In PHP, accumulation is a common operation and is often used in arrays. Array accumulation can be used to count the sum or count of certain data items, etc. Due to the flexibility of PHP arrays, it provides multiple ways to implement accumulation. This article will introduce several common PHP array accumulation methods.
Method 1: Use a loop
In PHP, you can use a for loop to iterate through each element in the array and then add them. The following is a sample code that uses a for loop to implement array accumulation:
$numbers = array(1, 2, 3, 4, 5); $total = 0; for($i=0;$i<count($numbers);$i++){ $total += $numbers[$i]; } echo '数组元素之和为:' . $total;
In the above code, we first define an array named numbers
and initialize its elements. Then, we define a total
variable to store the accumulated value of the array elements. Use a for loop to iterate through the entire array and add each element of it to the total
variable. Finally, we output the value of the total
variable, which is the sum of all elements in the array.
Method 2: Use the array_sum() function
PHP provides a array_sum()
function that can add all the elements in the array and return their sum. Using this function, you can complete the array accumulation operation with one line of code.
$numbers = array(1, 2, 3, 4, 5); $total = array_sum($numbers); echo '数组总和为:' . $total;
In the above code, we first define an array named numbers
and initialize its elements. Then, we use the array_sum()
function to add all the elements in the array, and we will get their sum, which is saved in the total
variable. Finally, we output the value of the total
variable.
Method 3: Use foreach loop
foreach
Loop is a powerful tool for traversing arrays. Using a foreach
loop, you can get the value of each element in the array and add them together. The following is a sample code for using the foreach
loop to implement array accumulation:
$numbers = array(1, 2, 3, 4, 5); $total = 0; foreach($numbers as $number){ $total += $number; } echo '数组总和为:' . $total;
Similar to using the for
loop, we first define an array named numbers
's array and initializes its elements. Then, we define a total
variable to store the accumulated value of the array elements. Use foreach
to loop through the entire array and add each element to the total
variable. Finally, we output the value of the total
variable, which is the sum of all elements in the array.
Summary
This article introduces three common array accumulation methods in PHP, including loops, array_sum()
functions and foreach
loops. Each accumulation method has its advantages and disadvantages, and the programmer can choose any of them to implement the array accumulation operation. However, in practice, the most appropriate method needs to be chosen based on the specific tasks and needs. If you need to accumulate the number of elements in the array, you can use the count()
function or the sizeof()
function to get the number of elements.
The above is the detailed content of How to accumulate arrays in php. For more information, please follow other related articles on the PHP Chinese website!
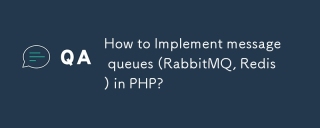
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
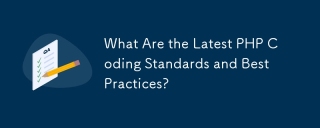
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
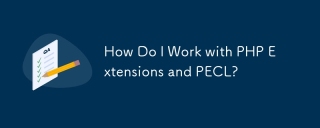
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
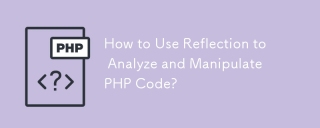
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
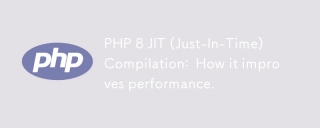
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
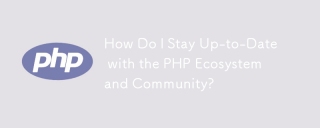
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
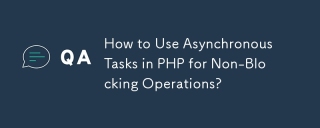
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
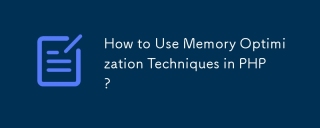
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
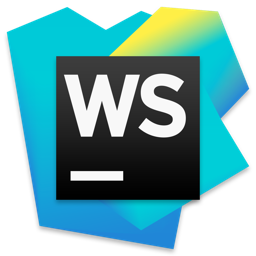
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
