To test React components using the useState hook, use Jest and React Testing Library to simulate interactions and verify state changes in the UI. 1) Render the component and check initial state. 2) Simulate user interactions like clicks or form submissions. 3) Verify the updated state reflects in the UI. Focus on behavior, not implementation details, and handle asynchronous updates with waitFor.
When testing React components that use the useState
hook, you're essentially looking at how state changes affect your component's behavior and rendering. It's about ensuring that your component responds correctly to state updates.
Let's dive into the world of testing these dynamic components.
Imagine you're crafting a piece of art, and every stroke of the brush changes the canvas. That's what useState
does in your React components—it lets you paint with state changes. Now, testing these components is like critiquing your own artwork, ensuring each stroke (or state change) results in the masterpiece you envisioned.
To start, you'll want to use a testing library like Jest along with React Testing Library. These tools help you simulate user interactions and check the component's output. Here's how you can approach this:
import React from 'react'; import { render, fireEvent } from '@testing-library/react'; import Counter from './Counter'; test('increments counter', () => { const { getByText } = render(<Counter />); const incrementButton = getByText('Increment'); // Initial state check expect(getByText('Count: 0')).toBeInTheDocument(); // Simulate a click fireEvent.click(incrementButton); // Check the new state expect(getByText('Count: 1')).toBeInTheDocument(); });
This test checks if clicking the 'Increment' button correctly updates the state from 0 to 1. But what if you're dealing with more complex state interactions? Let's say you have a form that uses multiple state variables. Here's how you might test that:
import React from 'react'; import { render, fireEvent } from '@testing-library/react'; import Form from './Form'; test('form submission updates state', () => { const { getByLabelText, getByText } = render(<Form />); // Fill in the form fireEvent.change(getByLabelText('Name:'), { target: { value: 'John Doe' } }); fireEvent.change(getByLabelText('Email:'), { target: { value: 'john@example.com' } }); // Submit the form fireEvent.click(getByText('Submit')); // Check the updated state expect(getByText('Name: John Doe')).toBeInTheDocument(); expect(getByText('Email: john@example.com')).toBeInTheDocument(); });
Now, let's talk about the nuances and potential pitfalls. One common mistake is testing implementation details rather than behavior. You want to focus on what the user sees and interacts with, not on how the state is managed internally.
For instance, avoid tests like this:
// Bad practice: Testing implementation details test('state is updated', () => { const { container } = render(<Counter />); const instance = container.firstChild._reactInternals; expect(instance.memoizedState).toBe(0); });
This test looks at the internal state of the component, which can be fragile and change with React's internals. Instead, focus on the rendered output and user interactions.
Another aspect to consider is testing asynchronous state updates. If your component uses useEffect
to update state asynchronously, you'll need to use waitFor
from React Testing Library to ensure your assertions run after the state has updated:
import { waitFor } from '@testing-library/react'; test('async state update', async () => { const { getByText } = render(<AsyncCounter />); fireEvent.click(getByText('Fetch Count')); await waitFor(() => { expect(getByText('Count: 10')).toBeInTheDocument(); }); });
When it comes to performance optimization and best practices, remember that over-testing can lead to slow test suites. Focus on the critical paths of your component. Also, consider using act
from ReactDOM/test-utils to wrap your state updates and ensure they're processed correctly:
import { act } from 'react-dom/test-utils'; test('counter increments', () => { let container; act(() => { container = render(<Counter />).container; }); const button = container.querySelector('button'); const label = container.querySelector('p'); act(() => { button.dispatchEvent(new MouseEvent('click', { bubbles: true })); }); expect(label.textContent).toBe('Count: 1'); });
In wrapping up, testing components with useState
is about ensuring your component's state changes are reflected correctly in the UI. It's an art and a science—testing not just for correctness but also for user experience. Keep your tests focused on behavior, use the right tools to simulate interactions, and don't forget to consider asynchronous updates. With these practices, you'll ensure your React components are robust and reliable.
The above is the detailed content of Testing Components That Use the useState() Hook. For more information, please follow other related articles on the PHP Chinese website!

React'slimitationsinclude:1)asteeplearningcurveduetoitsvastecosystem,2)SEOchallengeswithclient-siderendering,3)potentialperformanceissuesinlargeapplications,4)complexstatemanagementasappsgrow,and5)theneedtokeepupwithitsrapidevolution.Thesefactorsshou

Reactischallengingforbeginnersduetoitssteeplearningcurveandparadigmshifttocomponent-basedarchitecture.1)Startwithofficialdocumentationforasolidfoundation.2)UnderstandJSXandhowtoembedJavaScriptwithinit.3)Learntousefunctionalcomponentswithhooksforstate

ThecorechallengeingeneratingstableanduniquekeysfordynamiclistsinReactisensuringconsistentidentifiersacrossre-rendersforefficientDOMupdates.1)Usenaturalkeyswhenpossible,astheyarereliableifuniqueandstable.2)Generatesynthetickeysbasedonmultipleattribute

JavaScriptfatigueinReactismanageablewithstrategieslikejust-in-timelearningandcuratedinformationsources.1)Learnwhatyouneedwhenyouneedit,focusingonprojectrelevance.2)FollowkeyblogsliketheofficialReactblogandengagewithcommunitieslikeReactifluxonDiscordt

TotestReactcomponentsusingtheuseStatehook,useJestandReactTestingLibrarytosimulateinteractionsandverifystatechangesintheUI.1)Renderthecomponentandcheckinitialstate.2)Simulateuserinteractionslikeclicksorformsubmissions.3)Verifytheupdatedstatereflectsin

KeysinReactarecrucialforoptimizingperformancebyaidinginefficientlistupdates.1)Usekeystoidentifyandtracklistelements.2)Avoidusingarrayindicesaskeystopreventperformanceissues.3)Choosestableidentifierslikeitem.idtomaintaincomponentstateandimproveperform

Reactkeysareuniqueidentifiersusedwhenrenderingliststoimprovereconciliationefficiency.1)TheyhelpReacttrackchangesinlistitems,2)usingstableanduniqueidentifierslikeitemIDsisrecommended,3)avoidusingarrayindicesaskeystopreventissueswithreordering,and4)ens

UniquekeysarecrucialinReactforoptimizingrenderingandmaintainingcomponentstateintegrity.1)Useanaturaluniqueidentifierfromyourdataifavailable.2)Ifnonaturalidentifierexists,generateauniquekeyusingalibrarylikeuuid.3)Avoidusingarrayindicesaskeys,especiall


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
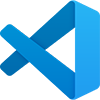
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
