Implementation method of recursive calling of Golang function
Implementation method of recursive calling of Golang functions
With the wide application of Golang in software development, recursive calling of functions has become an important means for programmers to implement complex logic and algorithms. Recursive calling refers to continuously calling itself within a function until a certain condition is met to terminate the loop. In this article, we will explore the implementation of recursive calling of Golang functions.
1. The basic definition of recursive call
Recursive call refers to the process of calling itself within a function. During the execution of the recursive function, the termination condition needs to be determined. If the condition is met, the recursive call will be stopped. Otherwise, continue calling the function itself until the termination condition is met.
In practical applications, recursive calls are used to deal with complex problems that can be split into multiple small problems in the same way, and each small problem can be solved by the same method.
One of the advantages of recursive calling is that it can make the code more concise and easier to understand. At the same time, it also provides a concise way to write some algorithms. One of the disadvantages of recursive calls is that they consume a lot of memory and cause performance problems, so they need to be used with caution in actual applications.
2. Implementation method of recursive calling
The recursive calling of Golang functions is similar to the recursive calling methods of other programming languages. We use a case to explain how to implement recursive calls in Golang.
Case: Calculate the factorial of an integer
In mathematics, factorial refers to the result of multiplying all positive integers from 1 to n, usually represented by the symbol n!. For example, 4!=4×3×2×1=24. Let's take calculating the factorial of an integer as an example to illustrate the implementation method of recursive calls.
In Golang, we can implement a function that calculates factorial through the following code:
func factorial(n int) int { if n == 0 || n == 1 { return 1 } else { return n * factorial(n-1) } }
The above code is a recursive function, and calls itself in the function to implement recursive calls. The first parameter n of the function is the integer whose factorial needs to be calculated. At the beginning of the function, we use an if statement to determine whether the value of n is 0 or 1. If n is 0 or 1, it returns 1 directly; otherwise, it calls itself recursively and returns n multiplied by the call result.
During a recursive call, each call will reduce the value of n by 1 until n equals 0 or 1. The call is terminated, that is, the condition of the above if statement is met. For example, when calculating the factorial of 4, the recursive call process is as follows:
factorial(4) = 4 * factorial(3)
factorial(3) = 3 * factorial(2)
factorial(2) = 2 * factorial(1)
factorial(1) = 1
Expand the above calling process and get the following table:
n | factorial(n) | n - 1 |
---|---|---|
4 | 3 | |
12 | 2 | |
24 | 1 | |
1 | 0 |
3. Precautions for recursive calls
When using recursive calls, you need to pay attention to the following important matters.
Determine the termination condition- In recursive calls, the termination condition must be clearly determined, otherwise it will lead to an infinite loop and waste system resources. In the factorial case above, the termination condition is that n equals 0 or 1.
- Recursive calls must have a clear calling condition. In the factorial case above, the calling condition is n equals n-1.
- When using recursive calls, you must pay attention to the order of function calls. If the order of calls is incorrect, recursive calls will not be executed normally.
- Recursive calls are very convenient when implementing certain algorithms, but they can also become one of the main reasons for low code performance. Therefore, in practical applications, recursive calls should be chosen carefully.
Conclusion
Through this article, we have learned about the implementation methods and precautions for recursive calling of Golang functions. Recursive calls are also widely used in other programming languages. In the actual coding process, we should seek a balance between maintaining code logic and performance to ensure code readability and execution efficiency.
The above is the detailed content of Implementation method of recursive calling of Golang function. For more information, please follow other related articles on the PHP Chinese website!
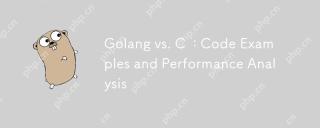
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
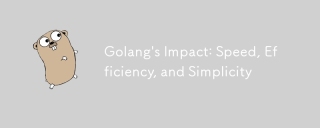
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
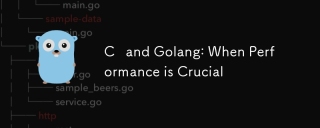
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
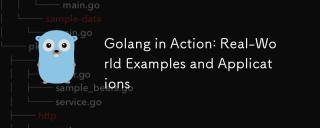
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
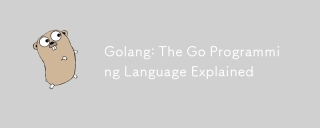
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
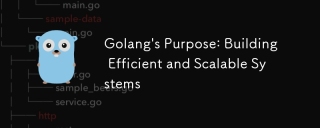
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
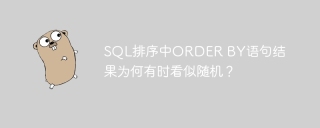
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
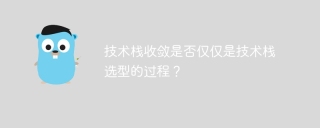
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
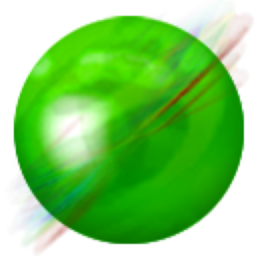
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
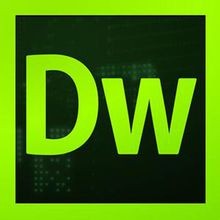
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version