(This article will introduce how to use the ORM framework xorm of the Go language to implement the inversion operation)
xorm is one of the commonly used ORM frameworks in the Go language. It supports many databases, including MySQL, PostgreSQL, SQLite, Oracle, and more. When using xorm for database operations, we often need to perform record reversal operations, that is, reversing records in a table into records in another table. The following will introduce how to use xorm to implement the reversal operation in the Go language.
First, we need to define our data model. Suppose we have a User model, which contains fields such as id, name, email, etc. We need to define two structures, representing the User table and ReverseUser table respectively.
type User struct { Id int64 `xorm:"'id' pk autoincr"` Name string `xorm:"'name'"` Email string `xorm:"'email'"` } type ReverseUser struct { Id int64 `xorm:"'id' pk autoincr"` Name string `xorm:"'name'"` Email string `xorm:"'email'"` Reverse string `xorm:"'reverse'"` }
It should be noted that we have added a new field Reverse in ReverseUser to store the reversed data.
Next, we need to use xorm for database operations. First, define two engines, corresponding to the User table and ReverseUser table:
userEngine, _ := xorm.NewEngine("mysql", "username:password@tcp(127.0.0.1:3306)/user_db?charset=utf8") userEngine.ShowSQL(true) reverseEngine, _ := xorm.NewEngine("mysql", "username:password@tcp(127.0.0.1:3306)/reverse_user_db?charset=utf8") reverseEngine.ShowSQL(true)
It should be noted that the database connection information here needs to be modified according to the actual situation.
Next, we need to convert the records in the User table to records in the ReverseUser table. This can be achieved with the following code:
users := make([]User, 0) err := userEngine.Find(&users) if err != nil { fmt.Println(err) return } reverseUsers := make([]ReverseUser, 0) for _, user := range users { reverseUsers = append(reverseUsers, ReverseUser{ Name: user.Name, Email: user.Email, Reverse: reverseString(user.Name), }) } err = reverseEngine.Insert(&reverseUsers) if err != nil { fmt.Println(err) return }
First, we use userEngine to get all the records from the User table. Then, iterate through each User record, convert it to a ReverseUser record, and add it to the reverseUsers slice. Finally, use reverseEngine to insert the records in reverseUsers into the ReverseUser table.
It should be noted that we store the reverse form of the name in the Reverse field of ReverseUser, which needs to be implemented through a function. The following is the implementation of this function:
func reverseString(str string) string { runes := []rune(str) for i, j := 0, len(runes)-1; i < j; i, j = i+1, j-1 { runes[i], runes[j] = runes[j], runes[i] } return string(runes) }
This function receives a string as a parameter and returns the reversed form of the string.
Finally, we need to do some finishing work and shut down the database engine:
defer userEngine.Close() defer reverseEngine.Close()
The above code implements the complete process of using xorm to perform the reversal operation. It should be noted that this method is suitable for small data tables and is not suitable for large data tables, because maintaining large slices in memory may cause performance problems. If your data table is very large, use appropriate paging techniques.
Summary:
In the Go language, it is very convenient to use the xorm framework to implement data reversal operations. We only need to define the data model, create a database engine, convert the data into the required format, and then insert it into the database. At the same time, we need to pay attention to some details, such as canceling the structure of the table, etc., to ensure that our code works properly.
The above is the detailed content of golang xorm reverse. For more information, please follow other related articles on the PHP Chinese website!
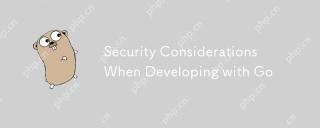
Gooffersrobustfeaturesforsecurecoding,butdevelopersmustimplementsecuritybestpracticeseffectively.1)UseGo'scryptopackageforsecuredatahandling.2)Manageconcurrencywithsynchronizationprimitivestopreventraceconditions.3)SanitizeexternalinputstoavoidSQLinj
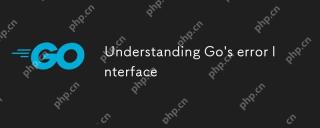
Go's error interface is defined as typeerrorinterface{Error()string}, allowing any type that implements the Error() method to be considered an error. The steps for use are as follows: 1. Basically check and log errors, such as iferr!=nil{log.Printf("Anerroroccurred:%v",err)return}. 2. Create a custom error type to provide more information, such as typeMyErrorstruct{MsgstringDetailstring}. 3. Use error wrappers (since Go1.13) to add context without losing the original error message,
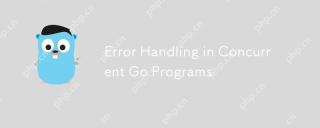
ToeffectivelyhandleerrorsinconcurrentGoprograms,usechannelstocommunicateerrors,implementerrorwatchers,considertimeouts,usebufferedchannels,andprovideclearerrormessages.1)Usechannelstopasserrorsfromgoroutinestothemainfunction.2)Implementanerrorwatcher
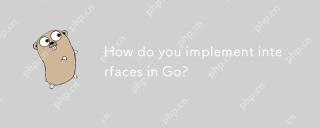
In Go language, the implementation of the interface is performed implicitly. 1) Implicit implementation: As long as the type contains all methods defined by the interface, the interface will be automatically satisfied. 2) Empty interface: All types of interface{} types are implemented, and moderate use can avoid type safety problems. 3) Interface isolation: Design a small but focused interface to improve the maintainability and reusability of the code. 4) Test: The interface helps to unit test by mocking dependencies. 5) Error handling: The error can be handled uniformly through the interface.
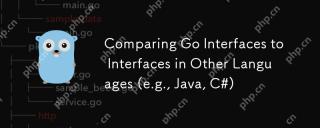
Go'sinterfacesareimplicitlyimplemented,unlikeJavaandC#whichrequireexplicitimplementation.1)InGo,anytypewiththerequiredmethodsautomaticallyimplementsaninterface,promotingsimplicityandflexibility.2)JavaandC#demandexplicitinterfacedeclarations,offeringc
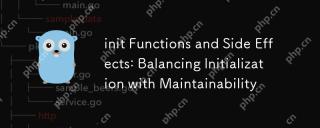
Toensureinitfunctionsareeffectiveandmaintainable:1)Minimizesideeffectsbyreturningvaluesinsteadofmodifyingglobalstate,2)Ensureidempotencytohandlemultiplecallssafely,and3)Breakdowncomplexinitializationintosmaller,focusedfunctionstoenhancemodularityandm
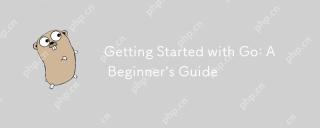
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
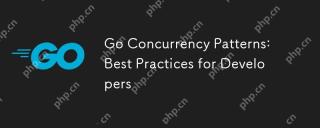
Developers should follow the following best practices: 1. Carefully manage goroutines to prevent resource leakage; 2. Use channels for synchronization, but avoid overuse; 3. Explicitly handle errors in concurrent programs; 4. Understand GOMAXPROCS to optimize performance. These practices are crucial for efficient and robust software development because they ensure effective management of resources, proper synchronization implementation, proper error handling, and performance optimization, thereby improving software efficiency and maintainability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
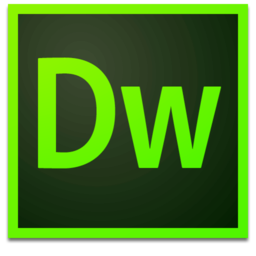
Dreamweaver Mac version
Visual web development tools
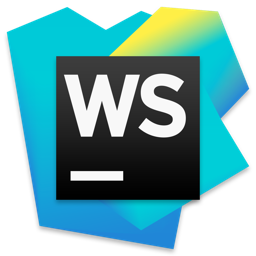
WebStorm Mac version
Useful JavaScript development tools
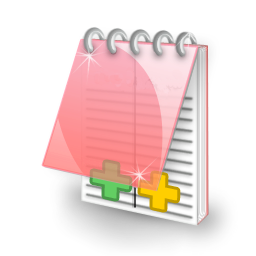
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
