In the modern Internet era, JavaScript has become one of the cores of front-end development. It can be used to create dynamic and interactive experiences for websites, applications, and other online experiences. For developers who want to improve their JavaScript programming skills, deleting all files in a directory may be one of the operations that all beginners want to try.
The process of deleting all files in a directory is not difficult, but it requires some basic JavaScript knowledge to complete. This article will introduce you to the process of using JavaScript to delete all files in a directory, and provide some notes and suggestions to help you better understand and apply these techniques.
- Find the directory where you want to delete files
To delete files in a directory, you need to first determine which directory you want to operate. In JavaScript, you can use the following code to get the path of a directory:
const directoryPath = "path/to/directory";
Here, "path/to/directory" is the actual path of the directory. You need to replace it with the path to the directory you want to operate on.
- Introduce the Node.js file system module
Before starting the deletion operation, you need to introduce the Node.js file system module. It contains many methods and functions for system file access and manipulation.
const fs = require('fs');
By introducing the file system module, you can easily access the files and folders you need to operate, so you can perform deletion operations.
- Get all files in a directory
To delete all files in a directory, you first need to get all files in the directory. In Node.js you can get all the files in a directory using the following code:
fs.readdir(directoryPath, function(err, files) { if (err) { return console.log('Unable to scan directory: ' + err); } // do something with the files });
Here you read the directory using the "fs.readdir" method and return the names of all the files in the directory or array. If an error occurs, the console will return an error message.
- Delete all files in the directory
Once you have identified all the files in the directory, you can delete them one by one using the following code:
files.forEach(function(file) { fs.unlink(directoryPath + '/' + file, function(err) { if (err) throw err; console.log(file + ' was deleted'); }); });
Here, use the "forEach" function to iterate through all the files in the directory and delete them using the "fs.unlink" method. If an error occurs, an error message will be thrown.
- Complete code example
Finally, the following code is a complete example to delete all files in the directory:
const fs = require('fs'); const directoryPath = "path/to/directory"; fs.readdir(directoryPath, function(err, files) { if (err) { return console.log('Unable to scan directory: ' + err); } files.forEach(function(file) { fs.unlink(directoryPath + '/' + file, function(err) { if (err) throw err; console.log(file + ' was deleted'); }); }); });
Please make sure to change "path/to /directory" with the actual path to the directory you want to operate on.
- Notes and Suggestions
Please note the following notes and suggestions when using JavaScript to delete all files in a directory:
- In Always check if the file exists before deleting it.
- Remember to perform backups or other security measures to prevent unnecessary file loss.
- Before deleting a file, it is best to confirm whether it can be recovered.
- Files in the Recycle Bin and Trash Can may not be permanently deleted, so please proceed with caution.
- If you are not familiar with JavaScript or file system operations, please do not attempt to delete any files or folders.
Summary
In this article, we introduced how to delete all files in a directory using JavaScript. We've also provided some considerations and suggestions to help you perform these operations safely. If you want to learn more about front-end development and JavaScript, please continue to check out related materials and references.
The above is the detailed content of Delete all files in the directory javascript. For more information, please follow other related articles on the PHP Chinese website!
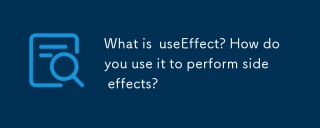
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
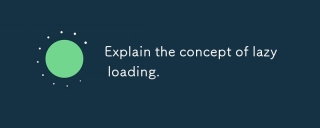
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
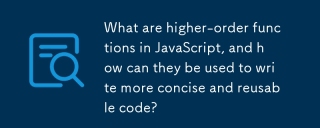
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
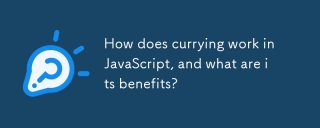
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
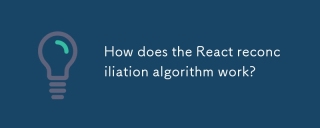
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
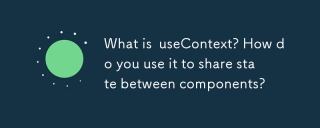
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
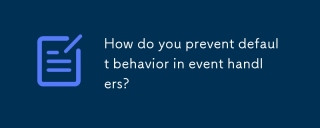
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
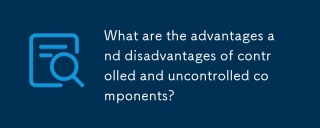
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
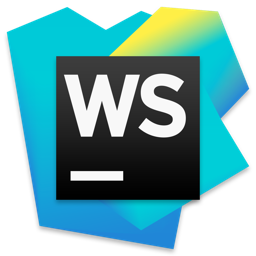
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
