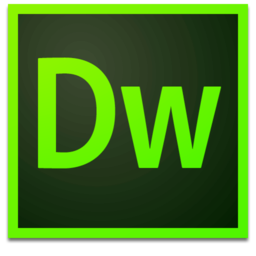
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
