In PHP, replacing the value specified in the array is a frequently used operation. In this article we will explore this topic and provide some examples.
First, let's create a sample array. We will use the following array:
$array = array( 'apple', 'banana', 'pear', 'orange' );
If we want to replace banana
with grape
, we can use the array_search()
function to find banana
position in the array and then use array_splice()
to replace it with grape
.
The sample code is as follows:
$key = array_search('banana', $array); if($key !== false) { array_splice($array, $key, 1, 'grape'); }
This will find the position of banana
in the array and replace it with grape
. Printing the array, we will find that banana
has been replaced by grape
.
The following is the complete sample code:
$array = array( 'apple', 'banana', 'pear', 'orange' ); $key = array_search('banana', $array); if($key !== false) { array_splice($array, $key, 1, 'grape'); } print_r($array);
Output result:
Array ( [0] => apple [1] => grape [2] => pear [3] => orange )
Another way to replace the specified value in an array is to use array_replace()
function. This function can be used to merge two or more arrays and replace elements with the same key in the previous array with elements from the later array. If a key name does not exist in the previous array, it will be added to the new array.
The following is an example of using the array_replace()
function to replace an array value:
$array = array( 'apple', 'banana', 'pear', 'orange' ); $new_array = array_replace($array, array('banana' => 'grape')); print_r($new_array);
The output result is:
Array ( [0] => apple [1] => grape [2] => pear [3] => orange )
In the above code, We passed two arrays to the array_replace()
function. The first array is the original array we want to replace the values with, and the second array is the new array we want to replace the values with. We pass array('banana' => 'grape')
as the second array and replace the values in the original array with the keys banana
.
In this way, we have successfully replaced banana
in the array with grape
.
In general, replacing the value specified in a PHP array is a common operation. Mastering the correct methods and functions can improve our programming efficiency. In this article, we introduced two methods of replacing specified values in an array: using the array_search()
and array_splice()
functions, and using array_replace()
function. Hope these examples are helpful!
The above is the detailed content of php replaces the value specified by the array. For more information, please follow other related articles on the PHP Chinese website!
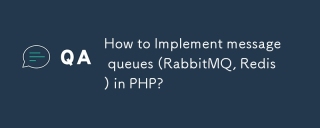
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
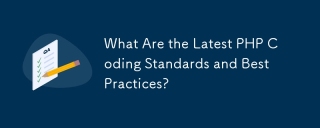
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
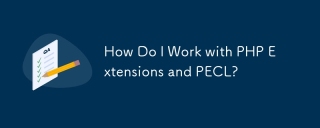
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
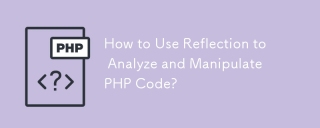
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
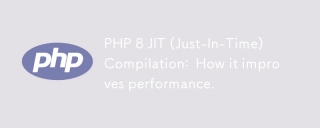
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
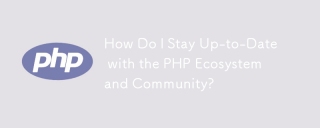
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
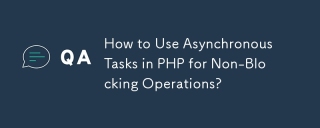
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
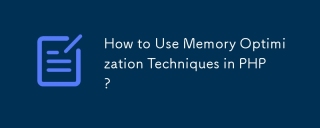
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
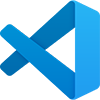
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
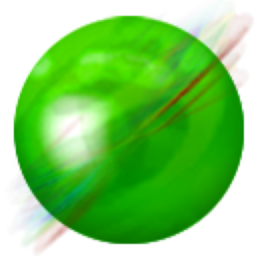
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
