Overview and classification of cache
Overview
Cache is a piece of memory space that saves temporary data
Why use Cache
Read the data from the data source (database or file) and store it in the cache. When retrieving it, obtain it directly from the cache, which can reduce the number of interactions with the database, which can improve the performance of the program. Performance!
Applicability of cache
Suitable for cache: frequently queried but not frequently modified (eg: provinces, cities, category data), the accuracy of the data has no impact on the final result Big
Not suitable for caching: frequently changing data, sensitive data (for example: stock market prices, bank exchange rates, money in bank cards), etc.
MyBatis Cache Category
Level 1 cache: It is the cache of the sqlSession object. It comes with it (no configuration required) and cannot be uninstalled (if you don’t want to use it). The life cycle of the first level cache is consistent with sqlSession.
Second level cache: It is the cache of SqlSessionFactory. As long as the SqlSession created by the same SqlSessionFactory shares the contents of the second-level cache, it can operate the second-level cache. If we want to use the second-level cache, we need to manually enable it ourselves (configuration is required).
Use of second-level cache
1. Enable the second-level cache in the core configuration file of mybatis
<!--**因为 cacheEnabled 的取值默认就为 true**,所以这一步可以省略不配置。为 true 代表开启二级缓存;为 false 代表不开启二级缓存。 --> <settings> <setting name="cacheEnabled" value="true"/> </settings>
2. Configure the use of the second-level cache in the Dao mapping file
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.itheima.dao.UserDao"> <!--配置二级缓存--> <cache/> <select id="findAll" resultType="user"> select * from t_user </select> <delete id="deleteById" parameterType="int"> delete from t_user where uid=#{id} </delete> </mapper>
3. The Pojo class for second-level caching must implement the Serializable interface
public class User implements Serializable { private int uid; private String username; private String sex; private Date birthday; private String address; // 省略setter,getter,构造...等方法 }
4. Test the use of second-level cache
Test code
@Test public void testFindAll() throws Exception{ // 1.加载mybatis核心配置文件 InputStream is = Resources.getResourceAsStream("SqlMapConfig.xml"); // 2.创建SqlSessionFactoryBuilder对象 SqlSessionFactoryBuilder sqlSessionFactoryBuilder = new SqlSessionFactoryBuilder(); // 3.构建SqlSessionFactory对象 SqlSessionFactory sqlSessionFactory = sqlSessionFactoryBuilder.build(is); // 4.获取SqlSession对象 SqlSession sqlSession = sqlSessionFactory.openSession(); // 5.获得dao接口的代理对象 UserDao userDao = sqlSession.getMapper(UserDao.class); // 6.执行sql语句,得到结果 List<User> list = userDao.findAll(); for (User user : list) { System.out.println("user = " + user); } sqlSession.close();//清除一级缓存 System.out.println("分割线----------------------------------"); SqlSession sqlSession2 = sqlSessionFactory.openSession(); UserDao userDao2 = sqlSession2.getMapper(UserDao.class); List<User> userList2 = userDao2.findAll(); for (User user : userList2) { System.out.println(user); } // 7.释放资源 sqlSession2.close(); }
- Test results:
#- After the above test, we found that two queries were executed, and after executing the first query, we turned off the first-level cache and then went to When executing the second query, we found that no SQL statement was issued to the database, so the data at this time can only come from what we call the second-level cache.
5. Test to turn off the second-level cache
-Test code
@Test public void testFindAll() throws Exception{ // 1.加载mybatis核心配置文件 InputStream is = Resources.getResourceAsStream("SqlMapConfig.xml"); // 2.创建SqlSessionFactoryBuilder对象 SqlSessionFactoryBuilder sqlSessionFactoryBuilder = new SqlSessionFactoryBuilder(); // 3.构建SqlSessionFactory对象 SqlSessionFactory sqlSessionFactory = sqlSessionFactoryBuilder.build(is); // 4.获取SqlSession对象 SqlSession sqlSession = sqlSessionFactory.openSession(); // 5.获得dao接口的代理对象 UserDao userDao = sqlSession.getMapper(UserDao.class); // 6.执行sql语句,得到结果 List<User> list = userDao.findAll(); for (User user : list) { System.out.println("user = " + user); } sqlSession.close();//清除一级缓存 System.out.println("分割线----------------------------------"); SqlSession sqlSession2 = sqlSessionFactory.openSession(); UserDao userDao2 = sqlSession2.getMapper(UserDao.class); userDao2.deleteById(5);// 关闭二级缓存 List<User> userList2 = userDao2.findAll(); for (User user : userList2) { System.out.println(user); } // 7.释放资源 sqlSession2.close(); }
-Test result
After the above Testing, we found that two queries were executed, and after executing the first query, we closed the first-level cache and the second-level cache. When we executed the second query, we found that a sql statement was issued to the database, so The data at this time comes from the database, not the cache.
The above is the detailed content of How to use the second-level cache of Mybatis in Java. For more information, please follow other related articles on the PHP Chinese website!
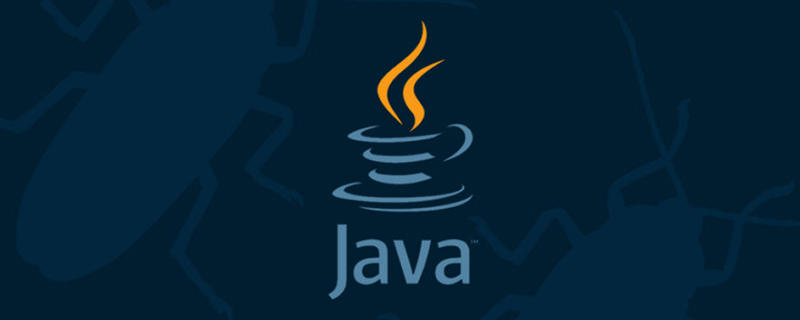
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
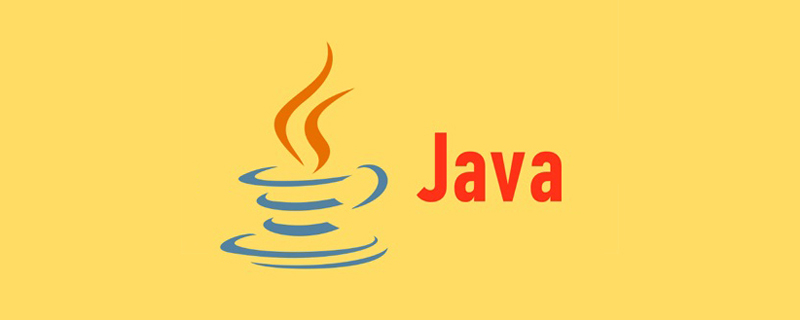
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
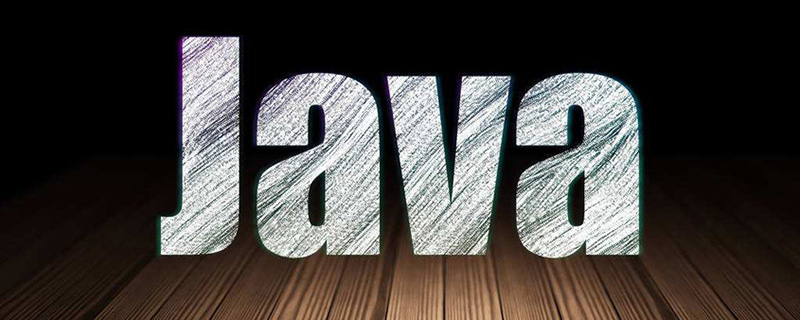
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
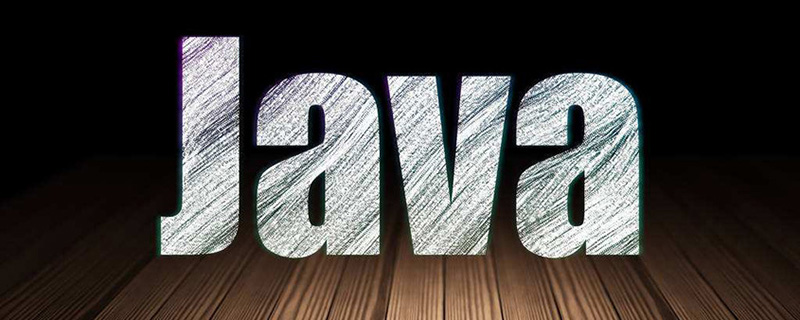
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
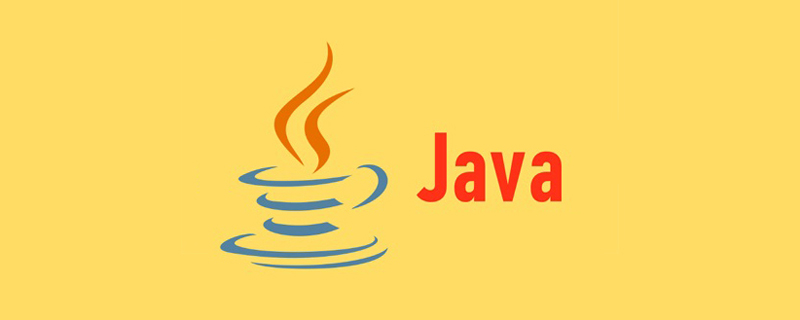
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
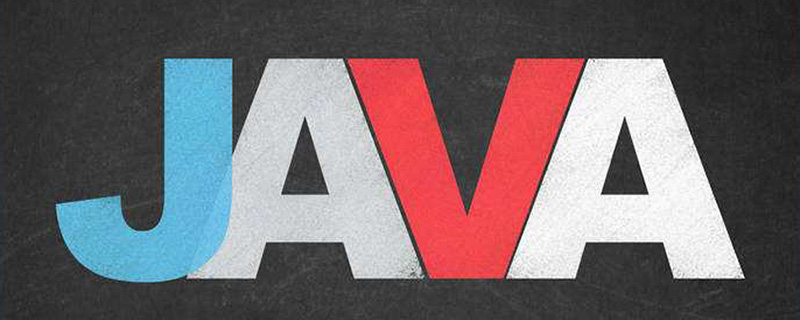
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
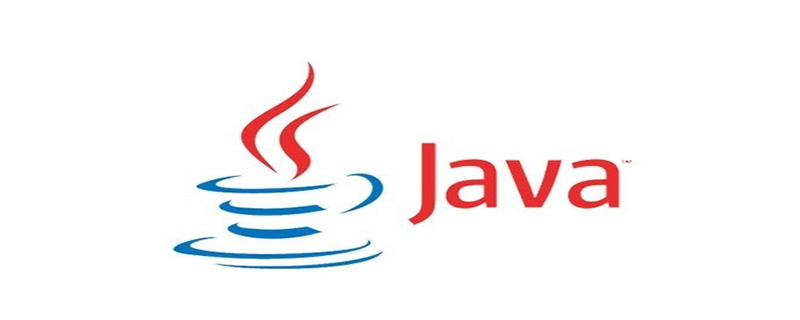
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。
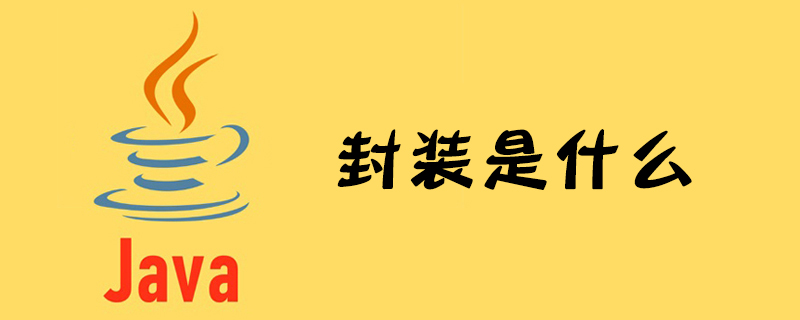
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
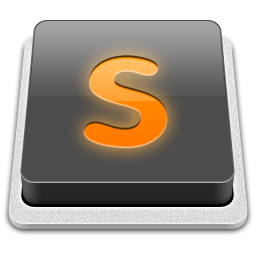
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.