Getting Started with PHP: PATCH Requests and Responses
With the continuous development of the Internet, the demand for front-end and back-end technologies is also increasing. As a back-end developer, mastering PHP is essential. In PHP development, we often need to process requests and responses. This article will discuss the PATCH request and response, providing a practical guide for PHP beginners.
1. PATCH request
PATCH request is an HTTP request method, which is used to update existing resources. In HTTP protocol, there is a way to update using PUT request. But PUT does have a problem, that is, if we only need to update part of the content instead of all the content when performing an update operation, then the PUT request will overwrite the unmodified content. The PATCH request solves this problem. Its function is to update only the resources specified in the request body.
So, how to send a PATCH request? The following is a simple example:
<?php $url = 'http://www.example.com/resource'; $data = array('field' => 'value'); $options = array( 'http' => array( 'header' => "Content-type: application/json ", 'method' => 'PATCH', 'content' => json_encode($data) ) ); $context = stream_context_create($options); $result = file_get_contents($url, false, $context); $response = json_decode($result); ?>
In this example, we use the file_get_contents function to send a PATCH request. We need to encode the data in the request body into JSON format and then put it into the content item of the options array. Create an HTTP context through the stream_context_create() function and then pass it as the third parameter to the file_get_contents() function.
2. PATCH response
When receiving the PATCH request, the server will process it accordingly and return the response result. So, how to handle the PATCH response? We can use PHP's curl library for processing. The following is a simple example:
<?php $url = 'http://www.example.com/resource'; $data = array('field' => 'value'); $ch = curl_init($url); curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'PATCH'); curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data)); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); $json = json_decode($response); ?>
In this example, we use the curl_init() function to initialize the request, and use the curl_setopt() function to set the request method, request body, and return result. Finally, we send the request using the curl_exec() function and close the request using the curl_close() function.
Summary
Understanding PATCH requests and responses is very important for beginners of PHP. The difference between a PATCH request and a PUT request is that only the resources specified in the request body are updated, rather than re-uploading the entire resource. The difference between a PATCH response and a PUT response is that the response text only contains changed fields. Mastering the use of PATCH allows us to process requests and responses more efficiently. I hope this article can be helpful to PHP beginners.
The above is the detailed content of Getting Started with PHP: PATCH Requests and Responses. For more information, please follow other related articles on the PHP Chinese website!
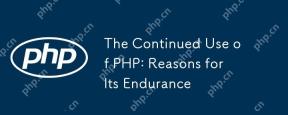
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
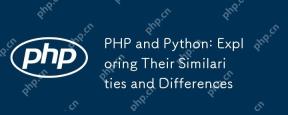
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
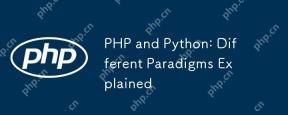
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
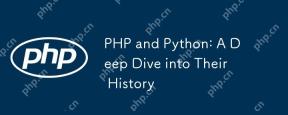
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
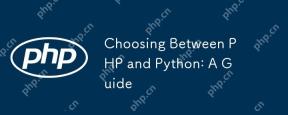
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
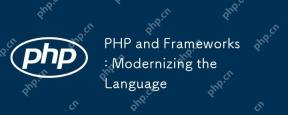
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
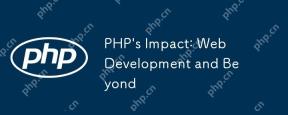
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
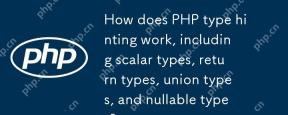
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
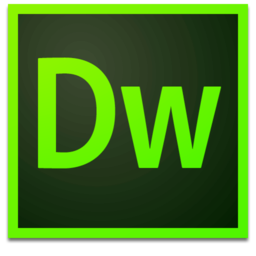
Dreamweaver Mac version
Visual web development tools
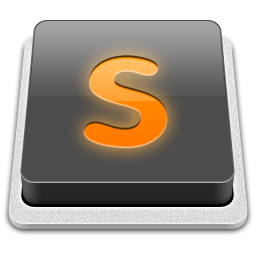
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
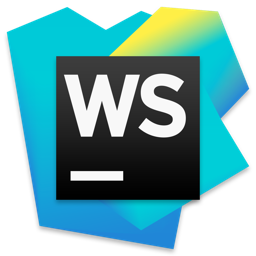
WebStorm Mac version
Useful JavaScript development tools