In PHP, Lambda function is also called anonymous function, which refers to a function that does not have an identifier. Lambda functions are also common in other programming languages, such as Python and JavaScript. Compared with regular functions, Lambda functions are more flexible and easier to use. PHP and other programming languages provide Lambda functions to allow programmers to handle complex logical operations more easily.
Lambda function is a new feature introduced in PHP5.3 version. Its syntax is relatively concise and can provide dynamic logical processing for the program without defining function names. The following is a simple usage of the Lambda function:
$func = function($arg1, $arg2) { return $arg1 + $arg2; };
The above code defines a Lambda function and assigns it to the variable $func. The Lambda function starts with the function keyword, followed by the formal parameter list, and then a pair of curly braces, which contains the implementation code of the Lambda function. In this example, the implementation code returns the result of adding the two formal parameters.
It should be noted that the parameter list of a Lambda function can be empty or can contain any number of parameters, but the parameter list needs to be placed in the formal parameter list, not after the function name.
The most common use of Lambda functions is as callback functions. For example, PHP's array_map function can apply a Lambda function to all elements of an array, thereby achieving a one-time processing operation on the array. The following is an example:
// 定义Lambda函数 $multiply = function($n) { return $n * 2; }; // 定义数组 $numbers = [1, 2, 3, 4, 5]; // 使用array_map函数对数组进行处理 $result = array_map($multiply, $numbers); // 输出结果:Array ( [0] => 2 [1] => 4 [2] => 6 [3] => 8 [4] => 10 ) print_r($result);
In the above code, $multiply is a Lambda function that multiplies each element by 2. The array_map function accepts this Lambda function as a processor and processes each element in the $numbers array. Apply this Lambda function to each element, and finally return a new array. This new array contains the result of multiplying all elements by 2.
In addition to serving as callback functions, Lambda functions can also be used in code blocks and closures. When using a Lambda function as a code block, its implementation code runs in the context of the caller. This is because Lambda functions do not have their own scope and they can access variables from external code. Here is an example of using a Lambda function as a code block:
$count = 0; $numbers = [1, 2, 3, 4, 5]; array_walk($numbers, function($value) use(&$count) { $count++; }); echo $count; // 输出值为5
In this example, we define a $count variable with an initial value of 0 and a $numbers array containing 5 elements. We used PHP's array_walk function to iterate over all elements in the array. In this process, we pass the Lambda function to the array_walk function as its second parameter. This Lambda function doesn't do any actual processing, but it uses the $count variable and increments it in the caller's context. Finally, the value of the $count variable we output is 5.
When a Lambda function needs to access external variables and retain their state, it can use closures. A closure is a Lambda function that has access to variables in the context in which it was created. Here is an example of using a Lambda function in the form of a closure:
function counter() { $count = 0; return function() use(&$count) { $count++; return $count; }; } $increment = counter(); echo $increment(); // 输出1 echo $increment(); // 输出2 echo $increment(); // 输出3
In this example, we define a counter function that returns a Lambda function. The returned Lambda function has the ability to access the $count variable originally defined. Each time the returned Lambda function is called, it increments the value of the $count variable and returns it. In this example, we first call the counter function and assign the return value to the $increment variable. We call $increment continuously and output the value after each increment, and eventually 1, 2, and 3 will be output.
In short, the Lambda function is a powerful but easy-to-understand function that can improve code quality, efficiency and flexibility in PHP development. If you haven’t used Lambda functions yet, now is the time to try it!
The above is the detailed content of Lambda function for PHP function. For more information, please follow other related articles on the PHP Chinese website!
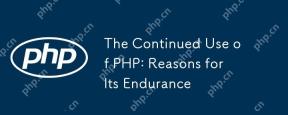
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
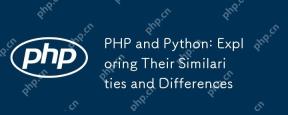
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
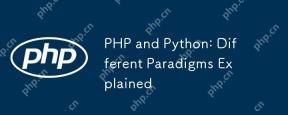
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
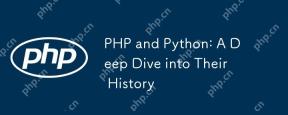
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
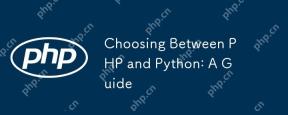
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
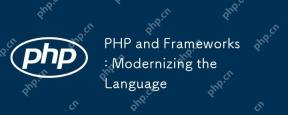
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
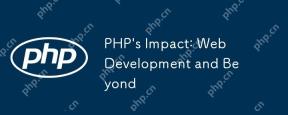
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
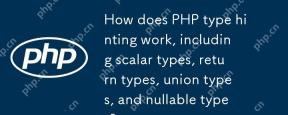
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
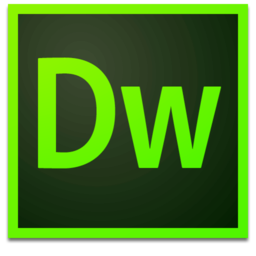
Dreamweaver Mac version
Visual web development tools
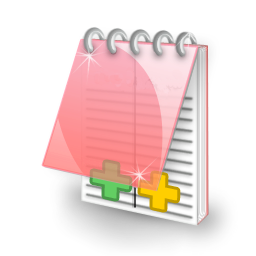
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
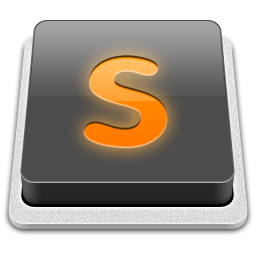
SublimeText3 Mac version
God-level code editing software (SublimeText3)