Golang function tcp, udp, http and websocket processing methods
With the continuous development of Internet technology, network communication has become an essential modern skill. In this process, function (Function), as an efficient and flexible programming method, has become a tool favored by more and more programmers. Among these functions, the processing methods based on TCP, UDP, HTTP and WebSocket protocols are particularly important. Today, this article will focus on the Golang function processing methods under these four protocols.
1. Function processing method based on TCP protocol
TCP (Transmission Control Protocol) is a connection-oriented, reliable, byte stream-based transport layer protocol. In Golang, we can create a simple TCP server through the following code:
package main import ( "fmt" "net" ) func main() { listener, err := net.Listen("tcp", "127.0.0.1:8080") if err != nil { fmt.Println("Error listening:", err.Error()) return } for { conn, err := listener.Accept() if err != nil { fmt.Println("Error accepting:", err.Error()) return } go handleRequest(conn) } } func handleRequest(conn net.Conn) { buf := make([]byte, 1024) for { _, err := conn.Read(buf) if err != nil { fmt.Println("Error reading:", err.Error()) return } conn.Write([]byte("Hello, world!")) } }
In the above code, we use the net package to create a TCP listener, and then use the Accept() method to wait for the client terminal connection. Next, we handle the connection in the handleRequest() function and send our response in the connection.
2. Function processing method based on UDP protocol
UDP (User Datagram Protocol) is a non-connection and unreliable transport layer protocol. In Golang, we can also easily create a simple UDP server, the code is as follows:
package main import ( "fmt" "net" ) func main() { udpAddr, err := net.ResolveUDPAddr("udp", "127.0.0.1:8080") if err != nil { fmt.Println("Error Resolving UDP address:", err.Error()) return } conn, err := net.ListenUDP("udp", udpAddr) if err != nil { fmt.Println("Error Listening:", err.Error()) return } defer conn.Close() for { buf := make([]byte, 1024) _, remoteAddr, err := conn.ReadFromUDP(buf) if err != nil { fmt.Println("Error Reading:", err.Error()) continue } conn.WriteToUDP([]byte("Hello, world!"), remoteAddr) } }
3. Function processing method based on HTTP protocol
HTTP (Hypertext Transfer Protocol) is An application layer protocol used to transfer data between web browsers and web servers. In Golang, we can use the net/http package in the standard library to create a simple HTTP server. The code is as follows:
package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, world!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
In the above code, we define a handler() function to handle HTTP request, and use http.HandleFunc() in the main() function to specify our handler. Finally, we use the http.ListenAndServe() method to start our server.
4. Function processing method based on WebSocket protocol
WebSocket is a full-duplex protocol, usually used to implement instant communication functions, such as online games, instant chat, etc. In Golang, we can use the gorilla/websocket package to create a WebSocket server. The code is as follows:
package main import ( "net/http" "github.com/gorilla/websocket" ) var upgrader = websocket.Upgrader{ ReadBufferSize: 1024, WriteBufferSize: 1024, } func handler(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { return } defer conn.Close() for { _, message, err := conn.ReadMessage() if err != nil { return } conn.WriteMessage(websocket.TextMessage, []byte("Hello, world!")) } } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
In the above code, we use the gorilla/websocket package to create a WebSocket server and define handler( ) function to handle WebSocket connections. Finally, we use the http.HandleFunc() and http.ListenAndServe() methods to start our server.
Summary
Whether it is TCP, UDP, HTTP or WebSocket protocol, Golang provides a rich function library to help us quickly build servers and handle various network communications. By learning the above four protocols and Golang's processing methods, I believe you will be able to better understand functional programming and be able to use Golang more flexibly to implement various network applications.
The above is the detailed content of Golang function tcp, udp, http and websocket processing methods. For more information, please follow other related articles on the PHP Chinese website!
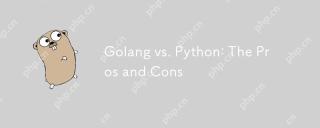
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
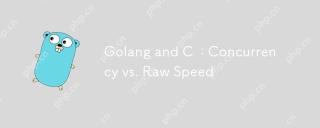
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
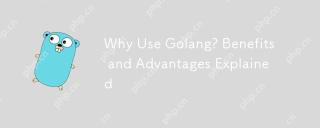
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
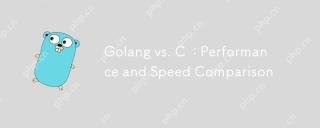
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
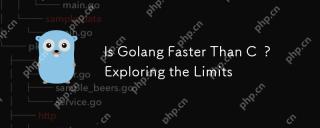
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
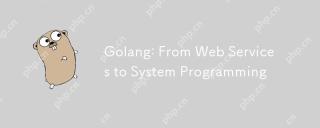
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
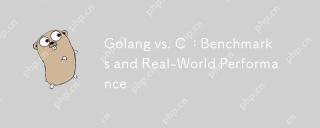
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
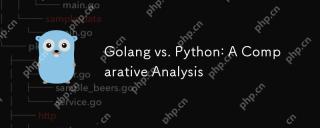
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
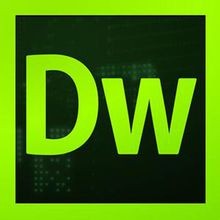
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
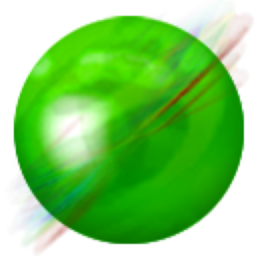
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment