As a popular front-end framework, Vue has powerful data binding and component development capabilities. However, in actual projects, we often encounter the need to call methods in components across levels. This article will introduce how to use Vue's component communication mechanism to implement cross-level calling methods in components.
1. Global event bus
Vue provides a very simple global event bus-event bus. The event center is a global Vue instance that can be accessed by all components and is used for cross-component communication. Typically, we create an event center in the root instance of Vue.
- Create a global event center
In the root component, we can use the "$emit" method of the Vue instance to dispatch events to the event center, or we can use "$ on" method listens to events in the event center. The following is a sample code:
// 在 main.js 中创建事件中心 import Vue from 'vue' export const EventBus = new Vue() // 在组件中派发事件 import { EventBus } from './main.js' EventBus.$emit('my-event', data) // 在组件中监听事件 import { EventBus } from './main.js' EventBus.$on('my-event', data => { // 处理事件 })
- Using the global event center to call methods across components
Using the event center, we can implement cross-component calling methods. In component A, use the "$emit" method to dispatch events to the event center; in component B, use the "$on" method to listen for events, and then call the method of component A. The following is an example of implementation:
// 组件A export default { methods: { myMethod() { console.log('Hello, world!') } } } // 组件B import { EventBus } from './main.js' export default { mounted() { EventBus.$on('my-event', () => { this.$refs.componentA.myMethod() }) } }
In the above code, "this.$refs.componentA" represents the subcomponent named "componentA" mounted in component B. In this way, we can The method of component A is called in component B.
2. $refs
In addition to the event center, Vue also provides a simple way to access component instances - $refs. This is an object that contains instances of all child components in the current component that reference the "ref" attribute. We can call child component methods by accessing the $refs object.
- Define $ref
In a template, we can reference child components by defining the "ref" attribute. The following is an example of implementation:
// 子组件 <template> <div ref="myComponent">Hello, world!</div> </template>
In the above code, we define a "ref" named "myComponent" in the template of the child component. This "ref" can be accessed in the parent component through the $refs object.
- Use $refs to call methods in the component
In the parent component, we can use the $refs object to access the methods in the child component. The following is an example of implementation:
// 子组件 export default { methods: { myMethod() { console.log('Hello, world!') } } } // 父组件 export default { mounted() { this.$refs.myComponent.myMethod() } }
In the above code, in the mounted hook of the parent component, we use "this.$refs.myComponent" to obtain the child component instance and call its internal method .
Summary:
This article introduces the use of event center and $refs to implement cross-level calling methods between Vue components. Event centers are suitable for communication between non-parent and child components, while $refs are suitable for communication between parent and child components. In actual development, we choose the appropriate method based on the actual situation to better manage the state and interaction of components.
The above is the detailed content of How vue calls methods in components across levels. For more information, please follow other related articles on the PHP Chinese website!

React'slimitationsinclude:1)asteeplearningcurveduetoitsvastecosystem,2)SEOchallengeswithclient-siderendering,3)potentialperformanceissuesinlargeapplications,4)complexstatemanagementasappsgrow,and5)theneedtokeepupwithitsrapidevolution.Thesefactorsshou

Reactischallengingforbeginnersduetoitssteeplearningcurveandparadigmshifttocomponent-basedarchitecture.1)Startwithofficialdocumentationforasolidfoundation.2)UnderstandJSXandhowtoembedJavaScriptwithinit.3)Learntousefunctionalcomponentswithhooksforstate

ThecorechallengeingeneratingstableanduniquekeysfordynamiclistsinReactisensuringconsistentidentifiersacrossre-rendersforefficientDOMupdates.1)Usenaturalkeyswhenpossible,astheyarereliableifuniqueandstable.2)Generatesynthetickeysbasedonmultipleattribute

JavaScriptfatigueinReactismanageablewithstrategieslikejust-in-timelearningandcuratedinformationsources.1)Learnwhatyouneedwhenyouneedit,focusingonprojectrelevance.2)FollowkeyblogsliketheofficialReactblogandengagewithcommunitieslikeReactifluxonDiscordt

TotestReactcomponentsusingtheuseStatehook,useJestandReactTestingLibrarytosimulateinteractionsandverifystatechangesintheUI.1)Renderthecomponentandcheckinitialstate.2)Simulateuserinteractionslikeclicksorformsubmissions.3)Verifytheupdatedstatereflectsin

KeysinReactarecrucialforoptimizingperformancebyaidinginefficientlistupdates.1)Usekeystoidentifyandtracklistelements.2)Avoidusingarrayindicesaskeystopreventperformanceissues.3)Choosestableidentifierslikeitem.idtomaintaincomponentstateandimproveperform

Reactkeysareuniqueidentifiersusedwhenrenderingliststoimprovereconciliationefficiency.1)TheyhelpReacttrackchangesinlistitems,2)usingstableanduniqueidentifierslikeitemIDsisrecommended,3)avoidusingarrayindicesaskeystopreventissueswithreordering,and4)ens

UniquekeysarecrucialinReactforoptimizingrenderingandmaintainingcomponentstateintegrity.1)Useanaturaluniqueidentifierfromyourdataifavailable.2)Ifnonaturalidentifierexists,generateauniquekeyusingalibrarylikeuuid.3)Avoidusingarrayindicesaskeys,especiall


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
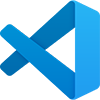
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
