1. Asynchronous streams
One of the main benefits of asyncio is the ability to use non-blocking streams.
Asyncio provides non-blocking I/O socket programming. This is provided via streaming.
Can open sockets that provide access to stream writers and stream writers. Use coroutines to write and read data from the stream and pause when appropriate. Once completed, the socket can be closed.
The asynchronous streaming functionality is low-level, which means that any protocols required must be implemented manually.
This may include common web protocols such as:
HTTP or HTTPS for interacting with the web server
SMTP for interacting with email servers
FTP for interacting with file servers.
These streams can also be used to create servers to handle requests using standard protocols, or to develop your own application-specific protocols.
Now that we know what asynchronous streams are, let’s see how to use them.
2. How to open the connection
You can use the asyncio.open_connection() function to open the asyncio TCP client socket connection.
This is a coroutine that must wait and return once the socket connection is opened.
This function returns the StreamReader and StreamWriter objects used to interact with the socket.
... # open a connection reader, writer = await asyncio.open_connection(...)
There are multiple parameters that can be used to configure the socket connection in the asyncio.open_connection() function.. The two required parameters are host and port.
host is a string specifying the server to connect to, such as a domain name or IP address.
port is the socket port number, such as 80 for HTTP server, 443 for HTTPS server, 23 for SMTP, etc.
... # open a connection to an http server reader, writer = await asyncio.open_connection('www.google.com', 80)
Supports encrypted socket connections via SSL protocol. Perhaps the most common example is HTTPS, which is replacing HTTP. This can be achieved by setting the "ssl" parameter to True.
... # open a connection to an https server reader, writer = await asyncio.open_connection('www.google.com', 443, ssl=True)
3. How to start the server
You can use the asyncio.start_server() function to open the asyncio TCP server socket. This is a coroutine that must wait.
This function returns an asyncio.Server object representing the running server.
... # start a tcp server server = await asyncio.start_server(...)
The three required parameters are the callback function, host and port. When the client connects to the server, the callback function is called, which is a named custom function.
The host is the domain name or IP address that the client will specify to connect to. The port used by FTP is 21 and the port used by HTTP is 80. These ports are the socket port numbers used to receive connections.
# handle connections async def handler(reader, writer): # ... ... # start a server to receive http connections server = await asyncio.start_server(handler, '127.0.0.1', 80)
4. How to use StreamWriter to write data
You can use asyncio.StreamWriter to transfer data to the socket. Data is written in bytes. Byte data can be written to a socket using the write() method.
... # write byte data writer.write(byte_data)
Alternatively, the writelines() method can be used to write multiple "lines" of byte data organized into a list or iterable.
... # write lines of byte data writer.writelines(byte_lines)
There are no methods to write data blocks or suspend calling coroutines. After writing byte data, it is best to empty the socket via the drain() method. This is a coroutine that will cause the caller to pause until the data is transferred and the socket is ready.
... # write byte data writer.write(byte_data) # wait for data to be transmitted await writer.drain()
5. How to use StreamReader to read data
Use asyncio.StreamReader to read data in the socket. The data is read in bytes format, so strings may need to be encoded before use. All read methods are coroutines that must wait.
You can read any number of bytes through the read() method, which will read until the end of file (EOF).
... # read byte data byte_data = await reader.read()
Additionally, the number of bytes to read can be specified via the "n" parameter. It might help if you know the expected number of bytes for the next response.
... # read byte data byte_data = await reader.read(n=100)
You can use the readline() method to read a single line of data. This will return bytes until a newline character "\n" or EOF is encountered.
This is useful when reading standard protocols that operate with lines of text.
... # read a line data byte_line = await reader.readline()
Additionally, there is a readexactly() method to read the exact number of bytes, otherwise an exception will be thrown, and a readuntil() method that will read bytes until the bytes are read Specify characters.
6. How to close the connection
You can use the asyncio.StreamWriter object to close the network socket. The socket can be closed by calling the close() method. This method does not block.
... # close the socket writer.close()
Although the close() method is non-blocking, we can wait for the socket to be completely closed before continuing. This can be achieved through the wait_closed() method. This is a coroutine that can be awaited.
... # close the socket writer.close() # wait for the socket to close await writer.wait_closed()
We can check whether the socket has been closed or is being closed through the is_closing() method.
... # check if the socket is closed or closing if writer.is_closing(): # ...
The above is the detailed content of How to use Python asynchronous non-blocking streams. For more information, please follow other related articles on the PHP Chinese website!
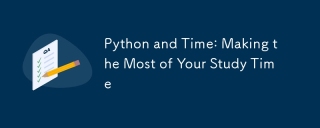
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
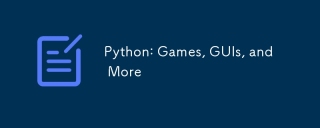
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
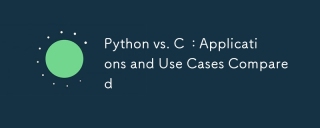
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
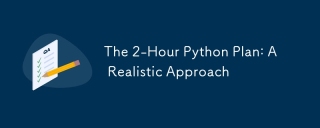
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
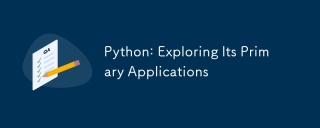
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
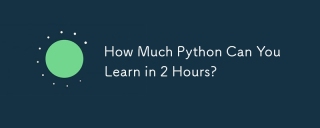
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
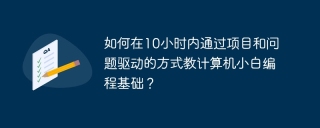
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
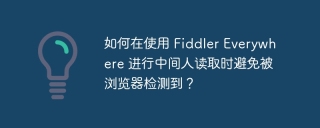
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
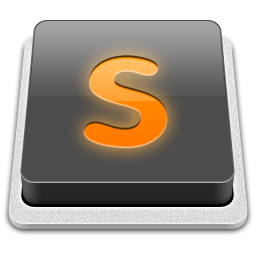
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
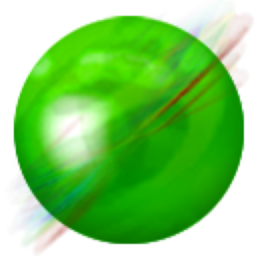
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment