When developing using PHP, we often encounter the need to sum the values in an array. At this time, we can use some functions provided by PHP to achieve this.
PHP provides two functions to calculate the sum of the values in an array: array_sum() and array_reduce().
The array_sum() function is used to calculate the sum of all numbers in the array, while the array_reduce() function is used to iterate over the values in the array, accumulate them according to the callback function, and finally return an accumulated result. .
For simple calculations, the array_sum() function is the most convenient. For example, the following example adds all the values in an array containing numbers:
$numbers = array(1, 2, 3, 4, 5); $total = array_sum($numbers); echo 'The total is: ' . $total;
Output:
The total is: 15
In the above code, $numbers is an array containing the numbers 1 to 5. Then, use the array_sum() function to calculate the sum of all numbers in the array, capture the result in the variable $total, and finally output the result.
If the array contains non-numeric values, they are ignored. For example, in the following example, although the array contains the string 'hello' which is not a number, it will be ignored and will not affect the calculation result:
$numbers = array(1, 'hello', 2, 3, 4, 5); $total = array_sum($numbers); echo 'The total is: ' . $total;
Output:
The total is: 15
If To calculate the sum of all numbers in a multidimensional array, you can use the array_walk_recursive() function. For example, the following example adds all the numbers in a multidimensional array:
$data = array( array(1, 2, 3), array(4, 5, 6), array( array(7, 8), array(9, 10) ) ); $total = 0; array_walk_recursive($data, function ($value) use (&$total) { $total += $value; }); echo 'The total is: ' . $total;
Output:
The total is: 55
In the above code, $data is an array containing multidimensional arrays. Then, using the array_walk_recursive() function, each number in the array is passed into an anonymous function, where it is accumulated. Finally, the result is stored in the variable $total and the result is output.
In addition to using the array_sum() function and array_reduce() function, we can also use a loop to calculate the sum of all numbers in the array. The following is an example of using a foreach loop:
$numbers = array(1, 2, 3, 4, 5); $total = 0; foreach ($numbers as $number) { $total += $number; } echo 'The total is: ' . $total;
Output:
The total is: 15
In the above code, the loop traverses all the values in the array $numbers and adds each value to In the variable $total, the final result is output.
In general, calculating the sum of all numbers in an array is a very basic requirement. PHP provides a variety of ways to complete this task. Developers can choose the method that suits them according to the actual situation.
The above is the detailed content of php sum array values. For more information, please follow other related articles on the PHP Chinese website!
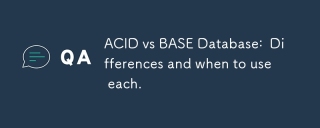
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
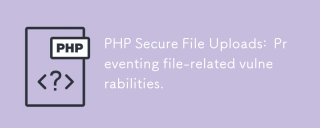
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
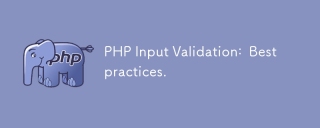
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
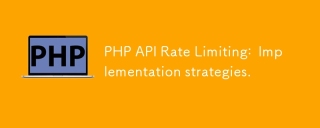
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
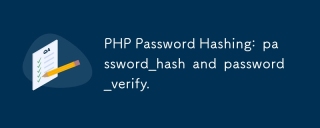
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
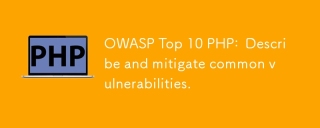
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
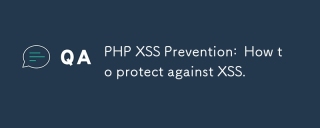
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
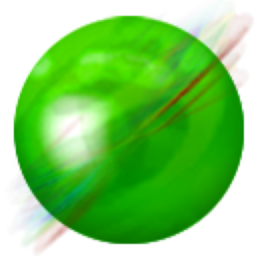
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
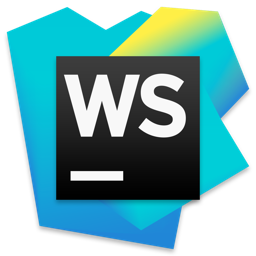
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version