As a Laravel developer, we often encounter various errors. Some are caused by coding errors, while others are caused by incorrect user input or improper operation. When these errors occur, a good error handling mechanism and error page are particularly important.
This article will introduce the error handling mechanism in Laravel and how to customize error pages and jumps. We will use Laravel 8 as an example.
Configuring Error Handler
In Laravel, all exceptions will be thrown to an exception handler. This handler is responsible for determining how to respond to these exceptions. Laravel provides a AppExceptionsHandler
class by default to handle all exceptions.
If you want to customize the exception response, you can edit it directly in the Handler
class. For example, you can customize the exception response through the render
method:
public function render($request, Throwable $exception) { if ($exception instanceof ModelNotFoundException) { return response()->json([ 'message' => 'Record not found' ], 404); } return parent::render($request, $exception); }
The above code demonstrates how to respond when the model is not found. Here we use response()->json
to return an error response in JSON format. If you want to return a view, you can use the view()
helper function.
Custom HTTP error page
In addition to the exception handler, we also need to customize the HTTP error page. Laravel makes this process very easy. We only need to create the corresponding view in the resources/views/errors
directory. For example:
-
resources/views/errors/404.blade.php
will handle HTTP 404 error -
resources/views/errors/500. blade.php
Will handle HTTP 500 errors
Note that you still need to beautify and style these views. Here we only provide the most basic response.
It should be noted that if your application is running in production mode and the sample data has not been set, then you may need to modify the APP_DEBUG
environment variable to disable debug mode and ensure Enable caching.
Custom Redirects
In addition to custom error responses, we can also customize redirects in the application. The most common redirect situation is when the user is not authenticated and we need to redirect them to the login page. In Laravel, use middleware
to protect routes, controller methods, etc.
For example, we can use auth
middleware to protect dashboard
routing:
Route::get('/dashboard', function () { // })->middleware(['auth']);
When the user is not authenticated, Laravel will automatically The user is redirected to the login
route, which is a function of Laravel's built-in authorization middleware. If you want to customize your redirects, you can use the RedirectIfAuthenticated
middleware, which can redirect the user to a specific page when they are already logged in.
For example, to redirect a logged in user from the /login
page to /dashboard
:
public function handle($request, Closure $next) { if (Auth::check()) { return redirect('/dashboard'); } return $next($request); }
This is how to handle errors in Laravel and custom redirection methods. Custom error pages and redirects can certainly improve your application’s user experience and improve your application’s accessibility. Of course, as we said, when encountering unknown errors, we should also give friendly error prompts to help users determine how to solve the problem.
The above is the detailed content of laravel error jump. For more information, please follow other related articles on the PHP Chinese website!
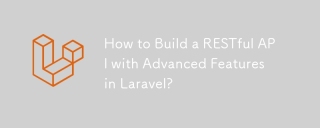
This article guides building robust Laravel RESTful APIs. It covers project setup, resource management, database interactions, serialization, authentication, authorization, testing, and crucial security best practices. Addressing scalability chall
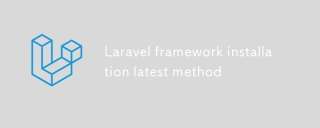
This article provides a comprehensive guide to installing the latest Laravel framework using Composer. It details prerequisites, step-by-step instructions, troubleshooting common installation issues (PHP version, extensions, permissions), and minimu
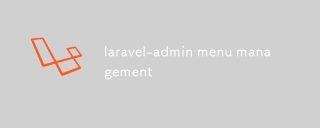
This article guides Laravel-Admin users on menu management. It covers menu customization, best practices for large menus (categorization, modularization, search), and dynamic menu generation based on user roles and permissions using Laravel's author
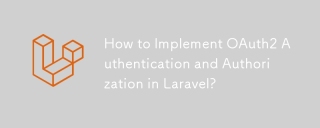
This article details implementing OAuth 2.0 authentication and authorization in Laravel. It covers using packages like league/oauth2-server or provider-specific solutions, emphasizing database setup, client registration, authorization server configu
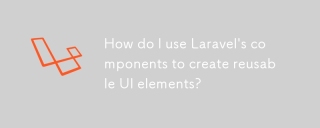
The article discusses creating and customizing reusable UI elements in Laravel using components, offering best practices for organization and suggesting enhancing packages.
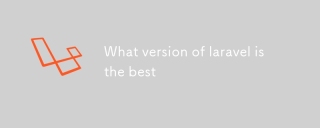
This article guides Laravel developers in choosing the right version. It emphasizes the importance of selecting the latest Long Term Support (LTS) release for stability and security, while acknowledging that newer versions offer advanced features.
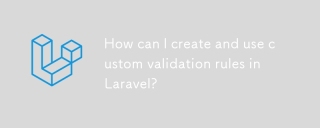
The article discusses creating and using custom validation rules in Laravel, offering steps to define and implement them. It highlights benefits like reusability and specificity, and provides methods to extend Laravel's validation system.
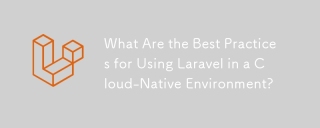
The article discusses best practices for deploying Laravel in cloud-native environments, focusing on scalability, reliability, and security. Key issues include containerization, microservices, stateless design, and optimization strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
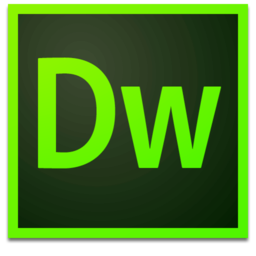
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
