annotation is similar to @Data. By default, all member variables are defined as private final modifications and no set method is generated. |
|
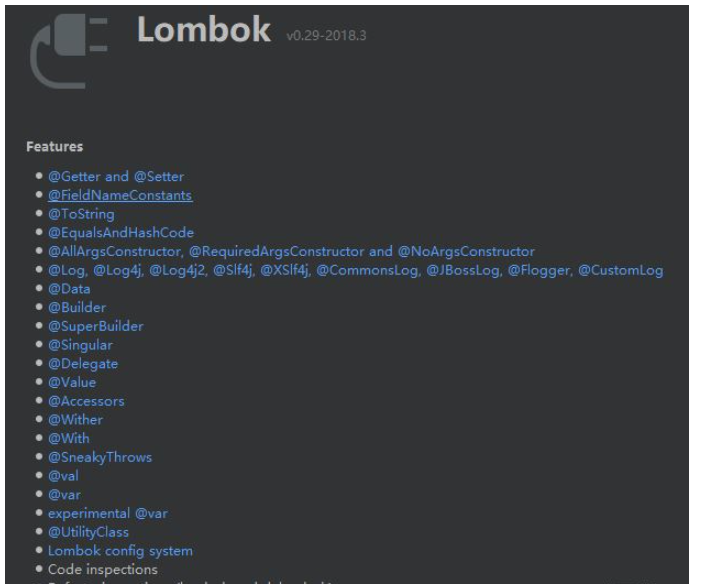
2. Reason for Lombok annotation failure
After introducing Lombok dependency in pom.xml, you also need toinstall the Lombok plug-in,restart IDEA can take effect.
Integration process
1. Introduce Lombok dependencies:
Copy the following code and insert it into pom.xml, wait for the maven warehouse to automatically download and install the dependencies, and there is no automatic package import click Import manually.
<!--导入lombok小辣椒驱动依赖,用来生成get/set等方法依赖-->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<!--<optional>true</optional>-->
<version>1.18.12</version>
<scope>provided</scope><!--自动生成有参无参构造-->
</dependency>
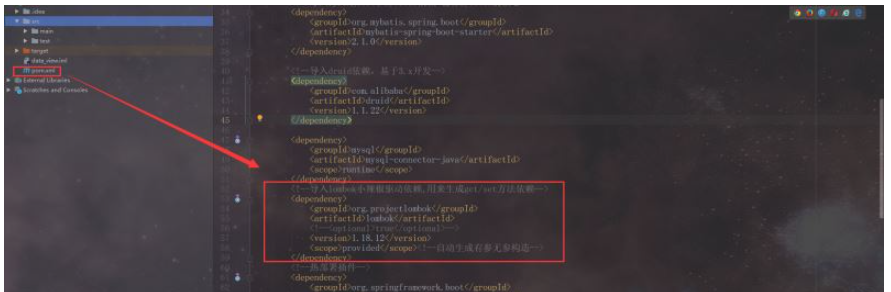
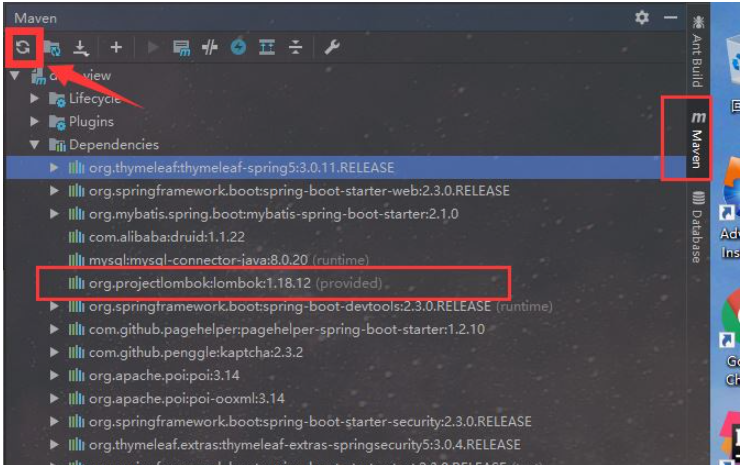
2. Install the Lombok plug-in
Click File-》Setting-》Plugins-》Search in IDEA After Lombok installs the plug-in, restart IDEA;
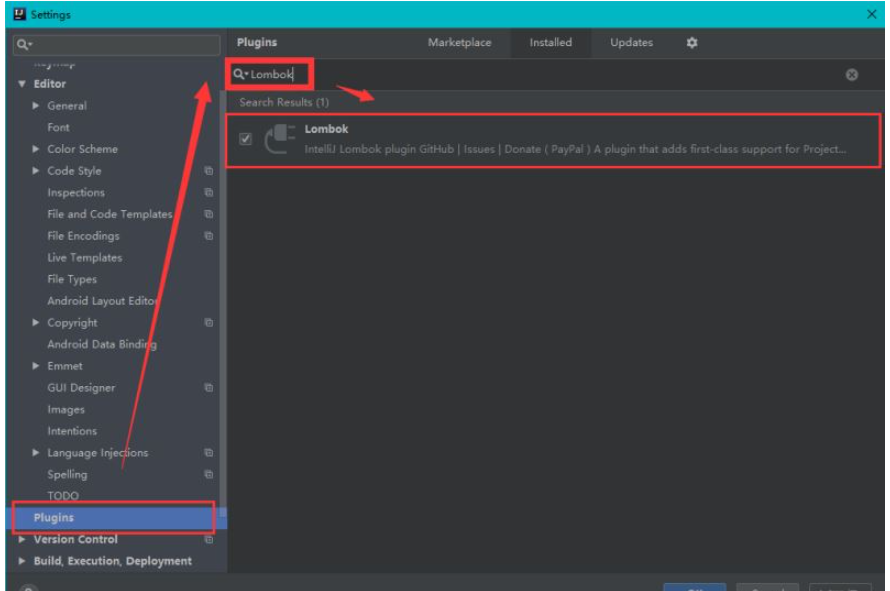
3. Use Lombok to generate getter/setter and other method program code examples for properties
a. Not written using Lombok Entity class (the program is fat and ugly)
Use the shortcut keys that come with the IDE to automatically generate getter/setter methods:
##
package com.dvms.entity;
/*
*文件名: Anglerecord
*创建者: CJW
*创建时间:2020/6/6 14:40
*描述: 记录
*/
public class Record {
private String time;
private String device;
private String state;
public Record(String time, String device, String state) {
this.time = time;
this.device = device;
this.state = state;
}
public Record() {
}
public String getTime() {
return this.time;
}
public String getDevice() {
return this.device;
}
public String getState() {
return this.state;
}
public Record setTime(String time) {
this.time = time;
return this;
}
public Record setDevice(String device) {
this.device = device;
return this;
}
public Record setState(String state) {
this.state = state;
return this;
}
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Record)) return false;
final Record other = (Record) o;
if (!other.canEqual((Object) this)) return false;
final Object this$time = this.getTime();
final Object other$time = other.getTime();
if (this$time == null ? other$time != null : !this$time.equals(other$time)) return false;
final Object this$device = this.getDevice();
final Object other$device = other.getDevice();
if (this$device == null ? other$device != null : !this$device.equals(other$device)) return false;
final Object this$state = this.getState();
final Object other$state = other.getState();
if (this$state == null ? other$state != null : !this$state.equals(other$state)) return false;
return true;
}
protected boolean canEqual(final Object other) {
return other instanceof Record;
}
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $time = this.getTime();
result = result * PRIME + ($time == null ? 43 : $time.hashCode());
final Object $device = this.getDevice();
result = result * PRIME + ($device == null ? 43 : $device.hashCode());
final Object $state = this.getState();
result = result * PRIME + ($state == null ? 43 : $state.hashCode());
return result;
}
public String toString() {
return "Record(time=" + this.getTime() + ", device=" + this.getDevice() + ", state=" + this.getState() + ")";
}
}
b. Introduction Lombok generation method (the program is slim and looks very comfortable)You can manually add annotations according to your needs, or you can right-click-》Refactor-》Lomok-》
package com.dvms.entity;
/*
*文件名: Anglerecord
*创建者: CJW
*创建时间:2020/6/6 14:40
*描述: 记录
*/
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import lombok.ToString;
import lombok.experimental.Accessors;
@Data
@ToString
@AllArgsConstructor
@NoArgsConstructor
@Accessors(chain = true) //链式调用
public class Record {
private String time;
private String device;
private String state;
}
Advantages and disadvantages (possible problems are solutions)Advantages: Lombok can automatically generate getter/setter, equals, toString and other methods for properties at compile time through annotations. , eliminating the trouble of manually rebuilding these codes, making the program entity class (entity/pojo) code look more "slim" and more stylish.
Disadvantages (possible problems): If it is personal development, the following problems may occur:
1.Lombok currently supports JDK1.8, which may become invalid after upgrading the JDK version. Solve Method: a. Use the Alt Insert shortcut key that comes with the IDE to generate getter/setter, equals, hashCode, toString and constructor methods;
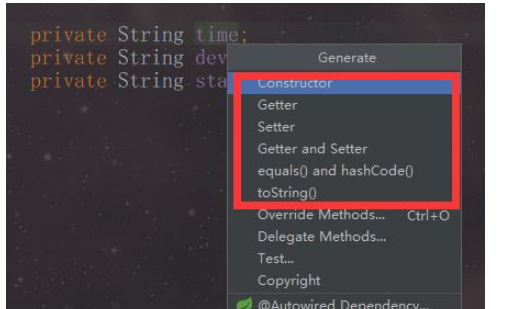
In the latest version DeLombok can be used to generate these methods using this tool. Right-click and select Refactored->DeLombok:
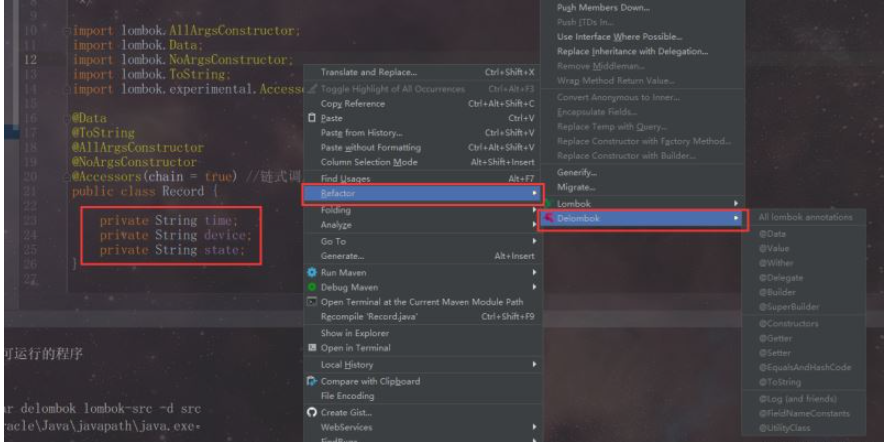
Or use a command:java -jar lombok.jar delombok src -d src-delomboked
Convert the class file implemented by Lombok annotation to a Java source that does not use Lombok document. Using Lombok can simplify JavaBean encapsulation, but it will affect readability. In addition, try not to use this annotation @AllArgsConstructor. This annotation provides a giant constructor, which gives the outside world the opportunity to modify all the properties in the class when initializing the object. It is very unsafe. After all, some properties of objects in Java classes should not be Revise. At the same time, if there are multiple properties in a Java class, Lombok will inject multiple parameter constructors into the Java class, and the order of the constructor parameters is completely controlled by Lombok. 3. After using Lombok to write Javabean code, other codes that rely on this javabean need to introduce Lombok dependencies, and the code coupling degree increases. At the same time, you also need to install the Lombok plug-in in the IDE. 4. If it is collaborative development, you need to pay attention to the following issues: When the Lombok plug-in is introduced into our program code, other people must also download and introduce the Lombok plug-in, otherwise the Java code may not work properly run.