Go language is an open source static compiled language developed by Google, referred to as golang. It is efficient, easy to learn and describe, and can handle large-scale data and concurrency problems, so it is widely used in many fields.
This article will introduce how to use golang to modify excel files. In most business fields and work, we need to deal with excel files frequently, which involves a series of operations such as inserting, deleting, updating and searching data. Therefore, it is very necessary to understand how to use golang to operate excel files.
Step1. Install excelize
There are many libraries available for processing excel files in golang, the most popular of which is excelize. This library can treat an xlsx file as a database, where we can create new data tables, insert new data rows, modify existing data, or add or delete rows or columns in the data.
In order to use the excelize library, we must install it first. You can install the library through the following command:
go get -u github.com/360EntSecGroup-Skylar/excelize
This command will download the excelize library to the $GOPATH/src path. If you haven't set your GOPATH yet, it is recommended to create a go directory in the user directory and add it to the environment variable.
Step2. Create excel file
First we need to create an excel file. This can be achieved by using the Excelize library's NewFile() method. The following is a sample code:
package main import ( "fmt" "github.com/360EntSecGroup-Skylar/excelize" ) func main() { // 创建一个excel文件 f := excelize.NewFile() // 在Sheet1中的A1单元格中设置一个值 f.SetCellValue("Sheet1", "A1", "Hello, world!") // 将数据写入文件并将其保存到本地磁盘 if err := f.SaveAs("test.xlsx"); err != nil { fmt.Println(err) } }
The above code creates an excel file named test.xlsx and writes a "Hello, world!" string to cell A1 of Sheet1. We save the file to the local disk by calling the f.SaveAs() method.
If you want to add a new sheet to an existing excel file, you can use the NewSheet() method. The sample code is as follows:
// 新建一个excel文件 f, err := excelize.OpenFile("test.xlsx") if err != nil { fmt.Println(err) } // 在现有文件中新建一个Sheet f.NewSheet("new sheet") // 在新Sheet的A1单元格中设置一个值 f.SetCellValue("new sheet", "A1", "Hello, world!") // 将数据写入文件并将其保存到本地磁盘 if err := f.SaveAs("test.xlsx"); err != nil { fmt.Println(err) }
Step3. Modify the excel file
Now that we have created an excel file, we can modify it directly. The following is a sample code for modifying an excel file:
// 打开一个excel文件 f, err := excelize.OpenFile("test.xlsx") if err != nil { fmt.Println(err) } // 获取Sheet1中的A1单元格的值 cell := f.GetCellValue("Sheet1", "A1") fmt.Println(cell) // Output: Hello, world! // 在Sheet1中的A2单元格中设置一个值 f.SetCellValue("Sheet1", "A2", "Nice to meet you!") // 在新Sheet上添加一个表格 f.SetCellValue("new sheet", "B2", "Excelize") // 将数据写入到文件中并将其保存到本地磁盘 if err := f.SaveAs("test.xlsx"); err != nil { fmt.Println(err) }
This sample code opens an excel file named test.xlsx. It reads a value from cell A1 of Sheet1 and prints it. Then new data was inserted into cell A2 of Sheet1 and cell B2 of the new sheet.
Step4. Delete excel file
Finally, if you want to delete a certain cell, row or column from the excel file, we can use DeleteCell(), DeleteRow() and DeleteCol( )method. The following is a sample code to delete cells, rows and columns:
// 打开一个excel文件 f, err := excelize.OpenFile("test.xlsx") if err != nil { fmt.Println(err) } // 删除Sheet1中的A2单元格 f.DeleteCell("Sheet1", "A2") // 删除Sheet1中的第二行 f.DeleteRow("Sheet1", 2) // 删除Sheet1中的第二列 f.DeleteCol("Sheet1", "B") // 将数据写入到文件中并将其保存到本地磁盘 if err := f.SaveAs("test.xlsx"); err != nil { fmt.Println(err) }
With these commands, we can easily delete excel files.
Conclusion
We now know how to use golang to operate excel files. In actual projects, these skills are of practical significance. From reading to modifying to deleting, we have introduced many ways to process excel files through this article. I believe that through the explanation of this article, readers can master the skills of using golang to operate excel files and apply them in actual development.
The above is the detailed content of golang modify excel. For more information, please follow other related articles on the PHP Chinese website!
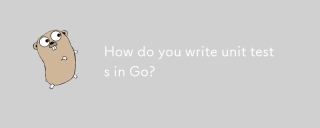
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
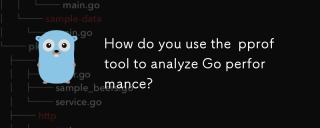
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
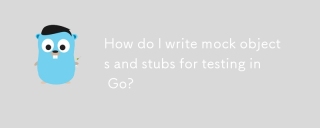
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
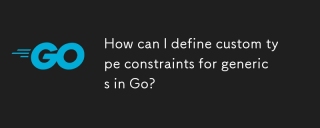
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
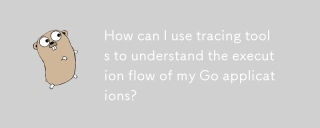
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
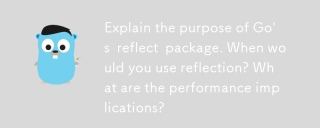
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
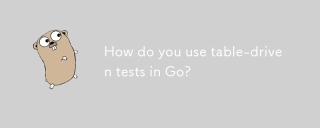
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
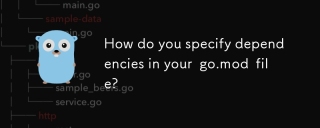
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
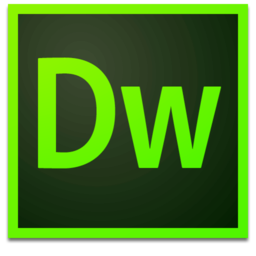
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
