Implementation of login window JavaScript
In daily website login operations, users need to enter their account number and password, and then click the "Login" button to complete the login operation. This login operation requires the cooperation of the front-end page and the back-end service to complete. This article will introduce how to use JavaScript to implement a simple login window.
- Front-end page design
We need to design the login window in html first. The simplest login window is a form, which contains two input boxes (account and password) and a submit button (login). We can use the <input>
tag to define input boxes and buttons, and then use the <form></form>
tag to define the form. The code is as follows:
<form> <label>账号</label> <input type="text" name="username" required /> <label>密码</label> <input type="password" name="password" required /> <button type="submit">登录</button> </form>
This form contains two input boxes, namely username
and password
. At the same time, a required
attribute is also specified to ensure that there must be a value in the input box. In order to make the internationalization more user-friendly and let users know what they need to input, the <label></label>
label is added to prompt the user to input items.
- JavaScript handles form submission
After the user enters their account number and password in the above form, they need to click the "Login" button to complete the submission operation. At this time we need to write JavaScript code to listen to the form submission event and process related logic. The code is as follows:
var form = document.querySelector('form'); form.addEventListener('submit', function(event) { event.preventDefault(); var username = form.elements['username'].value; var password = form.elements['password'].value; if (username === 'admin' && password === 'admin') { alert('登录成功'); } else { alert('账号或密码错误'); } });
obtains the DOM element of the form through the document.querySelector
method, and then uses the addEventListener
method to provide a callback function for the form's submission event. This callback function will be triggered when the "Login" button is clicked. The event
parameter represents event-related information. Use the event.preventDefault
method to prevent the default behavior, that is, the form submission operation.
Then, use the elements
attribute of the form element to get the value in the input box to determine whether the account password meets the requirements. If the requirements are met, use the alert
function to prompt the user to successfully log in; otherwise, prompt the user that the user account or password is incorrect.
- Data submission
In the actual login operation, the account number and password entered by the user need to be submitted to the back-end service for verification. In JavaScript, you can use the XMLHttpRequest
object to implement AJAX requests to achieve this purpose.
The code is as follows:
var form = document.querySelector('form'); form.addEventListener('submit', function(event) { event.preventDefault(); var username = form.elements['username'].value; var password = form.elements['password'].value; var xhr = new XMLHttpRequest(); xhr.open('POST', '/login'); xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); xhr.onreadystatechange = function() { if (xhr.readyState === 4 && xhr.status === 200) { var response = JSON.parse(xhr.responseText); if (response.code === 0) { alert('登录成功'); } else { alert(response.message); } } }; xhr.send('username=' + encodeURIComponent(username) + '&password=' + encodeURIComponent(password)); });
Among them, the XMLHttpRequest
object is used to send asynchronous HTTP requests. We use POST requests to send the username and password to the backend service as the request body content. The setRequestHeader
method is used to set the request header; the onreadystatechange
method is used to monitor changes in the request status and execute the callback function. When the request is successful and the return result is not empty, parse the result and determine whether the login is successful based on the return value.
- Conclusion
Through the above steps, we implemented a simple login window and used JavaScript code to handle the form submission and data submission functions. This implementation process also demonstrates the basic syntax and DOM operations of JavaScript, and also introduces the basic use of AJAX requests. Although such an implementation is simple, it is the basis of actual projects. On this basis, the login function can be further expanded according to actual needs.
The above is the detailed content of Implementation of login window javascript. For more information, please follow other related articles on the PHP Chinese website!
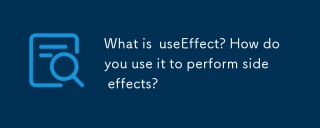
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
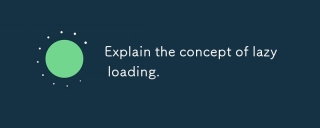
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
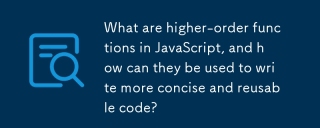
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
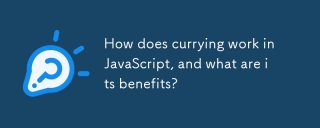
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
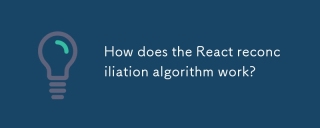
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
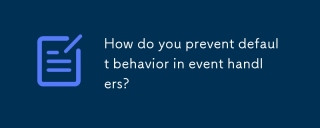
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
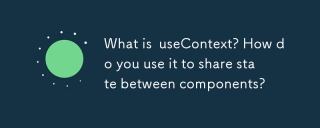
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
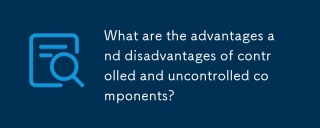
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
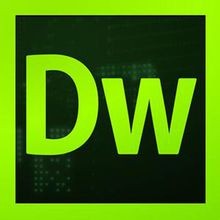
Dreamweaver CS6
Visual web development tools
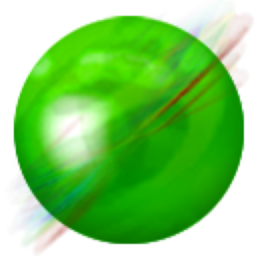
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
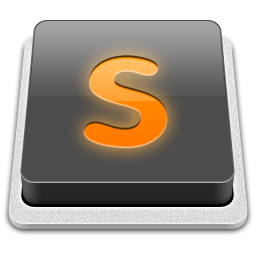
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
