


With the continuous development of computer technology, various languages have also emerged. Among them, Golang (also known as GO language) has become more and more popular among developers in recent years because of its efficiency, simplicity, and ease of learning. In Golang, function system calls and file system operations are common application techniques. This article will introduce the application methods of these techniques in detail to help everyone better master Golang development skills.
1. Function system call
1. What is the system call?
System call is a service interface provided by the operating system kernel and an interactive interface between user space and kernel space. By calling system calls, user space programs can send requests to the kernel space, obtain access and usage rights to system resources, and process underlying hardware resources.
2.What are the system call functions in Golang?
The system call functions of different operating systems in Golang are different. The following are several common system call functions:
- Unix/Linux system: syscall.Syscall in the syscall package Function
- Windows system: syscall.Syscall and syscall.Syscall6 functions in the syscall package
Common system calls include file reading and writing, network communication, sub-process operation, and system parameter acquisition wait.
3. How to use system call function?
The following is the basic method of using system call functions in Golang:
- Unix/Linux system:
Use syscall.Syscall to call system calls , and return the call result. For example, the following code demonstrates how to use system calls to read and write files:
data := []byte("hello, world!") file, err := syscall.Open("./test.txt", syscall.O_CREAT|syscall.O_TRUNC|syscall.O_RDWR, 0666) if err != nil { panic(err) } defer syscall.Close(file) _, _, err = syscall.Syscall(syscall.SYS_WRITE, uintptr(file), uintptr(unsafe.Pointer(&data[0])), uintptr(len(data))) if err != 0 { panic(err) }
Among them, the function syscall.Open is used to open files, and syscall.Syscall is used to make system calls. Finally use syscall.Close to close the file.
- Windows system:
Use syscall.Syscall and syscall.Syscall6 to call system calls. For example, the following code demonstrates how to use system calls to open a file:
h, _, _ := syscall.Syscall( procCreateFileW.Addr(), 6, uintptr(unsafe.Pointer(syscall.StringToUTF16Ptr(path))), uintptr(desiredAccess), uintptr(shareMode), uintptr(unsafe.Pointer(lpSecurityAttributes)), uintptr(creationDisposition), uintptr(flagsAndAttributes), ) if h == uintptr(syscall.InvalidHandle) { return nil, os.NewSyscallError(fmt.Sprintf("invalid handle: %X", h), err) } defer syscall.CloseHandle(syscall.Handle(h))
Among them, syscall.Syscall6 is used to open the file, and syscall.CloseHandle is used to close the file handle.
2. File system operations
1. What are file system operations?
File system operations are technologies that perform operations such as reading, writing, creating, and deleting files and directories. In Golang, the file system of the operating system can be easily implemented using the file system operation functions provided by the os package.
2.What are the file system operation functions in Golang?
The following are common file system operation functions in Golang:
- os.Open: open a file
- os.Create: create a file
- os.Remove: Delete the file
- os.Rename: Rename the file
- os.Mkdir: Create the directory
- os.Chdir: Change the current working directory
- os.Stat: Get file information
- os.ReadFile: Read file content
- os.WriteFile: Write file content
3. How to use File system operation function?
The following is the basic method of using file system operation functions in Golang:
Read file content:
data, err := ioutil.ReadFile("./test.txt") if err != nil { panic(err) } fmt.Print(string(data))
Write file content:
data := []byte("hello, world!") err := ioutil.WriteFile("./test.txt", data, 0666) if err != nil { panic(err) }
Create Directory:
err := os.Mkdir("./test", 0755) if err != nil { panic(err) }
Delete file:
err := os.Remove("./test.txt") if err != nil { panic(err) }
Rename file:
err := os.Rename("./test.txt", "./test_new.txt") if err != nil { panic(err) }
Get file information:
info, err := os.Stat("./test_new.txt") if err != nil { panic(err) } fmt.Println(info.Name(), info.Size())
3. Summary
In this article, we introduce the application skills of function system calls and file system operations in Golang, including the basic understanding of system calls, different system call functions of the operating system, and the use of system call functions to implement file read and write operations. At the same time, we also introduced the basic understanding of file system operations, common file system operation functions, and methods of using these functions to perform file reading, writing, creation, deletion and other operations. I hope this article can help everyone better master Golang development skills.
The above is the detailed content of Application skills of system calls and file system operations of Golang functions. For more information, please follow other related articles on the PHP Chinese website!
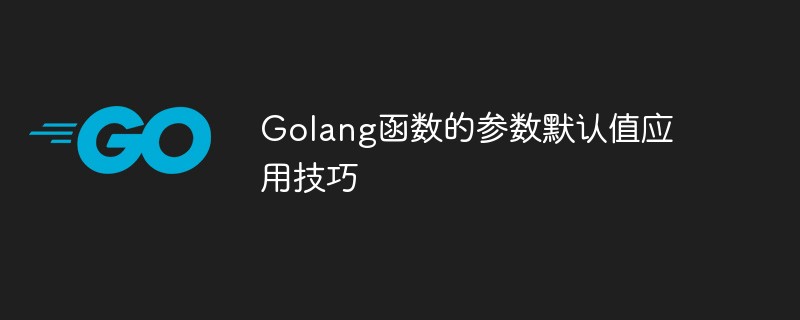
Golang是一门现代化的编程语言,拥有很多独特且强大的功能。其中之一就是函数参数的默认值应用技巧。本文将深入探讨如何使用这一技巧,以及如何优化代码。一、什么是函数参数默认值?函数参数默认值是指定义函数时为其参数设置默认值,这样在函数调用时,如果没有给参数传递值,则会使用默认值作为参数值。下面是一个简单的例子:funcmyFunction(namestr
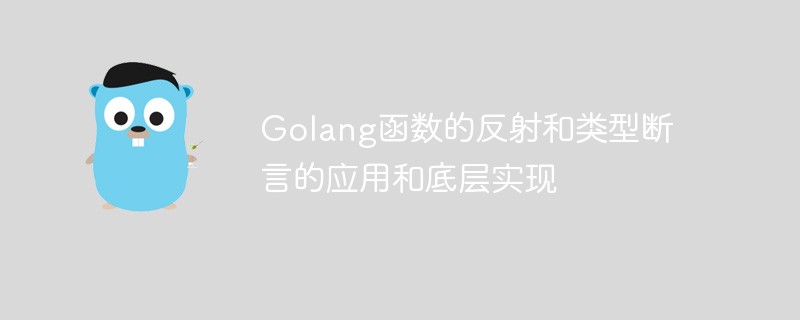
Golang函数的反射和类型断言的应用和底层实现在Golang编程中,函数的反射和类型断言是两个非常重要的概念。函数的反射可以让我们在运行时动态的调用函数,而类型断言则可以帮助我们在处理接口类型时进行类型转换操作。本文将深入讨论这两个概念的应用以及他们的底层实现原理。一、函数的反射函数的反射是指在程序运行时获取函数的具体信息,比如函数名、参数个数、参数类型等
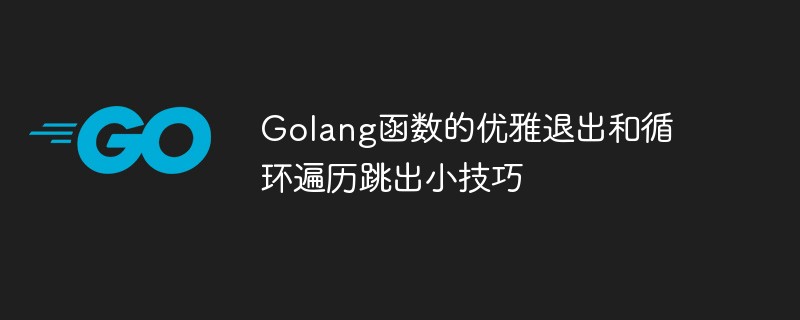
Golang作为一门开发效率高、性能优异的编程语言,其强大的函数功能是其关键特性之一。在开发过程中,经常会遇到需要退出函数或循环遍历的情况。本文将介绍Golang函数的优雅退出和循环遍历跳出小技巧。一、函数的优雅退出在Golang编程中,有时候我们需要在函数中优雅地退出。这种情况通常是因为我们在函数中遇到了一些错误或者函数的执行结果与预期不符的情况。有以下两
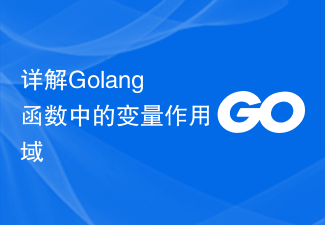
Golang函数中的变量作用域详解在Golang中,变量的作用域指的是变量的可访问范围。了解变量的作用域对于代码的可读性和维护性非常重要。在本文中,我们将深入探讨Golang函数中的变量作用域,并提供具体的代码示例。在Golang中,变量的作用域可以分为全局作用域和局部作用域。全局作用域指的是在所有函数外部声明的变量,即在函数之外定义的变量。这些变量可以在整
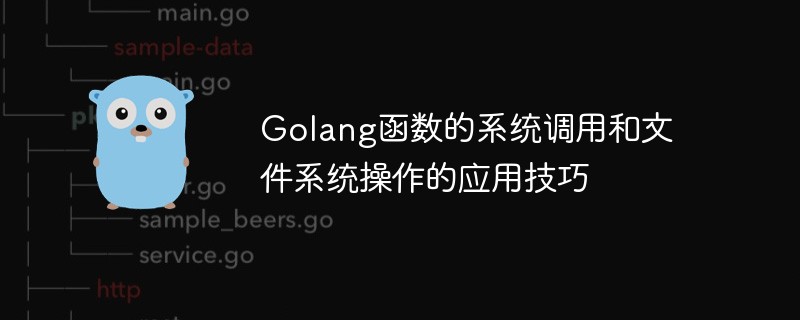
随着计算机技术的不断发展,各种语言也应运而生。其中,Golang(又称GO语言)因为其高效、简单、易于学习的特点,在近年来越来越受到开发者们的青睐。在Golang中,函数的系统调用和文件系统操作是常见的应用技巧。本文将详细介绍这些技巧的应用方法,以帮助大家更好地掌握Golang的开发技能。一、函数的系统调用1.系统调用是什么?系统调用是操作系统内核提供的服务
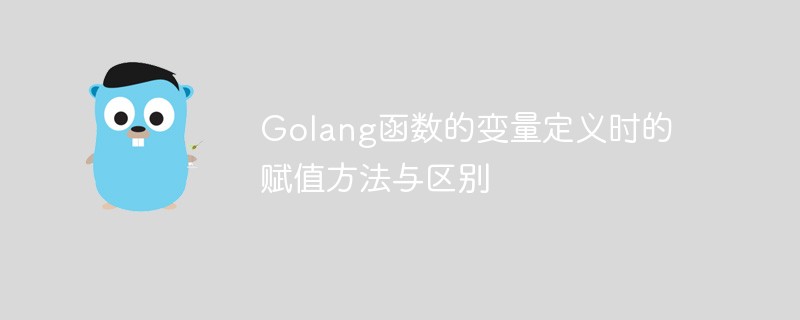
Golang是一种快速、高效、现代化的编程语言,它在编译时会自动检查类型,并且具有并发性和内存安全性等特点,因此被越来越多的开发者所青睐。在Golang中,我们经常需要使用函数来封装业务逻辑,而函数中的变量定义时的赋值方法是一个常见的问题,本文将详细讲解这个问题并分析其中的区别。变量定义在Golang中,可以使用var和:=两种方式来定义变量。其中,var方
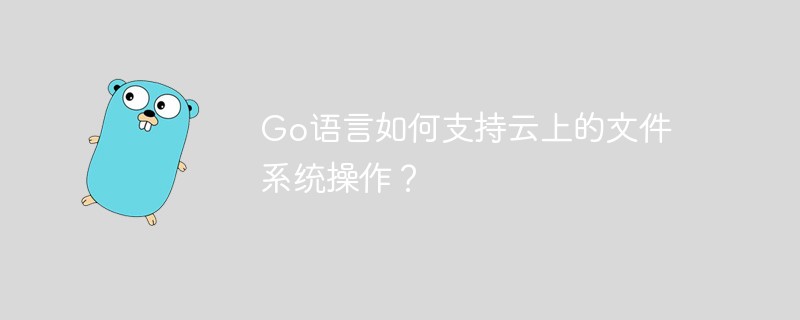
随着云计算技术的不断发展,越来越多的应用程序被迁移到了云上。由于云上的文件系统不同于本地文件系统,开发者需要使用不同的语言和工具来操作这些文件系统。Go语言是一种快速、高效、并发的编程语言,越来越受到开发者的青睐。本文将介绍如何使用Go语言来支持云上的文件系统操作。Go语言对云上文件系统的支持Go语言通过标准库提供了一组能够操作本地文件系统的函数,例如:os
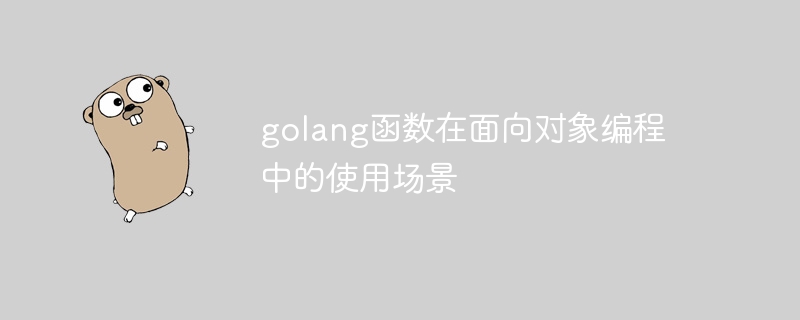
在面向对象编程(OOP)中,GoLang函数被用来封装数据和操作,实现代码的可重用性和模块化。具体用途包括:封装数据和逻辑,隐藏实现细节。实现多态性,以不同的方式处理不同类型的数据。促进代码重用,避免重复编写代码。事件处理,创建并行执行的函数来处理事件。实战案例中,GoLang函数用于实现用户账户管理系统的功能,如验证凭据、创建账户和处理用户请求。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
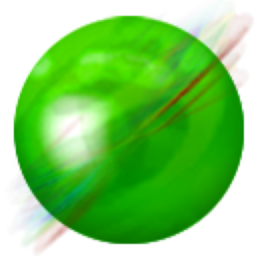
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
