PHP is a scripting language widely used in Web development. It has a huge community and rich resources, and can easily realize the development of dynamic Web pages and data interaction. However, in actual development, it may be necessary to remotely call other services or extend the PHP module to achieve more functions and performance optimization. This article will introduce how to make remote calls and module extensions in PHP.
1. PHP remote calling
In actual development, we may need to make remote calls to other services, such as calling the API of other Web services, calling remote databases, etc. At this time we can use the curl extension or stream extension provided by PHP to implement remote calls.
- curl extension
curl is a powerful open source tool that can be used to interact with file transfer protocols and supports HTTP/HTTPS/FTP and other protocols. PHP provides the curl extension, which allows us to easily implement remote calls to other services through PHP code. The following is a simple curl call example:
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'http://example.com/api'); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); print_r($response);
In the above code example, a curl session is first initialized through the curl_init() function, and then the URL to be requested, whether a return value is required, etc. are set through the curl_setopt() function. parameters, and finally execute the request through the curl_exec() function and close the curl session with the curl_close() function. Print the returned results through the print_r() function.
- stream extension
The stream extension provides a set of PHP functions that allow us to operate various streams in a unified way, including files, network connections, etc. You can use the stream_context_create() function to create a context, and then use it as the third parameter of the stream_socket_client() function to implement remote calls to other services. The following is a simple stream call example:
$ctx = stream_context_create([ 'http' => [ 'method' => 'POST', 'header' => 'Content-type: application/json', 'content' => json_encode(['name' => 'John', 'age' => 25]), ], ]); $fp = stream_socket_client('http://example.com/api', $err, $errstr, 30, STREAM_CLIENT_CONNECT, $ctx); $response = stream_get_contents($fp); fclose($fp); print_r($response);
In the above code example, a context is first created through the stream_context_create() function, in which the request method, request header, request body and other parameters are set. Then establish a network connection to the specified service through the stream_socket_client() function, and pass in the context as the third parameter. Finally, obtain the response content through the stream_get_contents() function and close the network connection.
2. PHP module extension
A PHP module is a set of functions or classes that can extend the functions of PHP and may bring improvements in performance, security, etc. The following are some commonly used and easily extensible PHP modules.
- Redis
Redis is an open source, high-performance, key-value data storage system that can be used for caching and data storage. PHP provides a redis extension that makes it easy to interact with Redis using PHP. The following is a simple redis operation example:
$redis = new Redis(); $redis->connect('127.0.0.1', 6379); $redis->set('name', 'Bob'); echo $redis->get('name');
In the above code example, a Redis object is first created through the new keyword and connected to the Redis server through the connect() function. Then set a key-value pair through the set() function, and then obtain the corresponding value through the get() function.
- PDO
PDO (PHP Data Objects) is a data access abstraction layer provided by PHP that can be used to interact with various databases. PDO provides a unified set of interfaces, supports functions such as preprocessing and precompiled statements, and has good security and code reusability. The following is a simple example of PDO connection to MySQL:
$dbh = new PDO('mysql:host=localhost;dbname=test', 'username', 'password'); $stmt = $dbh->prepare('SELECT * FROM users WHERE id = ?'); $stmt->bindParam(1, $id); $stmt->execute(); $result = $stmt->fetchAll(PDO::FETCH_ASSOC); print_r($result);
In the above code example, a PDO object is first created through the new keyword and the MySQL connection parameters are passed in. Then create a prepared statement through the prepare() function, using placeholders? , indicating that a parameter will be passed in later using the bindParam() function. Finally, the precompiled statement is executed through the execute() function, and the query results are obtained through the fetchAll() function.
3. Conclusion
This article introduces how to perform remote calls and module extensions in PHP, including using curl and stream extensions to implement remote calls, and using redis and PDO extensions for data storage and access. The above examples are only the simplest usage, and the specific implementation can be flexibly adjusted according to actual needs. I hope this article can help PHP developers better cope with various development tasks.
The above is the detailed content of How to perform remote calling and module expansion in PHP?. For more information, please follow other related articles on the PHP Chinese website!
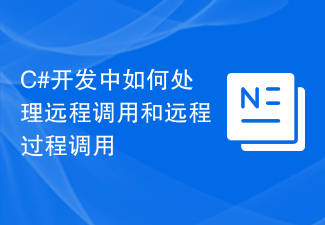
C#开发中如何处理远程调用和远程过程调用,需要具体代码示例引言:随着云计算和分布式系统的快速发展,远程调用和远程过程调用(RemoteProcedureCall,简称RPC)在软件开发中变得越来越重要。C#作为一种强大的编程语言,在处理远程调用和RPC方面也提供了一些强大的工具和框架。本文将给出一些关于如何处理远程调用和RPC的实用代码示例。一、远程调用
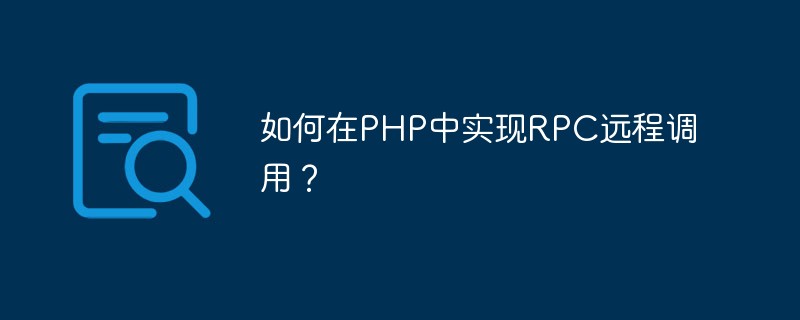
随着互联网的快速发展和云计算技术的广泛应用,分布式系统和微服务架构变得越来越普遍。在这样的背景下,远程过程调用(RPC)成为了一种常见的技术手段。RPC能够使得不同的服务在网络上实现远程调用,从而实现不同服务之间的互联操作,提高代码的复用性和可伸缩性。PHP作为一种广泛应用的Web开发语言,也很常用于各种分布式系统的开发。那么,如何在PHP中实现RPC远程调
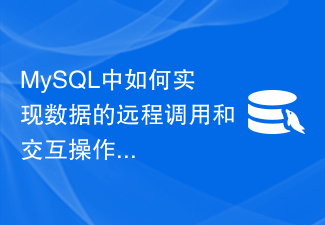
MySQL是一种关系型数据库管理系统,广泛应用于各种软件开发和数据管理场景中。它的一个重要特性是可以实现数据的远程调用和交互操作,本文将介绍如何在MySQL中实现这一功能,并提供相应的代码示例。MySQL提供了一种称为MySQL远程连接的功能,允许在不同的机器之间进行数据交互。为了实现远程连接,我们需要进行以下几个步骤:配置MySQL服务器首先,我们需要确保
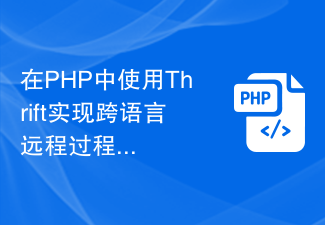
随着应用程序变得越来越复杂和分布式,跨语言远程过程调用(RPC)和通信变得越来越重要。在软件开发中,RPC是指通过网络使不同的程序或进程之间相互通信的技术。Thrift是一种简单易用的RPC框架,它能帮助我们快速开发高效的跨语言RPC服务。Thrift是由Facebook开发的,是一种高效的远程服务调用协议。它支持多种语言,包括PHP、Java、Python
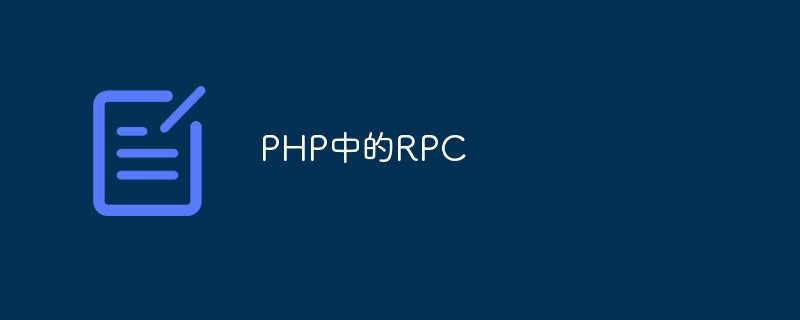
近年来,随着互联网技术的迅猛发展,分布式系统逐渐成为了互联网应用领域中不可缺少的部分。而分布式系统中的RPC技术则是实现不同进程、不同机器之间通讯的重要手段之一。其中,PHP中的RPC技术也逐渐成为了各大互联网企业中使用最为广泛的技术之一。RPC(RemoteProcedureCall)是指远程过程调用,即在不同的进程或不同的机器上,通过远程调用的方式实
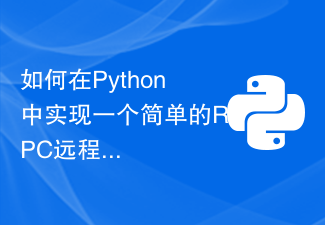
如何在Python中实现一个简单的RPC远程过程调用框架在分布式系统中,一种常见的通信机制是通过RPC(RemoteProcedureCall,远程过程调用)来实现不同进程之间的函数调用。RPC允许开发者像调用本地函数一样调用远程函数,使得分布式系统开发更加方便。本文将介绍如何使用Python实现一个简单的RPC框架,并提供详细的代码示例。1.定义RPC
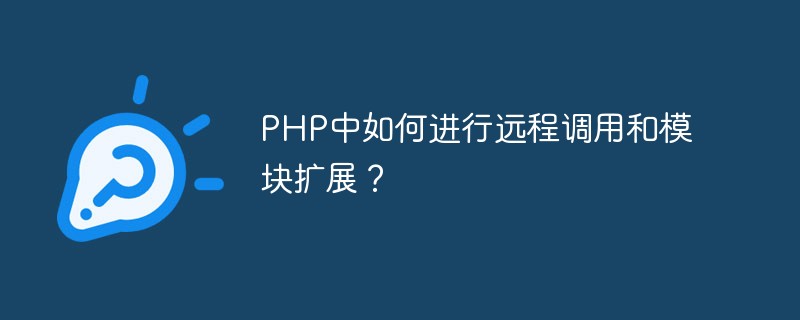
PHP是一门广泛应用于Web开发的脚本语言,拥有庞大的社区和丰富的资源,能够轻松实现动态Web页面的开发和数据交互。但在实际开发中,可能需要对其他服务进行远程调用或扩展PHP模块以实现更多的功能和性能优化。本文将介绍如何在PHP中进行远程调用和模块扩展。一、PHP远程调用在实际开发中,我们可能需要对其他服务进行远程调用,例如调用其他Web服务的API,调用远
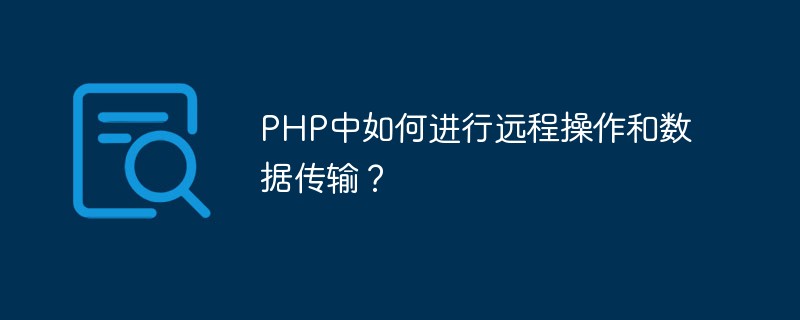
PHP是一种广泛运用的编程语言,主要用于编写Web应用程序。在Web应用程序中,PHP通常要调用远程API接口,访问远程数据库等,因此需要进行远程操作和数据传输。本文将详细介绍PHP中进行远程操作和数据传输的相关知识,包括curl、fsockopen、file_get_contents等常用方法。一、使用curl进行远程操作curl是PHP中非常常用的进行远


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
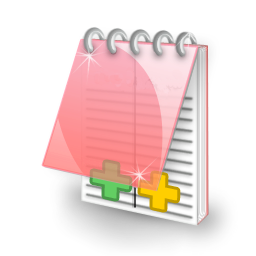
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
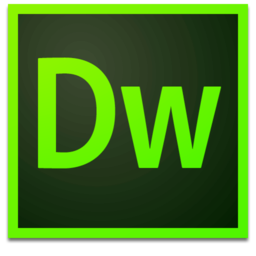
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
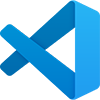
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
