In front-end development, we often need to use arrays to store and process data. Sometimes, we need to delete some elements from the array. In this case, we can use jQuery to easily remove specified elements from the array. This article will introduce how to use jQuery to delete elements in an array and how to implement it.
1. jQuery deletes the specified elements in the array
We can use jQuery’s grep
method to delete the specified elements in the array. This method returns a new array that does not contain the deleted elements.
The following is a sample code:
var array = [1, 2, 3, 4, 5]; var removeElement = 3; array = $.grep(array, function(value) { return value != removeElement; }); console.log(array); //[1, 2, 4, 5]
In the above code, we first define an array array
, and then define an element to be deleted removeElement
. We use the $.grep()
method to remove removeElement
and reassign the returned new array to array
.
In the above example, the $.grep()
method accepts two parameters: the first parameter is the array to be operated on, and the second parameter is a function. This function is used to specify filter conditions. A return value of true means retaining the element, and a return value of false means deleting the element. In this example, we use value != removeElement
to specify the filter condition, which means to retain elements that are not equal to removeElement
.
2. How to delete specified elements in an array
We have already introduced how to use jQuery to delete specified elements in an array. This section describes how to implement this functionality.
- Find out the subscript of the element to be deleted
First, we need to find out the subscript of the element to be deleted in the array. We can use JavaScript's indexOf()
method to find out the index of an element as follows:
var array = [1, 2, 3, 4, 5]; var removeElement = 3; var index = array.indexOf(removeElement); console.log(index); //2
In the above code, we use indexOf()
Method to find the index of element removeElement
. Indexes start counting from 0, so index
has a value of 2.
- Delete elements
After finding the subscript of the element, we can use JavaScript’s splice()
method to delete the element. As shown below:
var array = [1, 2, 3, 4, 5]; var removeElement = 3; var index = array.indexOf(removeElement); if (index > -1) { array.splice(index, 1); } console.log(array); //[1, 2, 4, 5]
In the above code, we first find the index of the element removeElement
and assign it to the variable index
. Then, we determine whether index
is greater than -1 (that is, whether the element exists in the array). If it exists, we use the splice()
method to remove the element. This method accepts two parameters: the index of the element to be deleted and the number of elements to be deleted. In this example, we are removing an element, so the second parameter is 1.
- Encapsulate as a jQuery plug-in
In order to facilitate reuse, we can encapsulate the above code into a jQuery plug-in. As shown below:
$.fn.removeFromArray = function(element) { var index = this.indexOf(element); if (index > -1) { this.splice(index, 1); } return this; };
In the above code, we add this method to jQuery's prototype object through $.fn
so that it can be called in a chain when using this method. This method accepts an element to be deleted as a parameter. We first find the index of the element and then use the splice()
method to delete the element. Finally, we return the modified array object itself.
An example of using this plugin is as follows:
var array = [1, 2, 3, 4, 5]; var removeElement = 3; array.removeFromArray(removeElement); console.log(array); //[1, 2, 4, 5]
In the above code, we use the removeFromArray()
method to remove the element removeElement
.
3. Summary
This article introduces how to use jQuery to delete specified elements in an array, and the implementation principle. Through studying this article, we can handle array operations more flexibly and improve code efficiency and development efficiency.
The above is the detailed content of jquery deletes specified elements in array. For more information, please follow other related articles on the PHP Chinese website!

TonavigateReact'scomplexecosystemeffectively,understandthetoolsandlibraries,recognizetheirstrengthsandweaknesses,andintegratethemtoenhancedevelopment.StartwithcoreReactconceptsanduseState,thengraduallyintroducemorecomplexsolutionslikeReduxorMobXasnee

Reactuseskeystoefficientlyidentifylistitemsbyprovidingastableidentitytoeachelement.1)KeysallowReacttotrackchangesinlistswithoutre-renderingtheentirelist.2)Chooseuniqueandstablekeys,avoidingarrayindices.3)Correctkeyusagesignificantlyimprovesperformanc

KeysinReactarecrucialforoptimizingtherenderingprocessandmanagingdynamiclistseffectively.Tospotandfixkey-relatedissues:1)Adduniquekeystolistitemstoavoidwarningsandperformanceissues,2)Useuniqueidentifiersfromdatainsteadofindicesforstablekeys,3)Ensureke

React's one-way data binding ensures that data flows from the parent component to the child component. 1) The data flows to a single, and the changes in the state of the parent component can be passed to the child component, but the child component cannot directly affect the state of the parent component. 2) This method improves the predictability of data flows and simplifies debugging and testing. 3) By using controlled components and context, user interaction and inter-component communication can be handled while maintaining a one-way data stream.

KeysinReactarecrucialforefficientDOMupdatesandreconciliation.1)Choosestable,unique,andmeaningfulkeys,likeitemIDs.2)Fornestedlists,useuniquekeysateachlevel.3)Avoidusingarrayindicesorgeneratingkeysdynamicallytopreventperformanceissues.

useState()iscrucialforoptimizingReactappperformanceduetoitsimpactonre-rendersandupdates.Tooptimize:1)UseuseCallbacktomemoizefunctionsandpreventunnecessaryre-renders.2)EmployuseMemoforcachingexpensivecomputations.3)Breakstateintosmallervariablesformor

Use Context and useState to share states because they simplify state management in large React applications. 1) Reduce propdrilling, 2) The code is clearer, 3) It is easier to manage global state. However, pay attention to performance overhead and debugging complexity. The rational use of Context and optimization technology can improve the efficiency and maintainability of the application.

Using incorrect keys can cause performance issues and unexpected behavior in React applications. 1) The key is a unique identifier of the list item, helping React update the virtual DOM efficiently. 2) Using the same or non-unique key will cause list items to be reordered and component states to be lost. 3) Using stable and unique identifiers as keys can optimize performance and avoid full re-rendering. 4) Use tools such as ESLint to verify the correctness of the key. Proper use of keys ensures efficient and reliable React applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
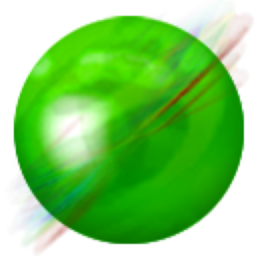
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Chinese version
Chinese version, very easy to use
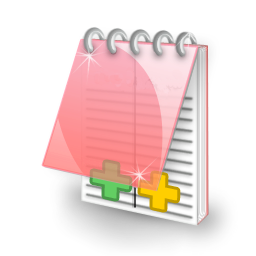
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
