As modern web applications continue to evolve, authentication and security are becoming increasingly important. One of the popular methods is to use JWT (JSON Web Tokens) tokens for authentication. This article will introduce you to the basics and techniques of implementing JWT tokens using PHP.
- JWT Token Overview
JWT is an open standard that defines a way to securely and efficiently transmit claims by transmitting JSON objects over the network. JWT consists of three parts: header, payload and signature. The header contains the type of token and the hash algorithm used, the payload contains authentication and authorization information, and the signature is a string combining the header and payload hashes and key.
JWT supports stateless, server-side authentication, avoiding the need to use server sessions or cookies. And it's easy to extend because it's a standardized JSON format.
- PHP implementation process
The PHP language provides many classes that can easily generate and verify JWT tags. Creating JWT in PHP requires the use of a library, we use the php-jwt library, which is available for free on GitHub.
Install the php-jwt library:
composer require firebase/php-jwt
Creating a JWT token is simple, here is the sample code:
require_once('vendor/autoload.php'); use FirebaseJWTJWT; $key = "example_key"; $payload = array( "iss" => "http://example.org", "aud" => "http://example.com", "iat" => 1356999524, "nbf" => 1357000000 ); $jwt = JWT::encode($payload, $key); print_r($jwt);
In this example , we use a key example_key
and some clues, such as the issuer of the token iss
, and the recipients who can use the token aud
. We also define the life cycle of the JWT, that is, it should be usable after the token issuance time iat
and before the current time point nbf
.
The final result of JWT is a long string in the form of AAA.BBB.CCC. AAA is the header, BBB is the payload, and CCC is the signature, which is generated by the key.
Verification of the JWT token requires the use of the same key, the sample code is as follows:
require_once('vendor/autoload.php'); use FirebaseJWTJWT; $key = "example_key"; $jwt = "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpc3MiOiJodHRwOi8vZXhhbXBsZS5vcmciLCJhdWQiOiJodHRwOi8vZXhhbXBsZS5jb20iLCJpYXQiOjEzNTY5OTk1MjQsIm5iZiI6MTM1NzAwMDAwMCwiZXhwIjoxMzU3MDAwMDAwfQ.kn4N5lvvvdQhL7rEixJOaYWKQZ3GzrCc8REIzc2Kw8c"; $decoded = JWT::decode($jwt, $key, array('HS256')); print_r($decoded);
If the token can be successfully decoded, then your code will output the same associative array as in the payload. If authentication fails, an exception will be thrown.
- Conclusion
This article explains how to implement JWT tokens using PHP. JWT is a simple but useful tool that can make your applications more secure and avoid using traditional session mechanisms. We hope this article helped you better understand JWT and how to use it in PHP applications.
The above is the detailed content of Getting Started with PHP: JWT Tokens. For more information, please follow other related articles on the PHP Chinese website!
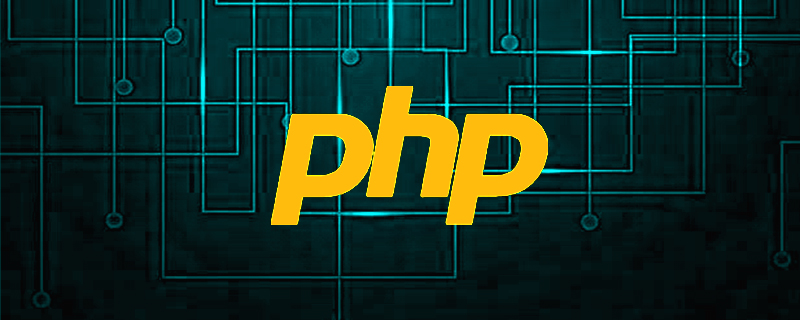
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
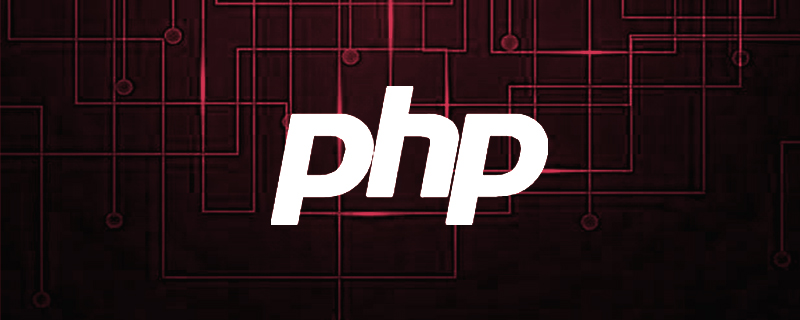
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
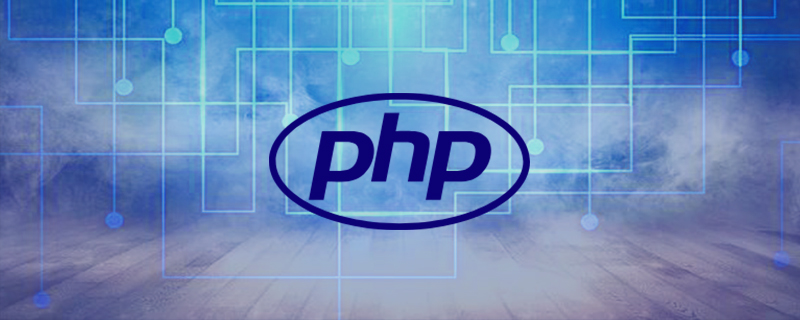
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
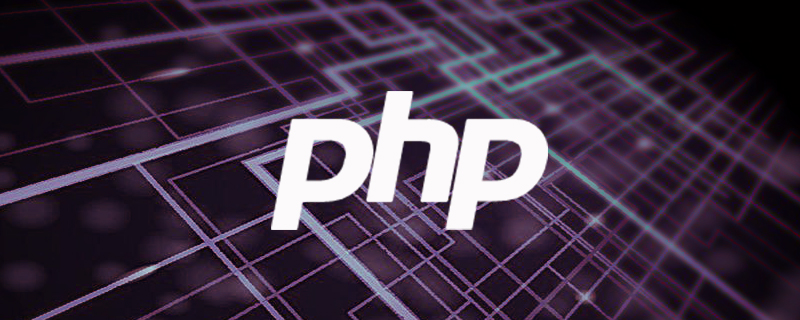
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
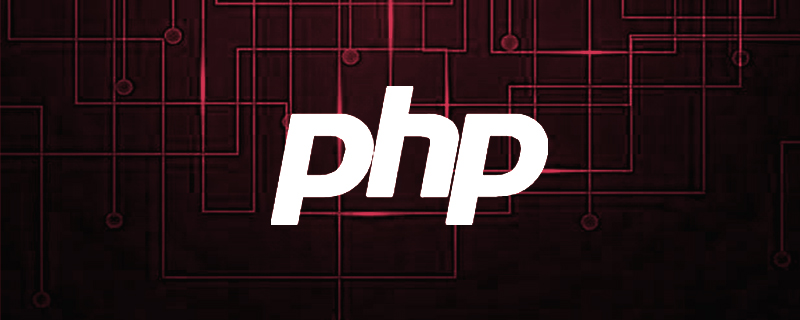
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
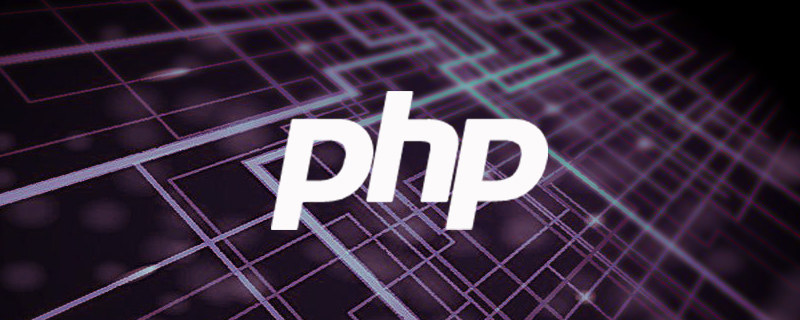
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
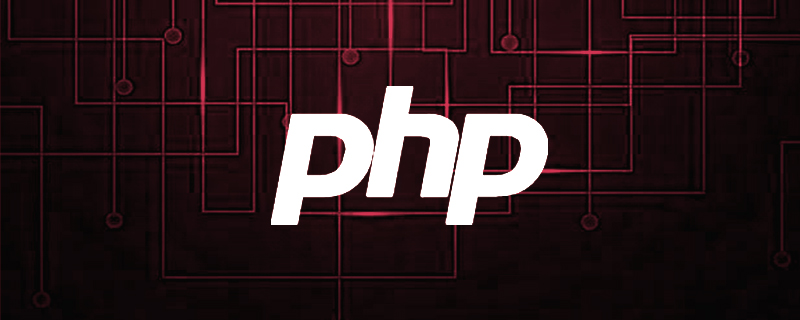
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
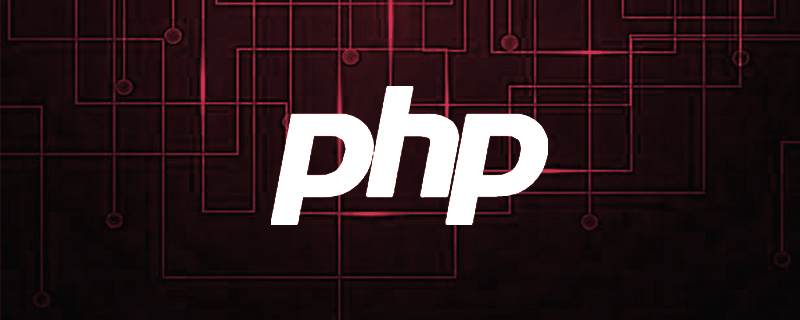
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
