RSS (Rich Site Summary) is an XML format standard used to push updated information to users. For blogs, news websites, or other types of content publishers, providing RSS subscriptions can keep users informed of updated content. In this article, we will discuss how to implement RSS subscription using PHP.
Step one: Generate RSS source
To implement the RSS subscription function, you first need to generate an RSS source (XML file) and store it in the directory of the web server. RSS feeds contain metadata about the content to be subscribed to, such as title, link, description, publication time, etc. The following is a simple RSS feed template example:
<?xml version="1.0" encoding="UTF-8"?> <rss version="2.0"> <channel> <title>我的博客</title> <link>http://www.example.com</link> <description>这是我的博客</description> <lastBuildDate><?=date("r")?></lastBuildDate> <item> <title>文章1</title> <link>http://www.example.com/articles/1</link> <description>这是文章1的描述</description> <pubDate><?=date("r")?></pubDate> </item> <item> <title>文章2</title> <link>http://www.example.com/articles/2</link> <description>这是文章2的描述</description> <pubDate><?=date("r")?></pubDate> </item> <!--更多文章--> </channel> </rss>
In this example, we use the RSS 2.0 version and define an RSS feed that contains more basic elements such as title, link, description, and release time. . We used PHP's date() function to dynamically generate the latest publishing time (lastBuildDate and pubDate).
Step 2: Output the RSS source
Next, we need to output the RSS source to the browser so that users can subscribe to it. In PHP, we can use the header() function to specify the MIME type as application/rss xml and output the RSS source:
<?php header("Content-Type: application/rss+xml; charset=utf-8"); echo file_get_contents("rss.xml"); ?>
In this example, we use the file_get_contents() function to read the RSS source file (rss.xml), and then use echo to output to the browser. This way, when users access this PHP file, they will see the generated RSS feed and can subscribe to it through their browser or other RSS reader.
Step Three: Implement RSS Subscription
Now that we have generated a subscribeable RSS feed, the next step is to enable users to subscribe to it. For this we can use an open source PHP library such as SimplePie or FeedWriter.
SimplePie is an open source PHP class library that can be used to parse and display RSS and Atom sources. It supports a variety of content formats and subscription formats, and is easy to use. The following is sample code for subscribing to an RSS feed using SimplePie:
require_once('simplepie.inc'); $feed = new SimplePie(); $feed->set_feed_url('http://www.example.com/rss.php'); $feed->init(); $feed->handle_content_type(); foreach ($feed->get_items() as $item) { echo $item->get_title(); echo $item->get_permalink(); }
In this example, we first include the files of the SimplePie library, then create a new SimplePie object ($feed) and specify the information to subscribe to The URL of the RSS feed. We used the set_feed_url() function to set the RSS feed URL and the init() function to initialize SimplePie. Finally, we use the get_items() function to iterate through each item of the RSS feed and output the title and URL.
FeedWriter is another open source PHP library that can be used to generate and write RSS and Atom feeds. It can easily create and edit a variety of content and supports various RSS and Atom versions. The following is an example of using FeedWriter to generate an RSS feed:
require_once('FeedWriter.php'); $feed = new FeedWriter(RSS2); $feed->setTitle('我的博客'); $feed->setLink('http://www.example.com'); $feed->setDescription('这是我的博客'); $feed->setChannelElements(array('language'=>'zh-cn')); $item = $feed->createNewItem(); $item->setTitle('文章1'); $item->setLink('http://www.example.com/articles/1'); $item->setDescription('这是文章1的描述'); $item->setDate(time()); $feed->addItem($item); $item = $feed->createNewItem(); $item->setTitle('文章2'); $item->setLink('http://www.example.com/articles/2'); $item->setDescription('这是文章2的描述'); $item->setDate(time()); $feed->addItem($item); $feed->generateFeed();
In this example, we first include the files of the FeedWriter library, then create a new FeedWriter object ($feed) and set the RSS version to RSS 2.0. We used the setTitle, setLink, setDescription and setChannelElements functions to set the title, link, description and language elements of the RSS feed. Next, we created each RSS item ($item) using the createNewItem function and added them to the RSS feed using the addItem function. Finally, we generate the RSS feed using the generateFeed function.
Conclusion
In this article, we introduced how to use PHP to implement RSS subscription functionality. First, we create an RSS source file and use header and echo to output it to the browser. Then, we introduced two PHP class libraries, SimplePie and FeedWriter, which can be used to parse, generate and edit RSS feeds. Whether you create your own RSS feed or subscribe to another website's RSS feed, these tools will help you do just that.
The above is the detailed content of How to implement RSS subscription in PHP. For more information, please follow other related articles on the PHP Chinese website!
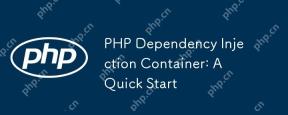
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
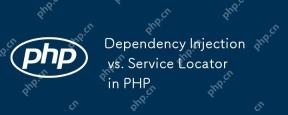
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
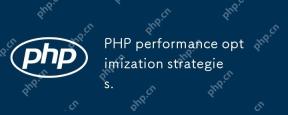
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
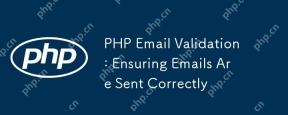
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
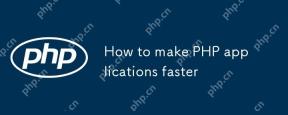
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
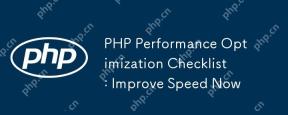
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
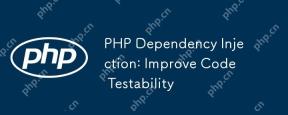
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
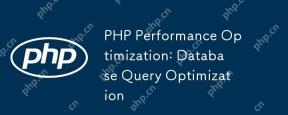
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
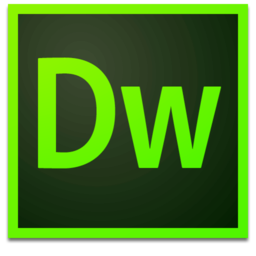
Dreamweaver Mac version
Visual web development tools
