React's One-Way Data Binding: Ensuring Predictable Data Flow
React's one-way data binding ensures that data flows from the parent component to the child component. 1) The data flows to a single, and the changes in the state of the parent component can be passed to the child component, but the child component cannot directly affect the state of the parent component. 2) This approach improves predictability of data flows and simplifies debugging and testing. 3) By using controlled components and context, user interaction and inter-component communication can be handled while maintaining a one-way data stream.
React's one-way data binding is a fundamental concept that ensures predictable data flow within your applications. This approach not only simplifies the management of state but also makes debugging and testing much easier. Let's dive deep into what one-way data binding means in React, why it's beneficial, and how to effectively implement it in your projects.
React's one-way data binding means that data flows in a single direction, from the parent components down to the child components. This ensures that changes in the state of a parent component can be propagated down to its children, but changes in child components cannot directly affect the parent's state. This unidirectional flow of data helps developers maintain a clear understanding of how data moves through their application.
Let's look at a simple example to illustrate this concept:
import React, { useState } from 'react'; function ParentComponent() { const [parentState, setParentState] = useState('Parent State'); Return ( <div> <h1 id="parentState">{parentState}</h1> <ChildComponent parentState={parentState} /> </div> ); } function ChildComponent({ parentState }) { Return ( <div> <p>Child Component: {parentState}</p> </div> ); }
In this example, ParentComponent
manages its own state and passes it down to ChildComponent
. The child can read the state but cannot modify it directly. If you want the child to change the parent's state, you would typically pass a callback function down from the parent to the child.
One of the key advantages of one-way data binding is its prediction. Because data flows in a single direction, it's easier to track down where a state change originated. This makes debugging much simpler because you can follow the flow of data from the point of change to where it's being used.
However, one-way data binding can sometimes feel limiting, especially if you're used to two-way data binding systems. For instance, in forms, you might need to manage state changes from both the parent and child components. React provides solutions like controlled components to handle such scenarios. Here's how you can implement a controlled input:
function ControlledInput() { const [value, setValue] = useState(''); const handleChange = (event) => { setValue(event.target.value); }; Return ( <input type="text" value={value} onChange={handleChange} /> ); }
In this controlled component, the input's value is managed by React state, and any change in the input triggers the handleChange
function to update the state. This maintains the one-way data flow while allowing user interaction to affect the state.
Another aspect to consider is performance. One-way data binding can lead to more efficient updates because React can optimize re-renders based on the flow of data. However, if not managed properly, it can also lead to unnecessary re-renders. To mitigate this, you can use techniques like React.memo
or useCallback
to prevent unnecessary re-renders of child components.
For instance, if you have a child component that doesn't need to re-render when the parent's state changes, you can wrap it with React.memo
:
const ChildComponent = React.memo(function ChildComponent({ parentState }) { Return ( <div> <p>Child Component: {parentState}</p> </div> ); });
This ensures that ChildComponent
only re-renders when its props change, which can be a significant optimization in larger applications.
When working with one-way data binding, it's also cruel to consider the communication between components. Sometimes, you might need to lift the state up to a common ancestor or use context to share state across deeply nested components. Here's an example of using context to share state:
const ThemeContext = React.createContext(); function App() { const [theme, setTheme] = useState('light'); Return ( <ThemeContext.Provider value={{ theme, setTheme }}> <Toolbar /> </ThemeContext.Provider> ); } function Toolbar() { Return ( <div> <ThemedButton /> </div> ); } function ThemedButton() { const { theme, setTheme } = useContext(ThemeContext); Return ( <button style={{ backgroundColor: theme === 'light' ? 'white' : 'black', color: theme === 'light' ? 'black' : 'white' }} onClick={() => setTheme(theme === 'light' ? 'dark' : 'light')} > Toggle Theme </button> ); }
In this example, ThemeContext
allows the ThemedButton
to access and modify the theme state managed by the App
component, maintaining one-way data flow while enabling deep component communication.
In conclusion, React's one-way data binding is a powerful tool that ensures predictable data flow, simplifies state management, and enhances the overall maintainability of your applications. While it might require a shift in thinking, especially for those accustomed to two-way binding, the benefits in terms of prediction and performance optimization are well worth the effort. By understanding and effectively implementing one-way data binding, you can build more robust and scalable React applications.
The above is the detailed content of React's One-Way Data Binding: Ensuring Predictable Data Flow. For more information, please follow other related articles on the PHP Chinese website!

React'sstrongcommunityandecosystemoffernumerousbenefits:1)ImmediateaccesstosolutionsthroughplatformslikeStackOverflowandGitHub;2)Awealthoflibrariesandtools,suchasUIcomponentlibrarieslikeChakraUI,thatenhancedevelopmentefficiency;3)Diversestatemanageme

ReactNativeischosenformobiledevelopmentbecauseitallowsdeveloperstowritecodeonceanddeployitonmultipleplatforms,reducingdevelopmenttimeandcosts.Itoffersnear-nativeperformance,athrivingcommunity,andleveragesexistingwebdevelopmentskills.KeytomasteringRea

Correct update of useState() state in React requires understanding the details of state management. 1) Use functional updates to handle asynchronous updates. 2) Create a new state object or array to avoid directly modifying the state. 3) Use a single state object to manage complex forms. 4) Use anti-shake technology to optimize performance. These methods can help developers avoid common problems and write more robust React applications.

React's componentized architecture makes scalable UI development efficient through modularity, reusability and maintainability. 1) Modularity allows the UI to be broken down into components that can be independently developed and tested; 2) Component reusability saves time and maintains consistency in different projects; 3) Maintainability makes problem positioning and updating easier, but components need to be avoided overcomplexity and deep nesting.

In React, declarative programming simplifies UI logic by describing the desired state of the UI. 1) By defining the UI status, React will automatically handle DOM updates. 2) This method makes the code clearer and easier to maintain. 3) But attention should be paid to state management complexity and optimized re-rendering.

TonavigateReact'scomplexecosystemeffectively,understandthetoolsandlibraries,recognizetheirstrengthsandweaknesses,andintegratethemtoenhancedevelopment.StartwithcoreReactconceptsanduseState,thengraduallyintroducemorecomplexsolutionslikeReduxorMobXasnee

Reactuseskeystoefficientlyidentifylistitemsbyprovidingastableidentitytoeachelement.1)KeysallowReacttotrackchangesinlistswithoutre-renderingtheentirelist.2)Chooseuniqueandstablekeys,avoidingarrayindices.3)Correctkeyusagesignificantlyimprovesperformanc

KeysinReactarecrucialforoptimizingtherenderingprocessandmanagingdynamiclistseffectively.Tospotandfixkey-relatedissues:1)Adduniquekeystolistitemstoavoidwarningsandperformanceissues,2)Useuniqueidentifiersfromdatainsteadofindicesforstablekeys,3)Ensureke


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
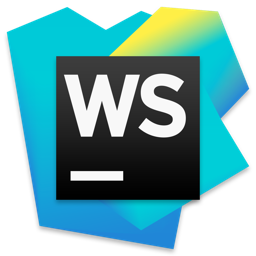
WebStorm Mac version
Useful JavaScript development tools
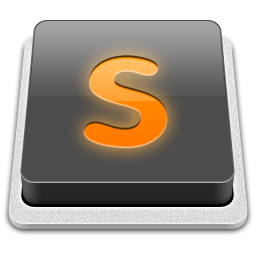
SublimeText3 Mac version
God-level code editing software (SublimeText3)
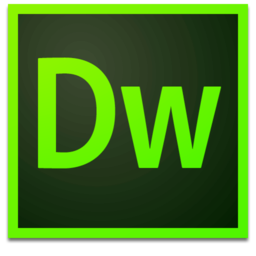
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
