Image processing functions in PHP
PHP, as a popular scripting language, provides many useful functions in image processing. This article will introduce some commonly used PHP image processing functions.
- gd library
The GD library is an open source graphics library that can dynamically generate images, including saving images in multiple formats. The GD library supports multiple formats including JPG, PNG, GIF, etc. By using the GD library, you can create complex images, add various texts, and various effects such as shadows, tilt, and more in PHP.
Creating an image is very simple, you just need to specify the image width and height using the imagecreatetruecolor() function.
<?php $width = 400; //设置图像宽度 $height = 300; //设置图像高度 $image = imagecreatetruecolor($width, $height); //创建图像 ?>
Executing the above code will create a new image. Next, we can add text to this image, draw lines, add various effects, and more. The following are some commonly used image processing functions.
- Image cutting
Image cropping is a common operation. You can cut an image to a specified length and width through the imagecrop() function. The following is a sample code for this function:
<?php $srcImage = imagecreatefromjpeg('source.jpg'); //加载源图像 $cropped = imagecrop($srcImage, ['x' => 0, 'y' => 0, 'width' => 200, 'height' => 200]); //剪切图像 ?>
- Image resizing
Scaling an image is a common processing method, and you can use the imagescale() function to scale the image. Here is the sample code for this function:
<?php $image = imagecreatefromjpeg('source.jpg'); //加载图像 $scale = 0.5; //缩放比例 $width = imagesx($image) * $scale; //计算新的宽度 $height = imagesy($image) * $scale; //计算新的高度 $newImage = imagescale($image, $width, $height); //缩放图像 ?>
In the above example, we have reduced the source image by 50%.
- Image rotation
Rotating images is a more complex processing method that can be achieved using the imagerotate() function. Here is the sample code for this function:
<?php $image = imagecreatefromjpeg('source.jpg'); //加载图像 $angle = 45; //旋转角度 $newImage = imagerotate($image, $angle, 0); //旋转图像 ?>
In this example, we rotate the image 45 degrees.
- Add watermark
Adding watermark is a common operation and can be achieved using the imagestring() function. Here is the sample code for this function:
<?php $image = imagecreatefromjpeg('source.jpg'); //加载图像 $textColor = imagecolorallocate($image, 255, 255, 255); //设置文本颜色 $fontSize = 16; //设置字体大小 $text = 'www.example.com'; //设定水印文本 imagestring($image, $fontSize, 10, 10, $text, $textColor); //添加水印 ?>
In the above example, we are adding a text watermark to the image.
Summary
PHP provides numerous image processing functions, including cutting, scaling, rotating, adding watermarks, etc. The gd library is one of the most commonly used libraries, but there are other libraries available, such as ImageMagick. By using these functions, you can easily implement various image processing operations in PHP.
The above is the detailed content of Image processing functions in PHP. For more information, please follow other related articles on the PHP Chinese website!
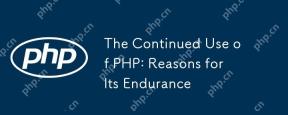
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
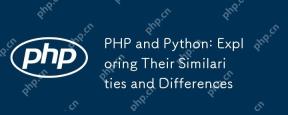
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
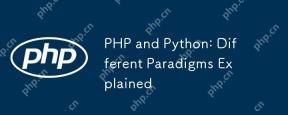
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
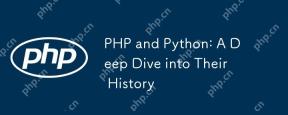
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
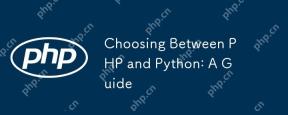
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
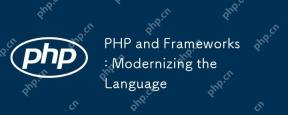
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
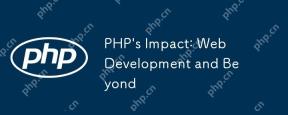
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
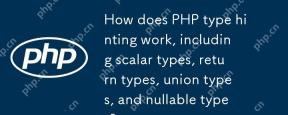
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
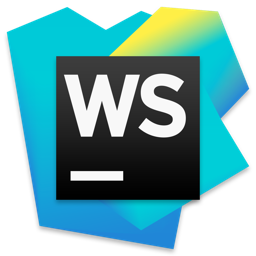
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
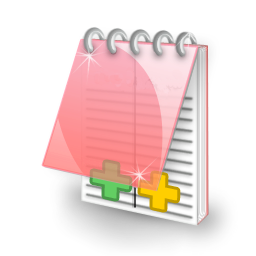
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software