Two-dimensional array is a nested data structure, which consists of multiple one-dimensional arrays. In PHP, adding two-dimensional array elements requires using one or more array functions. This article will introduce how to add two-dimensional array elements.
- Use the array_push function
The array_push function is a function provided by PHP that adds elements to the end of an array. You can use it to add new elements to a two-dimensional array. The specific method is as follows:
<?php $fruits = array( array("apple", 50), array("banana", 30), array("orange", 20), ); $fruits_new = array("grape", 40); array_push($fruits, $fruits_new); print_r($fruits); ?>
In the above code, we first define a two-dimensional array $fruits, which contains three one-dimensional arrays, each one-dimensional array contains the name and price of the fruit. We then define the new element we want to add as a one-dimensional array $fruits_new and use the array_push function to add it to the end of the $fruits array. Finally, use the print_r function to output the $fruits array.
The output result is as follows:
Array ( [0] => Array ( [0] => apple [1] => 50 ) [1] => Array ( [0] => banana [1] => 30 ) [2] => Array ( [0] => orange [1] => 20 ) [3] => Array ( [0] => grape [1] => 40 ) )
You can see that the new element has been successfully added to the two-dimensional array.
- Direct assignment
We can also directly assign new elements to the two-dimensional array. The specific method is as follows:
<?php $fruits = array( array("apple", 50), array("banana", 30), array("orange", 20), ); $fruits[] = array("grape", 40); print_r($fruits); ?>
In the above code, We define the new element we want to add as a one-dimensional array and add it to the end of the $fruits array using the [] operator. Finally, use the print_r function to output the $fruits array.
The output result is the same as the above example, and the new element has also been successfully added to the two-dimensional array.
- Use the array_merge function
The array_merge function is a function provided by PHP to merge multiple arrays into one array. You can also use it to merge an array with one or more elements into a new array. The specific method is as follows:
<?php $fruits = array( array("apple", 50), array("banana", 30), array("orange", 20), ); $fruits_new = array(array("grape", 40)); $fruits_merged = array_merge($fruits, $fruits_new); print_r($fruits_merged); ?>
In the above code, we define the new element to be added as a two-dimensional array $fruits_new, and use the array_merge function to merge it into the $fruits array. Finally, use the print_r function to output the $fruits_merged array.
The output result is also the same as the above example, and the new element has also been successfully added to the two-dimensional array.
Summary
The above are three methods of adding new elements to a two-dimensional array, namely using the array_push function, direct assignment and using the array_merge function. In actual development, just choose the appropriate method according to specific needs.
The above is the detailed content of How to add two-dimensional array elements in php. For more information, please follow other related articles on the PHP Chinese website!
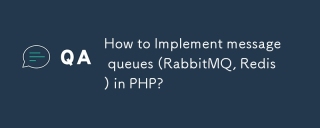
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
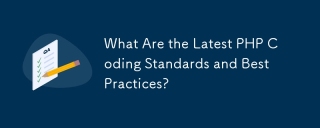
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
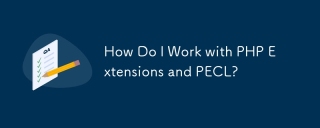
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
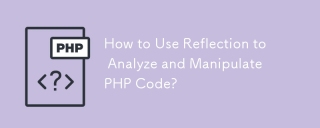
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
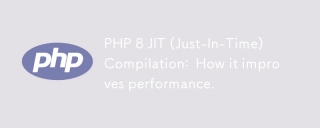
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
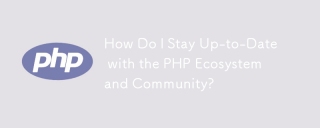
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
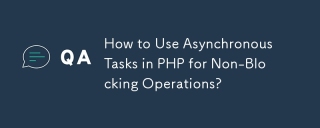
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
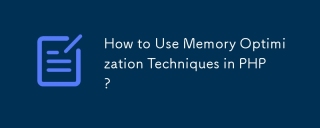
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
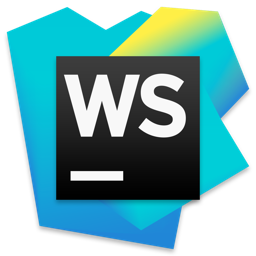
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
